TypeScript Generic Parameter Defaults
Last Updated :
03 Nov, 2023
TypeScript Generic Parameter Defaults are used to specify default values for generic type parameters in order to provide a fallback type when a type argument is not explicitly provided during instantiation. This allows you to make your generic types more flexible and easier to use in situations where type inference may not be straightforward.
Syntax:
function myFunction<T = DefaultType>(param: T): void {
// Function implementation
}
Where:
- T: This is the generic type parameter that can take any type.
- DefaultType: This is the default type that will be used for T if no type argument is provided during function or class instantiation.
A generic parameter default follows the following rules:
- When we provide a default parameter in the function, then it becomes optional for us to provide the type of the object
- Optional type parameters must not be followed by Required type parameters.
- All the constraints must be followed for the type parameter while creating default types for a type parameter.
- You are only required to specify type arguments for the type parameters that are required others will be resolved to default.
- The default type is inferred when a default type is specified and inference cannot choose a candidate.
- If a class or interface has a default value type and it merges with another class or interface then that also has the same default type, Also, it can introduce a new parameter type as long as it specifies a default.
Example 1: In this example, we will see how to specify the default type for a generic parameter when declaring a generic function. If you don’t provide a type argument when calling a generic function or instantiating a generic class, TypeScript will use the default type as the inferred type.
Javascript
function echo<T = string>(value: T): T {
return value;
}
const result = echo(42);
console.log(result)
|
Output:
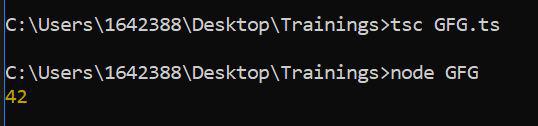
Example 2: In this example, we are checking whether value is given or not at the time of function invocation. if value is not given then it is returning the default value.
Javascript
function firstOrDefault<T = null >(array: T[], defaultValue: T): T {
return array.length > 0 ? array[0] : defaultValue;
}
const numbers: number[] = [1, 2, 3];
const firstNumber = firstOrDefault(numbers, 0);
console.log(firstNumber);
const emptyArray: string[] = [];
const firstString = firstOrDefault<string>(emptyArray, 'No elements' );
console.log(firstString);
|
Output:
Conclusion: In this article we have seen Generic Parameter Defaults and it’s syntax. Also we have seen what rules we have to follow. This helps in gettimg a default parameter value. Also it helps in code formatting and it adds another feature in robust typescript.
Share your thoughts in the comments
Please Login to comment...