Task Scheduling App with Node and Express.js
Last Updated :
12 Mar, 2024
Task Scheduling app is an app that can be used to create, update, delete, and view all the tasks created. It is implemented using NodeJS and ExpressJS. The scheduler allows users to add tasks in the cache of the current session, once the app is reloaded the data gets deleted. This can be scaled using databases like MongoDB and authentication and validation can be added as well.
In this article, we view a simple implementation of a popular example that allows readers to learn how to set up a backend server and create custom APIs using Node and Express.
Output Preview: Let us have a look at how the final output will look like.
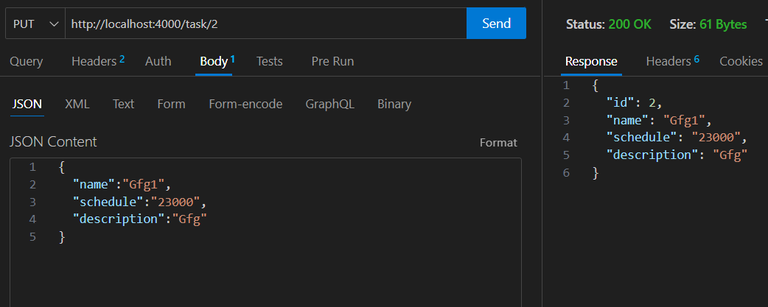
Task Scheduling App with NodeJS and ExpressJS
Prerequisites:
Approach to Create a Task Scheduling App:
- Create – A new task can be added.
- Update – A task can be updated when provided with an Id of the task
- Delete – A task can be deleted when provided with an Id of the task
- Get One Task – A task can be fetched when provided with an Id of the task
- Get All Tasks – All tasks created can be fetched.
- Import the necessary files. Initialize the express app and port to which the local server listens to.
- Set up a middleware using body-parser of express to parse JSON requests.
- Initialize a tasks array that is going to store all the tasks created by a user.
- Write the logic to push the new task created to the task array. This listens to route “/task” using a POST method.
- Write the logic to edit the task by using its id as reference. This listens to route “/task/:id” using a PUT method.
- Write the logic to fetch a single task using its id as reference. This listens to route “/task/:id” using a GET method.
- Write the logic to delete a task using id from the task array. This listens to route “/task/:id” using a DELETE method.
Steps to Create the NodeJS App and Installing Module
Step 1: Create the backend project by navigating to the folder and typing this command in the terminal:
npm init -y
Step 2: Install express using the following command and create an index.js file
npm install express
Project Structure:
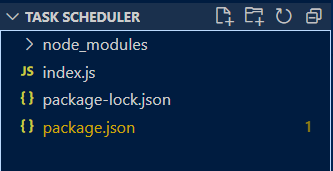
Project Structure
Example: Below is an example of creating a task Scheduling App with NodeJS and ExpressJS.
