In Java, HashMap is a part of Java’s collection since Java 1.2. This class is found in java.util package. It provides the basic implementation of the Map interface of Java. HashMap in Java stores the data in (Key, Value) pairs, and you can access them by an index of another type (e.g. an Integer). One object is used as a key (index) to another object (value). If you try to insert the duplicate key in HashMap, it will replace the element of the corresponding key.Â
What is HashMap?
Java HashMap is similar to HashTable, but it is unsynchronized. It allows to store the null keys as well, but there should be only one null key object and there can be any number of null values. This class makes no guarantees as to the order of the map. To use this class and its methods, you need to import java.util.HashMap package or its superclass.
Java HashMap Examples
Below is the implementation of an example of Java HashMap:
Java
import java.util.HashMap;
public class GFG {
public static void main(String[] args)
{
HashMap<String, Integer> map = new HashMap<>();
map.put( "vishal" , 10 );
map.put( "sachin" , 30 );
map.put( "vaibhav" , 20 );
System.out.println( "Size of map is:- "
+ map.size());
System.out.println(map);
if (map.containsKey( "vishal" )) {
Integer a = map.get( "vishal" );
System.out.println( "value for key"
+ " \"vishal\" is:- " + a);
}
}
}
|
Output
Size of map is:- 3
{vaibhav=20, vishal=10, sachin=30}
value for key "vishal" is:- 10
 HashMap Declaration
public class HashMap<K,V> extends AbstractMap<K,V>
implements Map<K,V>, Cloneable, Serializable
Parameters:
It takes two parameters namely as follows:
- The type of keys maintained by this map
- The type of mapped values
Note: Keys and value can’t be primitive datatype. Key in Hashmap is valid if it implements hashCode() and equals() method , it should also be immutable (immutable custom object ) so that hashcode and equality remains constant. Value in hashmap can be any wrapper class, custom objects, arrays, any reference type or even null .
For example : Hashmap can have array as value but not as key.
HashMap in Java implements Serializable, Cloneable, Map<K, V> interfaces.Java HashMap extends AbstractMap<K, V> class. The direct subclasses are LinkedHashMap and PrinterStateReasons.
Hierarchy of Java HashMap
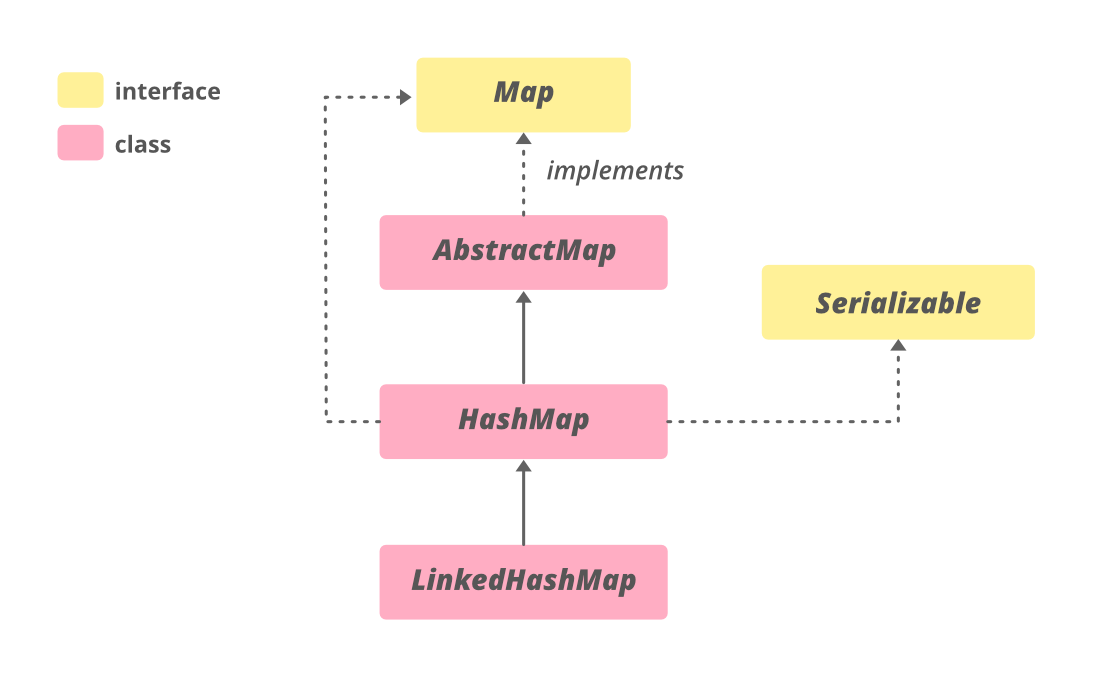
Characteristics of Java HashMap
A HashMap is a data structure that is used to store and retrieve values based on keys. Some of the key characteristics of a hashmap include:
- Fast access time: HashMaps provide constant time access to elements, which means that retrieval and insertion of elements are very fast, usually O(1) time complexity.
