What is appendChild in JavaScript ?
Last Updated :
14 Feb, 2024
In JavaScript, the appendChild() method is used to append a new child node to the end of the list of children of the specified parent node. This method is commonly used in DOM manipulation to add new elements to an existing HTML structure dynamically.
Syntax:
selectedElement.appendChild(newChild);
Parameters:
- newChild: The new child node that will be appended to the selected element.
Return Value:
The appendChild() method does not return anything instead it appends the new child element to the selected element.
Example 1: The below code is a basic implementation of the appendChild() method to append a child element.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >AppendChild Example</ title >
< style >
#container{
text-align: center;
}
</ style >
</ head >
< body >
< div id = "container" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< p >
This is the existing content of
the container.
</ p >
</ div >
< script >
const paragraph1 =
document.createElement('p');
paragraph1.textContent =
`This is a new paragraph element.
Added using appendChild() method.`;
document.getElementById('container').
appendChild(paragraph1);
</ script >
</ body >
</ html >
|
Output :
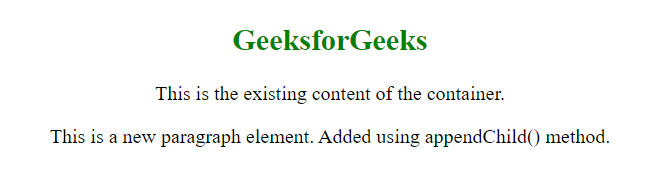
Example 2: The below code will explain the use of the appendChild() method to add content on click to the button.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >AppendChild Example</ title >
< style >
#container {
text-align: center;
}
button {
background-color: green;
color: #fff;
border: none;
border-radius: 10px;
padding: 15px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div id = "container" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< p >
This is the existing content of
the container.
</ p >
< button id = "btn" >
Click to add content
</ button >
</ div >
< script >
const btn = document.getElementById('btn');
btn.addEventListener('click', () => {
const paragraph1 =
document.createElement('p');
paragraph1.textContent =
`This is a new paragraph element.
Added using appendChild() method.`;
document.getElementById('container').
appendChild(paragraph1);
});
</ script >
</ body >
</ html >
|
Output :
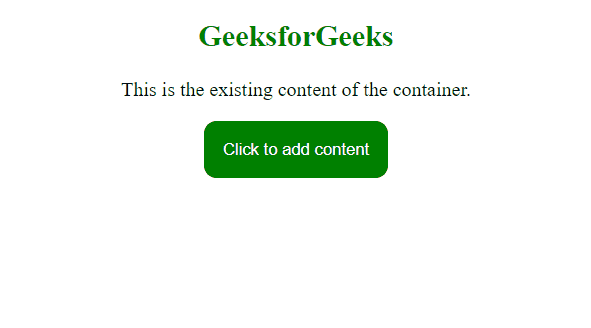
Share your thoughts in the comments
Please Login to comment...