Javascript// server.js
const express = require('express');
const app = express();
const port = 4000;
const bodyParser = require('body-parser');
// Middleware to parse JSON requests
app.use(bodyParser.json());
// Task list
let tasks = [];
// Function to execute tasks
const executeTask = (task) => {
console.log(`Executing task: ${task.name}`);
// Add your task execution logic here
};
// Schedule tasks
const scheduleTasks = () => {
tasks.forEach(task => {
setTimeout(() => {
executeTask(task);
}, task.schedule);
});
};
// Route to add a new task
app.post('/task', (req, res) => {
const { name, schedule, description } = req.body;
if (!name || !schedule) {
return res.status(400).send(
'Both name and schedule are required');
}
const task = {
id: tasks.length + 1,
name,
schedule,
description
};
tasks.push(task);
res.status(201).json(task);
});
// Route to edit a task
app.put('/task/:id', (req, res) => {
const taskId = parseInt(req.params.id);
const { name, schedule, description } = req.body;
const taskIndex = tasks.findIndex(
task => task.id === taskId);
if (taskIndex === -1) {
return res.status(404).send(
'Task not found');
}
if (!name || !schedule) {
return res.status(400).send(
'Both name and schedule are required');
}
tasks[taskIndex] = {
...tasks[taskIndex],
name,
schedule,
description
};
res.json(tasks[taskIndex]);
});
// Route to delete a task
app.delete('/task/:id', (req, res) => {
const taskId = parseInt(req.params.id);
const taskIndex = tasks.findIndex(
task => task.id === taskId);
if (taskIndex === -1) {
return res.status(404).send(
'Task not found');
}
const deletedTask = tasks.splice(
taskIndex, 1);
res.json(deletedTask[0]);
});
// Route to get all tasks
app.get('/tasks', (req, res) => {
res.json(tasks);
});
// Route to get a task by ID
app.get('/task/:id', (req, res) => {
const taskId = parseInt(req.params.id);
const task = tasks.find(
task => task.id === taskId);
if (task) {
res.json(task);
} else {
res.status(404).send(
'Task not found');
}
});
// Start the scheduler
scheduleTasks();
// Start the server
app.listen(port, () => {
console.log(
`Task scheduler app listening at http://localhost:${port}`);
});
Start your server using the following command.
node server.js
Output:
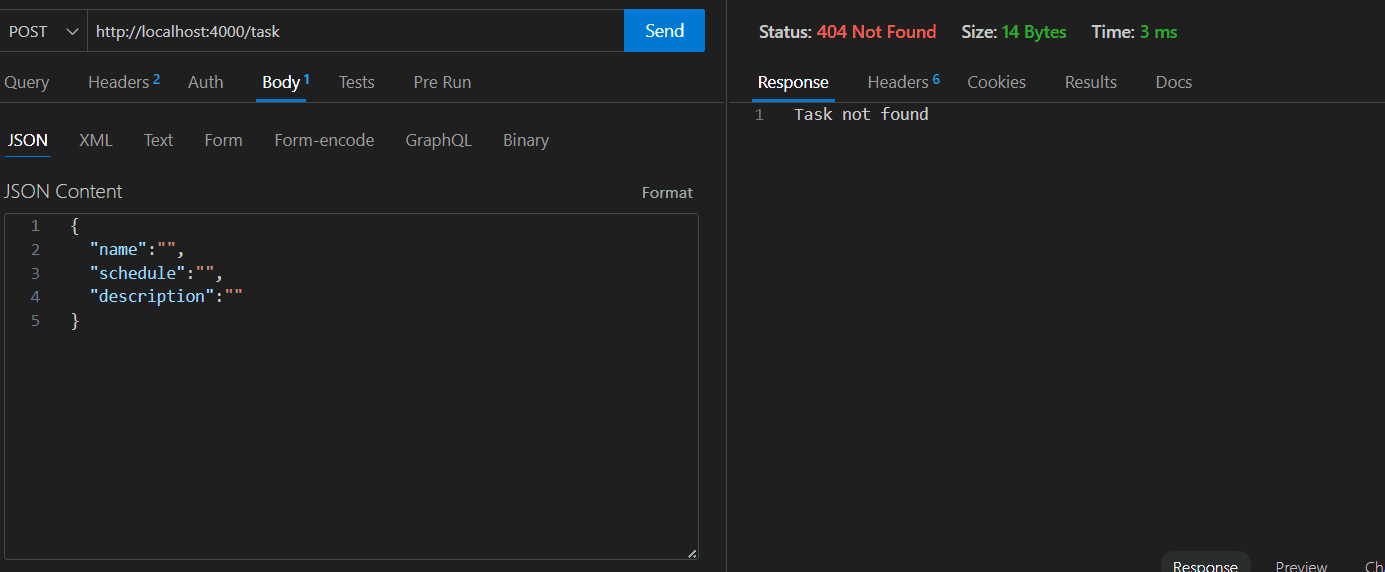
Task Scheduling App with NodeJS and ExpressJS
Share your thoughts in the comments
Please Login to comment...