TypeScript Object Type Excess Property Checks
Last Updated :
25 Oct, 2023
In this article, we are going to learn about Object Type Index Signatures in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, excess property checks refer to the behavior where TypeScript checks for extra or unexpected properties when you try to assign an object to a type with specific known properties.
Syntax:
type MyType = {
knownProperty1: Type1;
knownProperty2: Type2;
// . . .
};
Where-
- MyType is the name of the object type you are defining.
- knownProperty1, knownProperty2, and so on, are the properties with known names and their respective types.
- Type1, Type2, and so on, are the data types for the known properties.
Example 1: In this example, we define a Person type with two properties name and age. When we create the person object, we attempt to add an extra property email. TypeScript raises an error because the object literal is trying to specify properties that are not part of the Person type.
Javascript
type Person = {
name: string;
age: number;
};
const person: Person = {
name: "Alice" ,
age: 30,
email: "alice@example.com" ,
};
|
Output:
Example 2: In this example we are assigning the object to another variable,To work with objects that might have extra properties, you can use the object spread syntax (…) or explicitly assert the object’s type.
Javascript
type Person = {
name: string;
age: number;
};
const person: Person = {
name: "Alice" ,
age: 30,
...{ email: "alice@geeksforgeeks.org" },
};
console.log(person)
|
Output: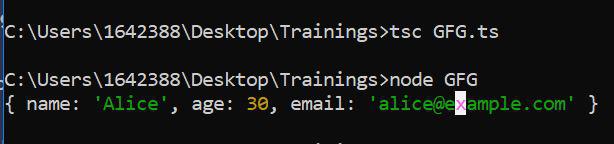
Example 3: In this example we are using type assertion. We use a type assertion (personWithExtraInfo as Person) to assert that personWithExtraInfo conforms to the Person type. This allows us to assign it to a variable of type Person (person). We can safely access the name and age properties on the person object because they are part of the Person type. If we try to access the city property, TypeScript will raise a compilation error because it’s not part of the Person type.
Javascript
type Person = {
name: string;
age: number;
};
const personWithExtraInfo = {
name: "Alice" ,
age: 30,
city: "Wonderland" ,
};
const person: Person = personWithExtraInfo as Person;
console.log(person.name);
console.log(person.age);
|
Output: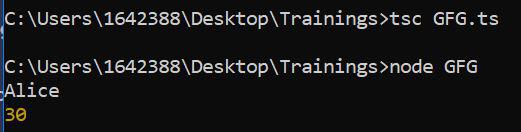
Example 4: In this example we are using String Index Signature. We have declared only one property name in the type. Other properties are declared using String Index signature.
Javascript
type Person = {
name: string;
[key: string]: any;
};
const person: Person = {
name: "GeeksforGeeks" ,
portal: "Computer Science Portal" ,
city: "Noida" ,
};
console.log(`Name: ${person.name}`);
console.log(`Portal: ${person.portal}`);
console.log(`City: ${person.city}`);
|
Output:
Share your thoughts in the comments
Please Login to comment...