TypeScript Optional Parameters in Callbacks
Last Updated :
25 Oct, 2023
In this article, we are going to learn about Optional Parameters in Callbacks in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, optional parameters in callbacks refer to the ability to specify some of the parameters of a callback function as optional, meaning that they may or may not be provided when the callback is invoked.
Syntax:
type MyCallback = (param1: string, param2?: number) => void;
function processCallback(callback: MyCallback, param1: string, param2?: number) {
callback(param1, param2);
}
Where-
- MyCallback is a type representing a callback function with a required param1 (string) and an optional param2 (number).
- processCallback is a function that accepts a callback of type MyCallback and optional parameters param1 (string) and param2 (number). It calls the callback function with the provided parameters.
Example 1: In this example, We define the MyCallback type, where param2 is an optional parameter. The function callback function takes two parameters, str (a required string) and num (an optional number). Inside the function, we log the received string and, if num is provided (i.e., not undefined), we also log the number. Otherwise, we indicate that the number is not provided. We call the myFunction callback twice: first with only the string parameter and then with both the string and number parameters.
Javascript
type MyCallback = (param1: string, param2?: number) => void;
const myFunction: MyCallback = (str, num) => {
console.log(`Received: ${str}`);
if (num !== undefined) {
console.log(`Number: ${num}`);
} else {
console.log( "Number is not provided." );
}
};
myFunction( "GeeksforGeeks" );
myFunction( "A Computer Science Portal" , 99);
|
Output: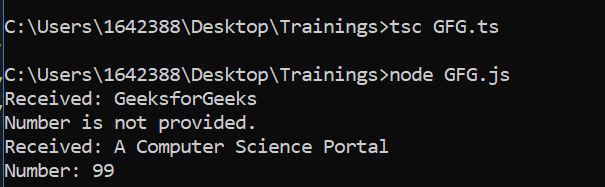
Example 2: In this example, We define a MathOperationCallback type for callback functions that perform mathematical operations on two numbers, with an optional description parameter.The performMathOperation function takes a callback function, two numbers (a and b), and an optional description parameter.
Javascript
type MathOperationCallback = (a: number, b: number,
description?: string) => number;
function performMathOperation(
operation: MathOperationCallback,
a: number,
b: number,
description?: string
) {
const result = operation(a, b);
console.log(`Operation: ${description || "Unnamed" }`);
console.log(`Result: ${result}`);
}
const customAddition: MathOperationCallback = (x, y, description) => {
const sum = x + y;
console.log(`Performing addition: ${description || "Unnamed" }`);
return sum;
};
performMathOperation(customAddition, 5, 3);
performMathOperation(customAddition, 10, 7, "Adding Numbers" );
|
Output: 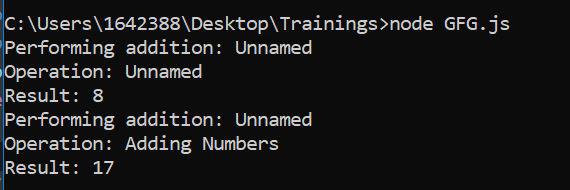
Share your thoughts in the comments
Please Login to comment...