Typing Game using React
Last Updated :
08 May, 2024
React Typing Game is a fun and interactive web application built using React. It tests and improves the user’s typing speed and accuracy by presenting sentences for the user to type within a specified time limit. The game provides real-time feedback by highlighting any mistakes made by the user, making it both educational and entertaining.
A preview image of the final output:
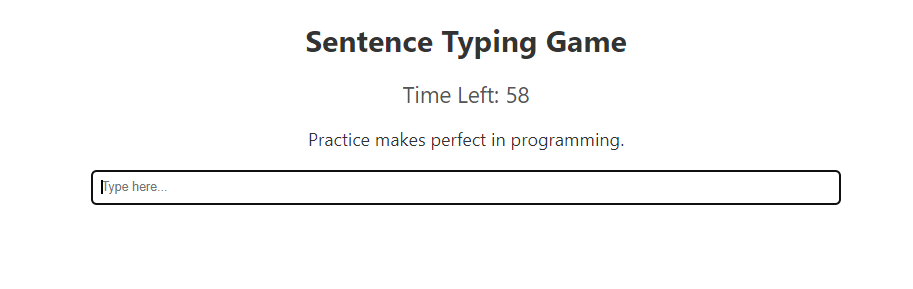
Prerequisite:
Approach to Create Typing Game using React
- Display a sentence for the user to type.
- Start a timer for the typing session.
- Highlight mistakes in real-time by changing the background color of the incorrect word.
- Calculate and display the user’s score based on correct typings.
- Provide a start button to initiate the typing game.
- Allow users to select difficulty levels for sentences.
- Display a game over message with the final score when the time runs out.
Steps to Create the React App:
Step 1: Set up a new React project using:
npx create-react-app typing-game
Step 2: Navigate to the root of the project using the following command:
cd typing-game
Project structure:
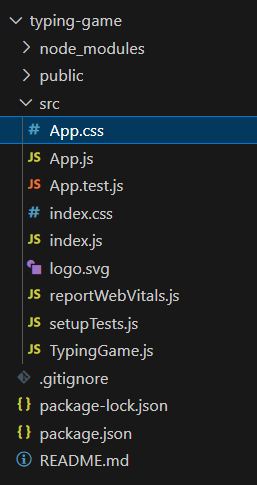
The Updated package.json will look like:
"dependencies": {
"react": "^18.3.0",
"react-dom": "^18.3.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: Below is an example of creating a write typing game using React.
CSS
/* App.css */
.container {
max-width: 800px;
margin: 0 auto;
text-align: center;
padding: 20px;
}
.title {
font-size: 2rem;
margin-bottom: 20px;
color: #333;
}
.start-button {
background-color: #4CAF50;
color: white;
border: none;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin-bottom: 20px;
cursor: pointer;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.start-button:hover {
background-color: #45a049;
}
.timer {
font-size: 1.5rem;
margin-bottom: 20px;
color: #555;
}
.sentence {
font-size: 1.2rem;
margin-bottom: 20px;
color: #333;
}
.input-container {
margin-bottom: 20px;
}
.input-field {
padding: 10px;
width: 100%;
box-sizing: border-box;
border-radius: 5px;
border: 1px solid #ccc;
transition: border-color 0.3s ease;
}
.input-field:focus {
border-color: #4CAF50;
}
.game-over {
font-size: 1.5rem;
color: #f44336;
margin-bottom: 20px;
transition: color 0.3s ease;
}
.game-over p {
margin: 5px 0;
}
.game-over:hover {
color: #d32f2f;
}
JavaScript
//TypingGame.js
import React, {
useState,
useEffect
} from 'react';
import './App.css';
const sentences = [
"The quick brown fox jumps over the lazy dog.",
"Coding is fun and challenging at the same time.",
"React.js is a popular JavaScript library for building user interfaces.",
"Practice makes perfect in programming.",
"Always strive for continuous improvement in your skills.",
"Keep calm and code on!",
"Programming is a creative process of problem-solving.",
"Efficiency and readability are key factors in writing good code.",
"Success in coding requires patience and perseverance.",
"Learning new technologies opens up endless possibilities.",
];
const TypingGame = () => {
const [sentence, setSentence] = useState('');
const [input, setInput] = useState('');
const [score, setScore] = useState(0);
const [time, setTime] = useState(60);
const [isGameOver, setIsGameOver] = useState(false);
const [isGameStarted, setIsGameStarted] = useState(false);
useEffect(() => {
if (isGameStarted) {
startGame();
}
}, [isGameStarted]);
useEffect(() => {
if (time > 0 && !isGameOver && isGameStarted) {
const timer = setTimeout(() => {
setTime((prevTime) => prevTime - 1);
}, 1000);
return () => clearTimeout(timer);
} else if (time === 0 && isGameStarted) {
setIsGameOver(true);
}
}, [time, isGameOver, isGameStarted]);
const startGame = () => {
generateRandomSentence();
setTime(60);
setIsGameOver(false);
};
const generateRandomSentence = () => {
const randomIndex = Math.floor(Math.random() * sentences.length);
setSentence(sentences[randomIndex]);
};
const handleChange = (e) => {
if (!isGameOver && isGameStarted) {
setInput(e.target.value);
if (e.target.value === sentence) {
setScore((prevScore) => prevScore + 1);
setInput('');
generateRandomSentence();
}
}
};
const handleStartGame = () => {
setIsGameStarted(true);
};
return (
<div className="container">
<h1 className="title">Sentence Typing Game</h1>
{!isGameStarted && (
<button onClick={handleStartGame}
className="start-button">
Start Game
</button>
)}
{isGameStarted && (
<>
<div className="timer">Time Left: {time}</div>
<div className="sentence">{sentence}</div>
{!isGameOver && (
<div className="input-container">
<input
type="text"
value={input}
onChange={handleChange}
className="input-field"
placeholder="Type here..."
autoFocus
disabled={isGameOver}
/>
</div>
)}
</>
)}
{isGameOver && (
<div className="game-over">
<p>Game Over!</p>
<p>Your Score: {score}</p>
</div>
)}
</div>
);
};
export default TypingGame;
JavaScript
//App.js
import React from 'react';
import TypingGame from './TypingGame';
function App() {
return (
<div className="App">
<TypingGame />
</div>
);
}
export default App;
Start your application using the following command:
npm start
Output:
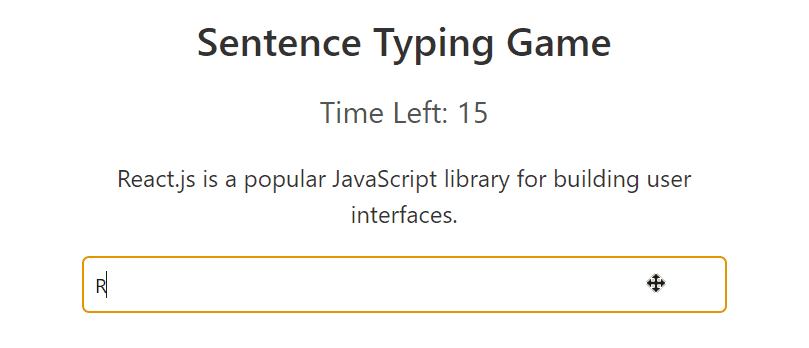
Typing Game using React
Share your thoughts in the comments
Please Login to comment...