Web Storage API
Last Updated :
11 Mar, 2024
Web API Storage facilitates the feature of storing data within the browser on the client side. It is also referred to as web storage. It uses a key-value pair format to store the data.
Web Storage API Concepts and Usage
The Web Storage API provides two mechanisms for storing data:
- sessionStorage:
- Maintains a separate storage area for each origin during the page session (as long as the browser remains open, including page reloads and restores).
- Stores data only for the current session, meaning it persists until the browser or tab is closed.
- Data is never transferred to the server.
- The storage limit is larger than that of cookies (up to 5MB).
- localStorage:
- Persists even when the browser is closed and reopened.
- Stores data with no expiration date and gets cleared only through JavaScript or by clearing the browser cache or locally stored data.
- The storage limit is greater than that of sessionStorage.
Both mechanisms are accessible via the Window.sessionStorage
 and Window.localStorage
 properties. Invoking one of these properties creates an instance of the Storage
 object, which allows you to set, retrieve, and remove data items.
Web Storage API Interfaces
- Storage: It provides access to a particular local storage.
- Window: It is a window containing a DOM document.
- StorageEvent: It is implemented by a storage event which is sent to a window when a storage area changes.
Examples Showing of Web Storage API
1. Using sessionStprage setItem Method:
Example: In this example, we will store the data using the sessionStorage setItem method and retrieve them using the sessionStorage getItem method.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Session storage</ title >
</ head >
< body >
< center >
< h1 style = "color:green" >Welcome To GFG</ h1 >
< h2 >Geeks details From session Storage</ h2 >
< h2 id = "ele" ></ h2 >
</ center >
< script >
sessionStorage.setItem('name', 'Arvind Kumar')
sessionStorage.setItem('Course', 'Mtech');
sessionStorage.setItem('Age', 23);
sessionStorage.setItem('Batch', '3rd');
document.getElementById("ele").innerHTML = '< h3 >Name: '
+ sessionStorage.getItem("name") + '</ h3 >< h3 >Course: ' +
sessionStorage.getItem('Course') + '</ h3 >' + '< h3 > Batch: ' +
sessionStorage.getItem('Batch') + '</ h3 >' + '< h3 >Age: ' +
sessionStorage.getItem('Age') + '</ h3 >';
</ script >
</ body >
</html
|
Output:
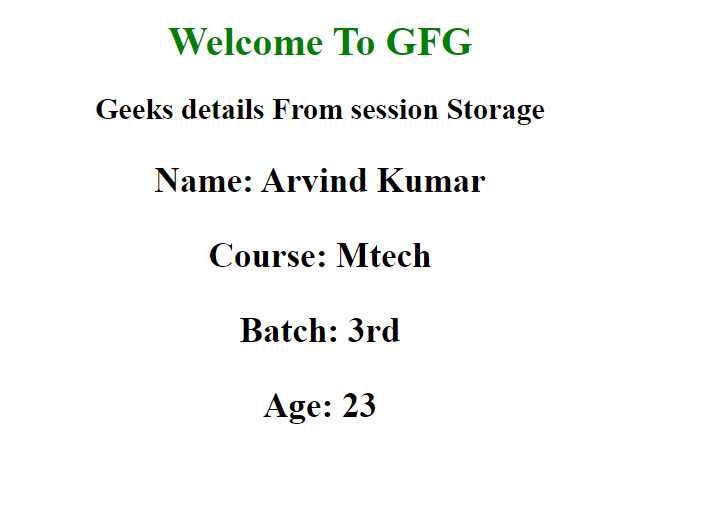
session1
Output of session Storage file:
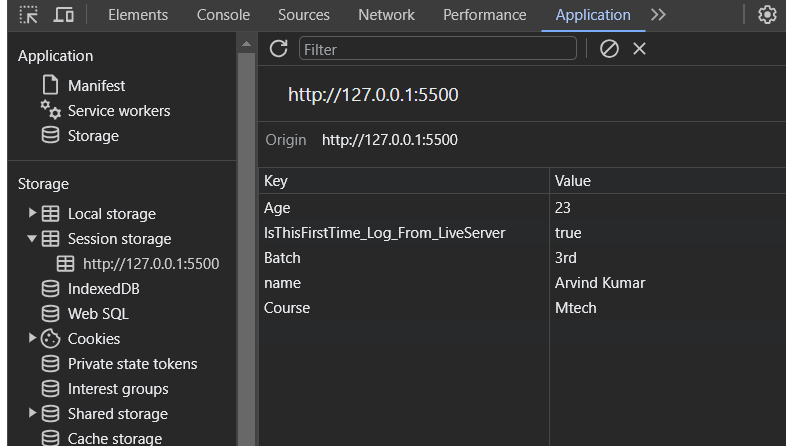
sessionStorage
2. Using localStorage setItem Method:
Example: In this example we will store the data using localStorage setItem and retrieve them using getItem method.
