TypeScript Using Class Types in Generics
Last Updated :
02 Nov, 2023
TypeScript Using class types in generics allows you to create more flexible and reusable code by specifying that a generic parameter should be a class constructor. This is particularly useful when you want to work with instances of classes but want to keep the code type safe. You can define a generic type that accepts class constructors and then use that type to create and work with instances of those classes.
Syntax
function createInstance<T>(
constructor: new (...args: any[]) => T,
...args: any[]
): T {
// Create and return an instance of the specified class
return new constructor(...args);
}
Where:
- T is a generic type parameter that represents the type of the instance to be created. It allows you to specify the expected return type of the factory function.
- constructor is a reference to the class constructor.
- new (…args: any[]) => T, indicating that it should accept any number of arguments (…args: any[]) and return an instance of type T.
- …args: any[] uses the rest parameter syntax (…args) to accept any number of additional arguments. These arguments are passed to the constructor when creating the instance.
Example 1: In this example, We define the createInstance function, which is a generic factory function. It accepts a class constructor (constructor) and any number of additional arguments (…args). The createInstance function creates an instance of the specified class by invoking the constructor with the provided arguments.
Javascript
function createInstance<T>(constructor:
new (...args: any[]) => T, ...args: any[]): T {
return new constructor(...args);
}
class Product {
constructor(
public name: string,
public price: number) { }
}
class Person {
constructor(
public name: string,
public age: number) { }
}
const laptop =
createInstance(Product, 'Laptop' , 999);
console.log(laptop);
const user =
createInstance(Person, 'GeeksforGeeks' , 30);
console.log(user);
|
Output: 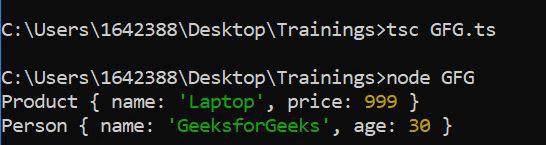
Example 2: In this example,We define a base class Animal with a name property and a makeSound method. The Dog class extends Animal and adds a breed property and overrides the makeSound method. We create a generic function printAnimalInfo that takes an argument of type T, where T extends the Animal class. This means it can accept instances of Animal or any class that extends it. We create instances of both Animal and Dog. We call the printAnimalInfo function with both instances..
Javascript
class Animal {
constructor(public name: string) { }
makeSound(): string {
return "Some generic animal sound" ;
}
}
class Dog extends Animal {
constructor(name: string, public breed: string) {
super (name);
}
makeSound(): string {
return "Woof! Woof!" ;
}
}
function printAnimalInfo<T extends Animal>
(animal: T): void {
console.log(`Name: ${animal.name}`);
console.log(`Sound: ${animal.makeSound()}`);
}
const genericAnimal = new Animal( "Generic Animal" );
const myDog = new Dog( "Buddy" , "Golden Retriever" );
printAnimalInfo(genericAnimal);
printAnimalInfo(myDog);
|
Output: 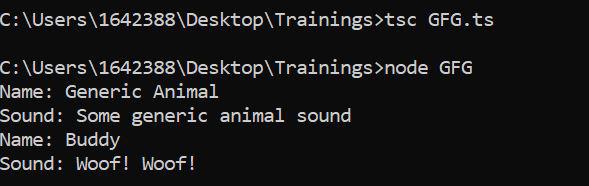
Reference: https://www.typescriptlang.org/docs/handbook/2/generics.html#using-class-types-in-generics
Share your thoughts in the comments
Please Login to comment...