TypeScript Object Types
Last Updated :
07 Nov, 2023
TypeScript Object Types is used to pass the object as a parameter in the functions. In which we pass properties with their specific type. the fundamental way that we group and pass around data is through objects. In TypeScript, we represent those through object types.
Syntax:
function anyName(fruit: { a: string; b: string }) {
console.log("The value of a is " + fruit.a);
console.log("The value of b is " + fruit.b);
}
anyName({ a: 'mango', b: 'apple' });
Parameters:
- anyName is the name of the function.
- a and b are properties passing as parameters with type string.
Example 1: In this example, we have a printPerson function that takes an object with a property name (a string) and marks(a number) as its parameter. We then call the ‘printPerson’ function with two anonymous objects, each representing a person with a name and marks.
Javascript
function printPerson(person: { name: string; marks: number }) {
console.log(`Name: ${person.name}, marks: ${person.marks}`);
}
printPerson({ name: "Akshit" , marks: 30 });
printPerson({ name: "Nikita" , marks: 25 });
|
Output:
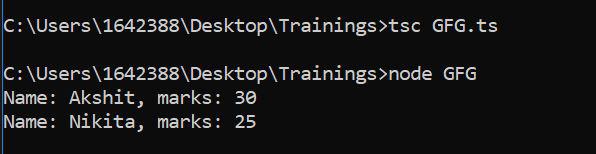
Example2: In this example, We are making marks as an optional parameter so if the value will be not provided for that we will get undefined in the console. also, we can check using if else whether the value is ubndefined or not and then we can return answer accordingly.
Javascript
function printPerson(person: { name: string; marks?: number }) {
console.log(`Name: ${person.name}, marks: ${person.marks}`);
}
printPerson({ name: "Akshit" , marks: 30 });
printPerson({ name: "Nikita" });
|
Output:
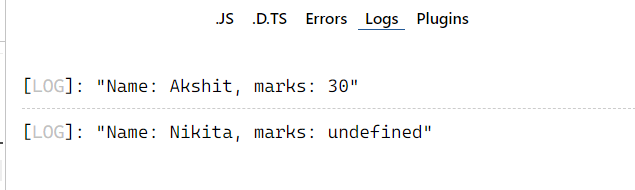
Output
Conclusion: In this article we have seen the Object type in TypeScript. Where he have seen how can we use object as a parameter in a function. Also we have seen the optional properties so that we can pass parameter optionally.
Share your thoughts in the comments
Please Login to comment...