HTML
<!DOCTYPE html>
< html >
< head >
< title >local storage</ title >
</ head >
< body >
< center >
< h1 style = "color:green" >Welcome To GFG</ h1 >
< p >
< label >Username : < input id = "name" /></ label >
</ p >
< p >
< label >Password : < input id = "password" /></ label >
</ p >
< p >
< button onclick = "fun()" >Submit</ button >
</ p >
< p >
< button onclick = "fun2()" >Get details</ button >
</ p >
< h2 id = "ele" ></ h2 >
</ center >
< script >
function fun() {
localStorage.setItem('name',
document.getElementById('name').value)
localStorage.setItem('password',
document.getElementById('password').value);
}
function fun2() {
document.getElementById("ele").innerHTML = '< h3 >name: '
+ localStorage.getItem("name") + '</ h3 >< h3 >Passward: ' +
localStorage.getItem('password') + '</ h3 >';
}
</ script >
</ body >
</ html >
|
Output:
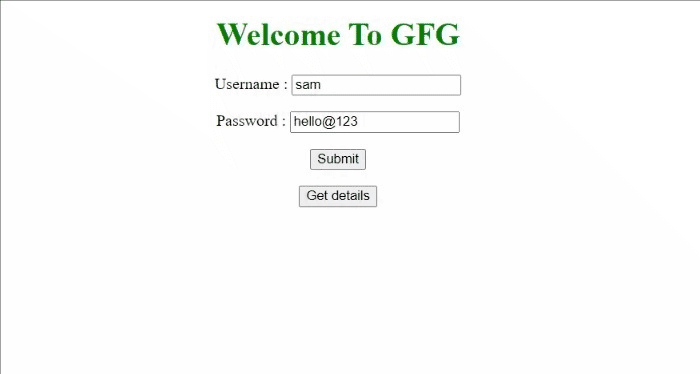
local2
Output of local storage:
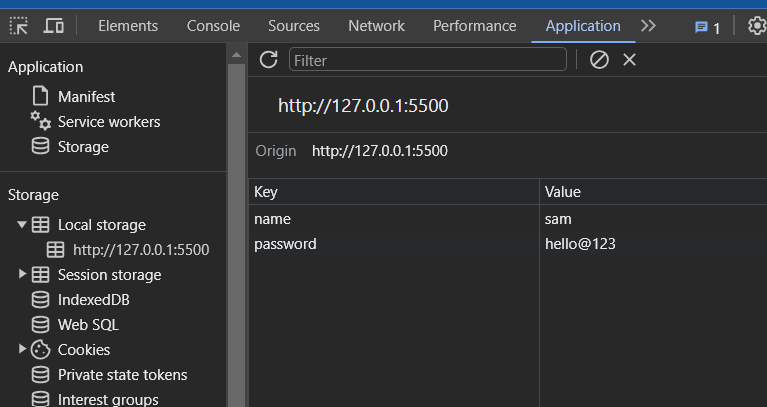
In summary, the Web Storage API simplifies data storage and retrieval, making it a valuable tool for web developers. Remember to use it wisely and consider the storage limits and security implications.
Supported Browsers:
- Chrome
- Edge
- Firefox
- Safari
- Opera
Share your thoughts in the comments
Please Login to comment...