TypeScript Generic Functions
Last Updated :
24 Oct, 2023
TypeScript is a powerful superset of JavaScript that adds static typing to the language. Generic functions enable you to write reusable code that adapts to different data types without sacrificing the benefits of static typing.
Syntax:
function functionName<T>(parameterName: T): ReturnType {
// the implementation
}
Where-
- functionName is the name of your generic function.
- <T> is the type parameter, T, allowing you to work with different data types within the function.
- parameterName represents the name of the function’s parameter, and T designates the type of data the parameter will accept.
- ReturnType specifies the expected type for the function’s return value.
Example: Generic Function with a single type parameter is a basic generic function in TypeScript, that can work with different data types while maintaining type safety. The type parameter represents the type of the argument and return value. When calling the generic function, you specify the type explicitly or let TypeScript infer it.
Javascript
function gfg<T>(arg: T): T {
return arg;
}
let result1: string = gfg<string>( "GEEKSFORGEEKS" );
let result2: number = gfg<number>(740);
let result3: boolean = gfg( false );
console.log(result1);
console.log(result2);
console.log(result3);
|
Output:
Example: A generic function with an array parameter allows you to work with arrays of different types. By using a type parameter, the function can handle arrays of various element types while maintaining type safety.
Javascript
function arrayEl<T>(arr: T[]): void {
for (const x of arr) {
console.log(x);
}
}
let elements: number[] = [101, 102, 103];
arrayEl(elements);
let elements1: string[] = [ "Geeks" , "For" , "Geeks" ];
arrayEl(elements1);
|
Output: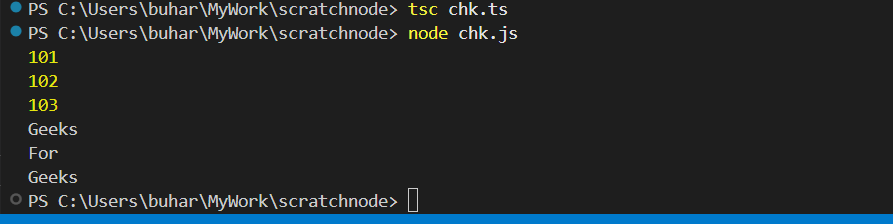
Conclusion: In TypeScript, generic functions let you build versatile functions that work with different data types while ensuring your code stays error-free. This flexibility is incredibly handy when you want to create functions that can handle a variety of situations without repeating code. Whether you’re working with arrays, objects, or any data structure, generic functions empower you to write cleaner and safer code.
Share your thoughts in the comments
Please Login to comment...