TypeScript Object Type Property Modifiers
Last Updated :
06 Nov, 2023
TypeScript Type Property Modifiers are used to specify each property in an object such as: the type, whether the property is optional, and whether the property can be written to.
TypeScript Object Type Property Modifiers:
- readonly Properties: An object type with readonly properties specifies that the properties of the object cannot be modified after their initial assignment, ensuring immutability and preventing accidental changes to the object’s values.
- Index Signatures: It allows you to define object types with dynamic keys, where the keys can be of a specific type, and the corresponding values can be of another type. This is particularly useful when you want to work with objects that have properties that are not known at compile-time but follow a specific pattern.
- Optional Properties: An object type can have optional properties, which means that some properties of the object can be present or absent when creating objects of that type. Optional properties are denoted using the ‘?’ modifier after the property name in the object type definition.
Example 1: In this example, Point is an object type with two read-only properties, x and y. When we attempt to modify the x property, TypeScript raises an error because it’s read-only.
Javascript
type Point = {
readonly x: number;
readonly y: number;
};
const point: Point = { x: 10, y: 20 };
console.log(point)
|
Output: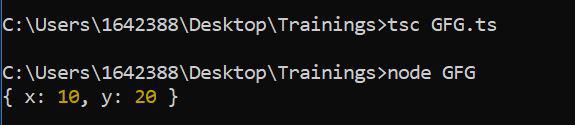
Example 2: In this example, Dictionary is an object type with an index signature [word: string]: string. which means it can have keys of type string and values of type string. myDictionary is an instance of a Dictionary with three key-value pairs. You can access and modify values using dynamic keys within the specified type constraints.
Javascript
type Dictionary = {
[word: string]: string;
};
const myDictionary: Dictionary = {
"apple" : "a fruit" ,
"banana" : "another fruit" ,
"car" : "a vehicle" ,
};
console.log(myDictionary[ "apple" ]);
|
Output: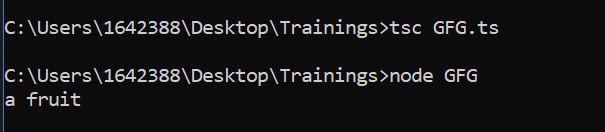
Example 3: In this example, We define a Course object type with one optional property: price. We create two objects of type Course, Course1 with just the name property and Course2 with both name and price. We safely access the optional property price using conditional checks to ensure its existence before displaying its value or indicating its absence.
Javascript
type Course = {
name: string;
price?: number;
};
const Course1: Course = {
name: "Java" ,
};
const Course2: Course = {
name: "C++" ,
price: 150.00,
};
if (Course1.price !== undefined) {
console.log(
`${Course1.name} costs $${Course1.price}`);
} else {
console.log(
`${Course1.name} price is not specified.`);
}
if (Course2.price !== undefined) {
console.log(
`${Course2.name} costs Rs${Course2.price}`);
} else {
console.log(
`${Course2.name} price is not specified.`);
}
|
Output:
Conclusion: In this article, we have seen Object Type Property Modifiers and it’s type with examples. They are used in property check in Typescript and it also make code more readable.
Reference: https://www.typescriptlang.org/docs/handbook/2/objects.html#property-modifiers
Share your thoughts in the comments
Please Login to comment...