TypeScript Working with Generic Type Variables
Last Updated :
08 Nov, 2023
In TypeScript, working with generic type variables is a powerful feature that allows you to create functions and classes that can work with a variety of data types while maintaining type safety.
Note: When you declare a generic function or class, you introduce a type variable enclosed in angle brackets (<>). This type variable serves as a placeholder for a specific type that will be provided when the function or class is used. This enables you to create more flexible and reusable code.
Syntax:
function identity<Type>(arg: Type): Type {
// Body of function
}
Parameters:
- Type is the type of datatype.
Example 1: In this example, we have created an identity function that is a generic function. we will first pass a string to this and then pass a number to this. Our function is able to infer the type of variable and print it. We are also printing its type using the ‘typeof()’ function.
Javascript
function identity<Type>(arg: Type): Type {
return arg;
}
let result = identity( "GeeksforGeeks" );
let result2 = identity(42);
console.log(result + " " + typeof result);
console.log(result2 + " " + typeof result2);
|
Output:

Example 2: In this example, we will see what if we want to log the length of the argument arg to the console with each call. This will give us error. Make note that these type variables stand in for any and all types, so someone using this function could have passed in a number instead, which does not have a .length member. so, instead of Type we have to use Type[] as a parameter.
Javascript
function identity<Type>(arg: Type): Type {
return arg.length;
}
let result = identity(44);
console.log(result)
|
Output:
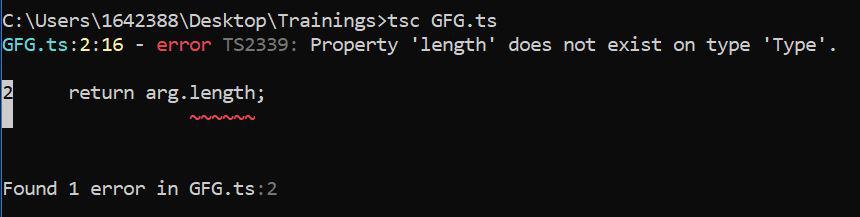
Conclusion: In this article we learnt that about working with generic types in typescript. In TypeScript, working with generic type variables is a powerful feature that allows you to create functions and classes that can work with a variety of data types while maintaining type safety.
Share your thoughts in the comments
Please Login to comment...