- Uses hashing function: HashMaps uses a hash function to map keys to indices in an array. This allows for a quick lookup of values based on keys.
- Stores key-value pairs: Each element in a HashMap consists of a key-value pair. The key is used to look up the associated value.
- Supports null keys and values: HashMaps allow for null values and keys. This means that a null key can be used to store a value, and a null value can be associated with a key.
- Not ordered: HashMaps are not ordered, which means that the order in which elements are added to the map is not preserved. However, LinkedHashMap is a variation of HashMap that preserves the insertion order.
- Allows duplicates: HashMaps allow for duplicate values, but not duplicate keys. If a duplicate key is added, the previous value associated with the key is overwritten.
- Thread-unsafe: HashMaps are not thread-safe, which means that if multiple threads access the same hashmap simultaneously, it can lead to data inconsistencies. If thread safety is required, ConcurrentHashMap can be used.
- Capacity and load factor: HashMaps have a capacity, which is the number of elements that it can hold, and a load factor, which is the measure of how full the hashmap can be before it is resized.
Creating HashMap in Java
Let us understand how we can create HashMap in Java with an example mentioned below:
Java
import java.util.HashMap;
public class ExampleHashMap {
public static void main(String[] args) {
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put( "John" , 25 );
hashMap.put( "Jane" , 30 );
hashMap.put( "Jim" , 35 );
System.out.println(hashMap.get( "John" ));
hashMap.remove( "Jim" );
System.out.println(hashMap.containsKey( "Jim" ));
System.out.println(hashMap.size());
}
}
|
Java HashMap Constructors
HashMap provides 4 constructors and the access modifier of each is public which are listed as follows:
- HashMap()
- HashMap(int initialCapacity)
- HashMap(int initialCapacity, float loadFactor)
- HashMap(Map map)
Now discussing the above constructors one by one alongside implementing the same with help of clean Java programs.
1. HashMap()
It is the default constructor which creates an instance of HashMap with an initial capacity of 16 and a load factor of 0.75.
Syntax:
HashMap<K, V> hm = new HashMap<K, V>();
Example
Java
import java.io.*;
import java.util.*;
class GFG {
public static void main(String args[])
{
HashMap<Integer, String> hm1 = new HashMap<>();
HashMap<Integer, String> hm2
= new HashMap<Integer, String>();
hm1.put( 1 , "one" );
hm1.put( 2 , "two" );
hm1.put( 3 , "three" );
hm2.put( 4 , "four" );
hm2.put( 5 , "five" );
hm2.put( 6 , "six" );
System.out.println( "Mappings of HashMap hm1 are : "
+ hm1);
System.out.println( "Mapping of HashMap hm2 are : "
+ hm2);
}
}
|
Output
Mappings of HashMap hm1 are : {1=one, 2=two, 3=three}
Mapping of HashMap hm2 are : {4=four, 5=five, 6=six}
2. HashMap(int initialCapacity)
It creates a HashMap instance with a specified initial capacity and load factor of 0.75.
Syntax:
HashMap<K, V> hm = new HashMap<K, V>(int initialCapacity);
Example
Java
import java.io.*;
import java.util.*;
class AddElementsToHashMap {
public static void main(String args[])
{
HashMap<Integer, String> hm1 = new HashMap<>( 10 );
HashMap<Integer, String> hm2
= new HashMap<Integer, String>( 2 );
hm1.put( 1 , "one" );
hm1.put( 2 , "two" );
hm1.put( 3 , "three" );
hm2.put( 4 , "four" );
hm2.put( 5 , "five" );
hm2.put( 6 , "six" );
System.out.println( "Mappings of HashMap hm1 are : "
+ hm1);
System.out.println( "Mapping of HashMap hm2 are : "
+ hm2);
}
}
|
Output
Mappings of HashMap hm1 are : {1=one, 2=two, 3=three}
Mapping of HashMap hm2 are : {4=four, 5=five, 6=six}
3. HashMap(int initialCapacity, float loadFactor)
It creates a HashMap instance with a specified initial capacity and specified load factor.
