View Existing Validation Rules in MongoDB
Last Updated :
28 Dec, 2023
MongoDB is a NoSQL database. MongoDB database can handle large, complex, and unstructured data with easy scalability. It allows us to make changes to the Schema as and when required. The Schema validation rules are a set of rules that are applied to the document when it is added to the collection. If the document adheres to the rules, then it is added, else the MongoDB throws a validation error that prohibits adding the document to the collection. MongoDB also allows us to view the existing schema validation rules that are applied to any collection.
We will be using Mongoose in the below example to understand and view the JSON Schema validation in a MongoDB collection. mongosh is a CLI provided by the MongoDB community itself to interact with its utilities and collections, i.e., without any GUI.
JSON Schema Validation Rules
JSON Schema validation rules are a bunch of rules in a JSON format, that define which document can be added into a collection. The Schema can have rules, like the `age` property should have a minimum value of 18, and so the document, that we are trying to add, will fail to get inserted into the collection if it does not follow the said criteria in the Schema validation.
Viewing Existing Schema Validation Rules
We can view the existing JSON Schema Validation in the collections present in MongoDB. Let’s see how we can do this in the steps below –
Step 1: Create a MongoDB Collection and Add the Schema Validation Rules
We will create a users collection and add a JSON Schema Validation rule to the collection. We will be using the below JSON schema as a validator to add to the collection. This JSON Schema contains properties that specify which properties will the documents in the collection have, and the validators on the properties are used for data validation before entering any document in the collection. If the data doesn’t pass the validation, MongoDB throws an exception error.
JSON Schema for Validation:
We will create a users collection and add a JSON Schema Validation rule to the collection.
db.createCollection('users', {
validator: {
"$jsonSchema": {
"bsonType": "object",
"properties": {
"first_name": {
"bsonType": "string",
"description": "must be a string and is required"
},
"last_name": {
"bsonType": "string",
"description": "must be a string and is required"
},
"email": {
"bsonType": "string",
"pattern": "^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,4}$",
"description": "must be a valid email address"
}
}
}
}
})
Output:
{ ok: 1 }
Step 2: Using the db.getCollectionInfos() Method to View the Validation Rules
We will use the db.getCollectionInfos() method to return the validation rules for the users collection. The db.getCollectionInfos() method provides the meta information related to the collection, like what are the properties, their validations, their types, etc.
Output:
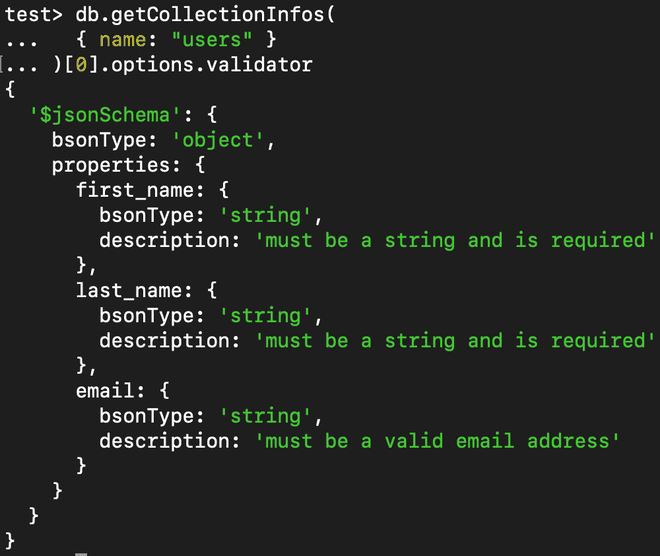
Step 3: Using the listCollections Syntax to Return the Validation Rules for the Collection
We will use the listCollections syntax here to view the validation rules. The listCollections syntax provides us a cursor that contains information about the collection, like the properties and their types, their validations, etc. Both, the listCollections and getCollectionsInfo return the same information, but the output format differs slightly for each command.
Output:
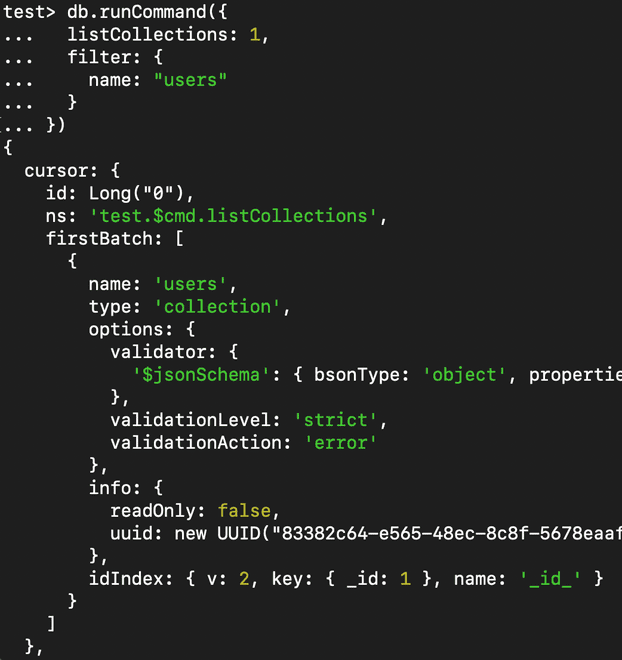
Conclusion
In conclusion, we learned about the JSON Schema validation in MongoDB, and how we can view the applied Schema validations on a given MongoDB collection. There are currently 2 different ways to view the schema validations that we learnt, one is using the db.getCollectionInfos() method, which returns the validation rules for the Users collection, and the other is listCollections that provides a cursor that contains information about the collection.
Share your thoughts in the comments
Please Login to comment...