Spring Cloud – Create Your Custom Load Balancer
Last Updated :
10 Jun, 2023
Spring Cloud is a collection of projects like load balancing, service discovery, circuit breakers, routing, micro-proxy, etc will be given by Spring Cloud. So spring Cloud basically provides some of the common tools and techniques and projects to quickly develop some common patterns of the microservices.
Taking about the general definition, Load Balancer is a network device that sits between a set of backend servers and clients. It distributes the incoming traffic to multiple servers to reduce the load. Load Balancers typically use various algorithms, such as round-robin to determine which server to send incoming traffic to. Please refer to the below image and observe how the above problem is fixed by Load Balancer.
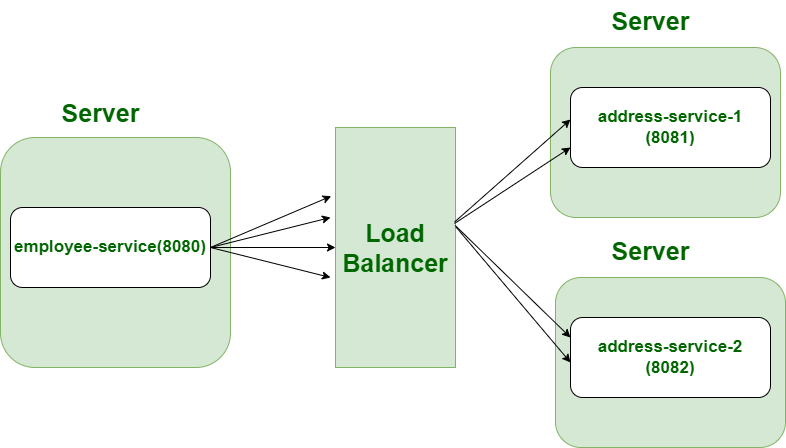
So, in Spring, if you want to use Load Balancer then Spring Cloud provides us with some already developed ready-made Load Balancer. By using this you don’t need to write any Load Balancer or create your own, you can just use the tool very easily by importing some dependencies and writing minimal code. There are two ways to load balance the request
- Client-Side Load Balancer
- Server-Side Load Balancer
Examples:
- Client-Side Load Balancer Example: Spring Cloud Load Balancer, Netflix Ribbon
- Server-Side Load Balancer Example: Spring Cloud Gateway, Netflix Zuul
In Spring it is possible that we can create our custom Load Balancer using ReactiveLoadBalancer. The ReactiveLoadBalancer implementation that is used by default is RoundRobinLoadBalancer. Let’s see the implementation through an example.
Example
Create a CustomLoadBalancerConfig class. Below is the code for CustomLoadBalancerConfig.class file.
Java
import org.springframework.cloud.client.ServiceInstance;
import org.springframework.cloud.loadbalancer.core.RandomLoadBalancer;
import org.springframework.cloud.loadbalancer.core.ReactorLoadBalancer;
import org.springframework.cloud.loadbalancer.core.ServiceInstanceListSupplier;
import org.springframework.cloud.loadbalancer.support.LoadBalancerClientFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.core.env.Environment;
public class CustomLoadBalancerConfig {
@Bean
ReactorLoadBalancer<ServiceInstance> randomLoadBalancer(Environment environment,
LoadBalancerClientFactory loadBalancerClientFactory) {
String name = environment.getProperty(LoadBalancerClientFactory.PROPERTY_NAME);
return new RandomLoadBalancer(loadBalancerClientFactory
.getLazyProvider(name, ServiceInstanceListSupplier. class ),
name);
}
}
|
Create another class named DemoServiceLoadBalancer. Annotate the class with @LoadBalancerClient annotation with these parameters.
@LoadBalancerClient(name = "demo-service", configuration = CustomLoadBalancerConfig.class)
Inside the class Create Feign Client Bean and annotate it with @Bean and @LoadBalanced annotation.
@Bean
@LoadBalanced
public Feign.Builder feignBuilder() {
return Feign.builder();
}
Below is the complete code for DemoServiceLoadBalancer.class file.
Java
import feign.Feign;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.cloud.loadbalancer.annotation.LoadBalancerClient;
import org.springframework.context.annotation.Bean;
@LoadBalancerClient (name = "demo-service" , configuration = CustomLoadBalancerConfig. class )
public class DemoServiceLoadBalancer {
@Bean
@LoadBalanced
public Feign.Builder feignBuilder() {
return Feign.builder();
}
}
|
And this is how you can create your Custom Load Balancer in Spring Cloud.
Share your thoughts in the comments
Please Login to comment...