Syntax:
HashMap<K, V> hm = new HashMap<K, V>(int initialCapacity, float loadFactor);
Example
Java
import java.io.*;
import java.util.*;
class GFG {
public static void main(String args[])
{
HashMap<Integer, String> hm1
= new HashMap<>( 5 , 0 .75f);
HashMap<Integer, String> hm2
= new HashMap<Integer, String>( 3 , 0 .5f);
hm1.put( 1 , "one" );
hm1.put( 2 , "two" );
hm1.put( 3 , "three" );
hm2.put( 4 , "four" );
hm2.put( 5 , "five" );
hm2.put( 6 , "six" );
System.out.println( "Mappings of HashMap hm1 are : "
+ hm1);
System.out.println( "Mapping of HashMap hm2 are : "
+ hm2);
}
}
|
Output
Mappings of HashMap hm1 are : {1=one, 2=two, 3=three}
Mapping of HashMap hm2 are : {4=four, 5=five, 6=six}
 4. HashMap(Map map)
It creates an instance of HashMap with the same mappings as the specified map.
HashMap<K, V> hm = new HashMap<K, V>(Map map);
Java
import java.io.*;
import java.util.*;
class AddElementsToHashMap {
public static void main(String args[])
{
Map<Integer, String> hm1 = new HashMap<>();
hm1.put( 1 , "one" );
hm1.put( 2 , "two" );
hm1.put( 3 , "three" );
HashMap<Integer, String> hm2
= new HashMap<Integer, String>(hm1);
System.out.println( "Mappings of HashMap hm1 are : "
+ hm1);
System.out.println( "Mapping of HashMap hm2 are : "
+ hm2);
}
}
|
Output
Mappings of HashMap hm1 are : {1=one, 2=two, 3=three}
Mapping of HashMap hm2 are : {1=one, 2=two, 3=three}
1. Adding Elements in HashMap in Java
In order to add an element to the map, we can use the put() method. However, the insertion order is not retained in the Hashmap. Internally, for every element, a separate hash is generated and the elements are indexed based on this hash to make it more efficient.
Java
import java.io.*;
import java.util.*;
class AddElementsToHashMap {
public static void main(String args[])
{
HashMap<Integer, String> hm1 = new HashMap<>();
HashMap<Integer, String> hm2
= new HashMap<Integer, String>();
hm1.put( 1 , "Geeks" );
hm1.put( 2 , "For" );
hm1.put( 3 , "Geeks" );
hm2.put( 1 , "Geeks" );
hm2.put( 2 , "For" );
hm2.put( 3 , "Geeks" );
System.out.println( "Mappings of HashMap hm1 are : "
+ hm1);
System.out.println( "Mapping of HashMap hm2 are : "
+ hm2);
}
}
|
Output
Mappings of HashMap hm1 are : {1=Geeks, 2=For, 3=Geeks}
Mapping of HashMap hm2 are : {1=Geeks, 2=For, 3=Geeks}
2. Changing Elements in HashMap in Java
After adding the elements if we wish to change the element, it can be done by again adding the element with the put() method. Since the elements in the map are indexed using the keys, the value of the key can be changed by simply inserting the updated value for the key for which we wish to change.
Java
import java.io.*;
import java.util.*;
class ChangeElementsOfHashMap {
public static void main(String args[])
{
HashMap<Integer, String> hm
= new HashMap<Integer, String>();
hm.put( 1 , "Geeks" );
hm.put( 2 , "Geeks" );
hm.put( 3 , "Geeks" );
System.out.println( "Initial Map " + hm);
hm.put( 2 , "For" );
System.out.println( "Updated Map " + hm);
}
}
|
Output
Initial Map {1=Geeks, 2=Geeks, 3=Geeks}
Updated Map {1=Geeks, 2=For, 3=Geeks}
3. Removing Element from Java HashMap
In order to remove an element from the Map, we can use the remove() method. This method takes the key value and removes the mapping for a key from this map if it is present in the map.
Java
import java.io.*;
import java.util.*;
class RemoveElementsOfHashMap{
public static void main(String args[])
{
Map<Integer, String> hm
= new HashMap<Integer, String>();
hm.put( 1 , "Geeks" );
hm.put( 2 , "For" );
hm.put( 3 , "Geeks" );
hm.put( 4 , "For" );
System.out.println( "Mappings of HashMap are : "
+ hm);
hm.remove( 4 );
System.out.println( "Mappings after removal are : "
+ hm);
}
}
|
Output
Mappings of HashMap are : {1=Geeks, 2=For, 3=Geeks, 4=For}
Mappings after removal are : {1=Geeks, 2=For, 3=Geeks}
 4. Traversal of Java HashMap
We can use the Iterator interface to traverse over any structure of the Collection Framework. Since Iterators work with one type of data we use Entry< ? , ? > to resolve the two separate types into a compatible format. Then using the next() method we print the entries of HashMap.
