What is the Difference Between BehaviorSubject and Observable in Angular ?
Last Updated :
05 Dec, 2023
Angular being a powerful framework offers various features and tools. One such powerful feature is to handle asynchronous operations by implementing the BehaviorSubject and Observable, which are the key components of the RxJS library. It also helps to manage the flow of data reactively. This article covers the basics of BehaviorSubject and Observable in Angular, along with understanding their basic implementation, & the differences between them.
BehaviorSubject
BehaviorSubject is a concept in the RxJS library that is an extension of observable. This not only provides a stream of data but maintains the most recent value or latest value to subscribers. It acts as both observer and observable. Here when a late subscriber arrives, they immediately receive the latest value present. This is an advantage to BehaviorSubject when the current state is crucial, and subscribers need to access values immediately to the latest emitted value.
Syntax
import { BehaviorSubject } from 'rxjs';
// Create a BehaviorSubject with an initial value
const myBehaviorSubject = new BehaviorSubject<string>('Initial Value');
// Subscribe to the BehaviorSubject
myBehaviorSubject.subscribe(value => {
// Handle the received value
});
// Update the value of the BehaviorSubject
myBehaviorSubject.next('New Value');
Example: This example involves a button when clicked updates the value and displays on the screen. We also have another button to subscribe to BehaviorSubject. Through this, we demonstrate how values are updated after subscribing to BehaviorSubject.
HTML
< div >
< p >Current Value: {{ currentValue }}</ p >
< button (click)="updateValue()">
Update Value
</ button >
< button (click)="delayedSubscribeToBehaviorSubject()">
Subscribe to BehaviorSubject
</ button >
< div * ngIf = "behaviorSubjectSubscriptionValue" >
< p >
Subscribed to BehaviorSubject:
{{ behaviorSubjectSubscriptionValue }}
</ p >
</ div >
</ div >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { BehaviorSubject, Observable } from 'rxjs' ;
@Component({
selector: 'app-difference-example' ,
templateUrl: './difference-example.component.html' ,
})
export class DifferenceExampleComponent implements OnInit {
private myBehaviorSubject =
new BehaviorSubject<string>( 'Initial Value' );
currentValue!: string;
behaviorSubjectSubscriptionValue!: string;
ngOnInit() {
this .myBehaviorSubject.subscribe(value => {
this .currentValue = value;
});
}
updateValue() {
const newValue =
`New Value ${Math.floor(Math.random() * 100)}`;
this .myBehaviorSubject.next(newValue);
}
delayedSubscribeToBehaviorSubject() {
setTimeout(() => {
this .myBehaviorSubject.subscribe(value => {
this .behaviorSubjectSubscriptionValue = value;
});
}, 1000);
}
}
|
Output: As you can observe through the above example, the updated value just updates the value then after subscribing to BehaviorSubject, the values are initialized from the latest value and are updated the same.
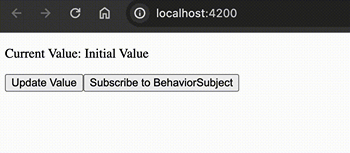
Observable
An observable represents a stream of data that can be observed over a period of time. It helps in asynchronous operations well which helps components to react to the changes by subscribing to it. Late subscribers receive values only after subscription, unlike BehaviorSubject which gets the latest value. These are like immutable which can’t access the previous values before subscription.
Syntax
import { Observable } from 'rxjs';
// Create a regular Observable with no initial value
const myObservable = new Observable<string>(observer => {
observer.next('Initial Value'); // No initial value, just an example
});
// Subscribe to the Observable
myObservable.subscribe(value => {
// Handle the received value
});
// Function to emit a new value in the Observable syntax
const emitValue = () => {
// Trigger the emission of a new value
};
Example: The below program describes the usage of BehaviorSubject and Observable with the help of the same goal. So, we can figure out the difference between them and their work.Â
The example is the same as for BehaviorSubject, which involves a button that on clicked updates the value and displays it on the screen. We also have another button to subscribe to Observable. Through this, we demonstrate how values are updated after subscribing to observable.
HTML
< div >
< p >
Current Value: {{ currentValue }}
</ p >
< button (click)="updateValue()">
Update Value
</ button >
< button (click)="delayedSubscribeToObservable()">
Subscribe to Observable
</ button >
< div * ngIf = "observableSubscriptionValue" >
< p >
Subscribed to Observable:
{{ observableSubscriptionValue }}
</ p >
</ div >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { Observable } from 'rxjs' ;
@Component({
selector: 'app-difference-example' ,
templateUrl: './difference-example.component.html' ,
})
export class DifferenceExampleComponent implements OnInit {
private myObservable =
new Observable<string>(observer => {
observer.next( 'Initial Value' );
this .emitValue = () => observer.next(
`New Value ${Math.floor(Math.random() * 100)}`);
});
currentValue!: string;
observableSubscriptionValue!: string;
emitValue!: () => void;
ngOnInit() {
this .myObservable.subscribe(value => {
this .currentValue = value;
});
}
updateValue() {
this .emitValue();
}
delayedSubscribeToObservable() {
setTimeout(() => {
this .myObservable.subscribe(value => {
this .observableSubscriptionValue = value;
});
}, 1000);
}
}
|
Output: As you can observe through the above example, the updated value just updates the value then after subscribing to Observable the values are not initialized and started from a new initial state unlike BehaviorSubject which is initialized with the latest value.
.gif)
Difference between BehaviorSubject and Observable
Category
|
BehaviorSubject
|
Observable
|
Initialization
|
Has initial value emitted immediately after subscription. |
No initial value |
Immediate Emit
|
Emits the latest value and all subsequent new values to new subscribers. |
Emits values over time which are after subscription. |
Last value memory
|
Retains the latest value. |
Does not retain previous ones. |
Use case
|
Useful when we need subscribers to get the current value. |
Useful when past values are not very important. |
Example
|
Maintaining the current user state in an application. |
Periodic data updates. |
Flexibility
|
More complex, used when components need immediate access to the current state or values. |
Simple, used when there is a need for one-way data flow. |
Share your thoughts in the comments
Please Login to comment...