TypeScript Object Type Optional Properties
Last Updated :
24 Oct, 2023
In this article, we are going to learn about Object Type Optional Properties in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, an object type can have optional properties, which means that some properties of the object can be present or absent when creating objects of that type. Optional properties are denoted using the ‘?’ modifier after the property name in the object type definition.
Syntax:
type TypeName = {
propertyName: PropertyType;
optionalPropertyName?: OptionalPropertyType;
};
Where-
- TypeName is the name of the object type you’re defining.
- propertyName is a required property of the object, with its type specified.
- optionalPropertyName? is an optional property of the object, denoted by the ‘?’ symbol after the property name. It can be omitted when creating objects of this type.
- OptionalPropertyType represents the type of the optional property.
Example 1: In this example, We define a Course object type with one optional property: price. We create two objects of type Course, Course1 with just the name property and Course2 with both name and price.We safely access the optional property price using conditional checks to ensure its existence before displaying its value or indicating its absence.
Javascript
type Course = {
name: string;
price?: number;
};
const Course1: Course = {
name: "Java",
};
const Course2: Course = {
name: "C++",
price: 150.00,
};
if (Course1.price !== undefined) {
console.log(`${Course1.name} costs $${Course1.price}`);
} else {
console.log(`${Course1.name} price is not specified.`);
}
if (Course2.price !== undefined) {
console.log(`${Course2.name} costs Rs${Course2.price}`);
} else {
console.log(`${Course2.name} price is not specified.`);
}
|
Output: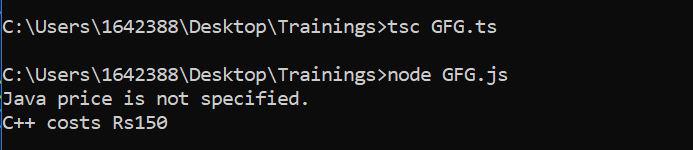
Example 2: In this example,We have the strictNullChecks option enabled, which ensures that you handle undefined and null values appropriately.We define a Person object type with two optional properties: age, which can be undefined, and address, which can be null or undefined.
Javascript
type Person = {
name: string;
age?: number;
address?: string | null ;
};
const person1: Person = {
name: "Akshit",
age: 25,
};
const person2: Person = {
name: "Bob",
address: null ,
};
if (person1.age !== undefined) {
console.log(`${person1.name}'s age is ${person1.age}`);
} else {
console.log(`${person1.name} did not provide an age.`);
}
if (person2.address !== undefined && person2.address !== null ) {
console.log(`${person2.name} lives at ${person2.address}`);
} else {
console.log(`${person2.name} did not provide an address.`);
}
|
Output:

Conclusion: In this article we have discussed Object Type Optional Properties and it’s syntax. It is used for creating an object having some optional properties. It helps in creating different kind of objects having different key and value pair properties.
Share your thoughts in the comments
Please Login to comment...