Java
import java.util.HashMap;
import java.util.Map;
public class TraversalTheHashMap {
public static void main(String[] args)
{
HashMap<String, Integer> map = new HashMap<>();
map.put( "vishal" , 10 );
map.put( "sachin" , 30 );
map.put( "vaibhav" , 20 );
for (Map.Entry<String, Integer> e : map.entrySet())
System.out.println( "Key: " + e.getKey()
+ " Value: " + e.getValue());
}
}
|
Output
Key: vaibhav Value: 20
Key: vishal Value: 10
Key: sachin Value: 30
Complexity of HashMap in Java
HashMap provides constant time complexity for basic operations, get and put if the hash function is properly written and it disperses the elements properly among the buckets. Iteration over HashMap depends on the capacity of HashMap and the number of key-value pairs. Basically, it is directly proportional to the capacity + size. Capacity is the number of buckets in HashMap. So it is not a good idea to keep a high number of buckets in HashMap initially.
Adding Elements in HashMap
|
O(1)
|
O(N)
|
Removing Element from HashMap
|
O(1)
|
O(N)
|
Extracting Element from Java
|
O(1)
|
O(N)
|
Important Features of HashMap
To access a value one must know its key. HashMap is known as HashMap because it uses a technique called Hashing. Hashing is a technique of converting a large String to a small String that represents the same String. A shorter value helps in indexing and faster searches. HashSet also uses HashMap internally.
A few important features of HashMap are:Â
- HashMap is a part of java.util package.
- HashMap extends an abstract class AbstractMap which also provides an incomplete implementation of the Map interface.
- It also implements a Cloneable and Serializable interfaces. K and V in the above definition represent Key and Value respectively.
- HashMap doesn’t allow duplicate keys but allows duplicate values. That means A single key can’t contain more than 1 value but more than 1 key can contain a single value.
- HashMap allows a null key also but only once and multiple null values.
- This class makes no guarantees as to the order of the map; in particular, it does not guarantee that the order will remain constant over time. It is roughly similar to HashTable but is unsynchronized.
Internal Structure of HashMap
Internally HashMap contains an array of Node and a node is represented as a class that contains 4 fields:Â
- int hash
- K key
- V value
- Node next
It can be seen that the node is containing a reference to its own object. So it’s a linked list.Â
HashMap:Â
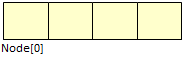
Node:Â
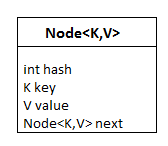
The performance of HashMap depends on 2 parameters which are named as follows:
- Initial Capacity
- Load Factor
1. Initial Capacity – It is the capacity of HashMap at the time of its creation (It is the number of buckets a HashMap can hold when the HashMap is instantiated). In java, it is 2^4=16 initially, meaning it can hold 16 key-value pairs.
2. Load Factor – It is the percent value of the capacity after which the capacity of Hashmap is to be increased (It is the percentage fill of buckets after which Rehashing takes place). In java, it is 0.75f by default, meaning the rehashing takes place after filling 75% of the capacity.
3. Threshold – It is the product of Load Factor and Initial Capacity. In java, by default, it is (16 * 0.75 = 12). That is, Rehashing takes place after inserting 12 key-value pairs into the HashMap.
4. Rehashing – It is the process of doubling the capacity of the HashMap after it reaches its Threshold. In java, HashMap continues to rehash(by default) in the following sequence – 2^4, 2^5, 2^6, 2^7, …. so on.Â
If the initial capacity is kept higher then rehashing will never be done. But by keeping it higher increases the time complexity of iteration. So it should be chosen very cleverly to increase performance. The expected number of values should be taken into account to set the initial capacity. The most generally preferred load factor value is 0.75 which provides a good deal between time and space costs. The load factor’s value varies between 0 and 1.Â
Note: From Java 8 onward, Java has started using Self Balancing BST instead of a linked list for chaining. The advantage of self-balancing bst is, we get the worst case (when every key maps to the same slot) search time is O(Log n).Â
Synchronized HashMap
As it is told that HashMap is unsynchronized i.e. multiple threads can access it simultaneously. If multiple threads access this class simultaneously and at least one thread manipulates it structurally then it is necessary to make it synchronized externally. It is done by synchronizing some object which encapsulates the map. If No such object exists then it can be wrapped around Collections.synchronizedMap() to make HashMap synchronized and avoid accidental unsynchronized access. As in the following example:Â
Map m = Collections.synchronizedMap(new HashMap(...));
Now the Map m is synchronized. Iterators of this class are fail-fast if any structure modification is done after the creation of the iterator, in any way except through the iterator’s remove method. In a failure of an iterator, it will throw ConcurrentModificationException.
Â
Applications of HashMap:Â
HashMap is mainly the implementation of hashing. It is useful when we need efficient implementation of search, insert and delete operations. Please refer to the applications of hashing for details.
Methods in HashMapassociate
- K – The type of the keys in the map.
- V – The type of values mapped in the map.
clear() |
Removes all of the mappings from this map. |
clone() |
Returns a shallow copy of this HashMap instance: the keys and values themselves are not cloned. |
compute(K key, BiFunction<? super K, ? super V,? extends V> remappingFunction) |
Attempts to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) |
If the specified key is not already associated with a value (or is mapped to null), attempts to compute its value using the given mapping function and enters it into this map unless null. |
computeIfPresent(K key, BiFunction<? super K, ? super V,? extends V> remappingFunction) |
If the value for the specified key is present and non-null, attempts to compute a new mapping given the key and its current mapped value. |
containsKey(Object key) |
Returns true if this map contains a mapping for the specified key. |
containsValue(Object value) |
Returns true if this map maps one or more keys to the specified value. |
entrySet() |
Returns a Set view of the mappings contained in this map. |
get(Object key) |
Returns the value to which the specified key is mapped, or null if this map contains no mapping for the key. |
isEmpty() |
Returns true if this map contains no key-value mappings. |
keySet() |
Returns a Set view of the keys contained in this map. |
merge(K key, V value, BiFunction<? super V, ? super V,? extends V> remappingFunction) |
If the specified key is not already associated with a value or is associated with null, associate it with the given non-null value. |
put(K key, V value) |
Associates the specified value with the specified key in this map. |
putAll(Map<? extends K,? extends V> m) |
Copies all of the mappings from the specified map to this map. |
remove(Object key) |
Removes the mapping for the specified key from this map if present. |
size() |
Returns the number of key-value mappings in this map. |
values() |
Returns a Collection view of the values contained in this map. |
 Methods inherited from class java.util.AbstractMap
equals()
|
Compares the specified object with this map for equality. |
hashCode()
|
Returns the hash code value for this map. |
toString()
|
Returns a string representation of this map. |
Methods inherited from interface java.util.Map
equals() |
Compares the specified object with this map for equality. |
forEach(BiConsumer<? super K, ? super V> action)
|
Performs the given action for each entry in this map until all entries have been processed or the action throws an exception. |
getOrDefault(Object key, V defaultValue) |
Returns the value to which the specified key is mapped, or defaultValue if this map contains no mapping for the key. |
hashCode() |
Returns the hash code value for this map. |
putIfAbsent(K key, V value) |
If the specified key is not already associated with a value (or is mapped to null) associates it with the given value and returns null, else returns the current value. |
remove(Object key, Object value) |
Removes the entry for the specified key only if it is currently mapped to the specified value. |
replace(K key, V value) |
Replaces the entry for the specified key only if it is currently mapped to some value. |
replace(K key, V oldValue, V newValue) |
Replaces the entry for the specified key only if currently mapped to the specified value. |
replaceAll(BiFunction<? super K,? super V,? extends V> function)
|
Replaces each entry’s value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. |
Advantages of Java HashMap
- Fast retrieval: HashMaps provide constant time access to elements, which means that retrieval and insertion of elements is very fast.
- Efficient storage: HashMaps use a hashing function to map keys to indices in an array. This allows for quick lookup of values based on keys, and efficient storage of data.
- Flexibility: HashMaps allow for null keys and values, and can store key-value pairs of any data type.
- Easy to use: HashMaps have a simple interface and can be easily implemented in Java.
- Suitable for large data sets: HashMaps can handle large data sets without slowing down.
Disadvantages of Java HashMap
- Unordered: HashMaps are not ordered, which means that the order in which elements are added to the map is not preserved.
- Not thread-safe: HashMaps are not thread-safe, which means that if multiple threads access the same hashmap simultaneously, it can lead to data inconsistencies.
- Performance can degrade: In some cases, if the hashing function is not properly implemented or if the load factor is too high, the performance of a HashMap can degrade.
- More complex than arrays or lists: HashMaps can be more complex to understand and use than simple arrays or lists, especially for beginners.
- Higher memory usage: Since HashMaps use an underlying array, they can use more memory than other data structures like arrays or lists. This can be a disadvantage if memory usage is a concern.
FAQs on Java HashMap
1. What is a HashMap in Java?
HashMap in Java is the class from the collection framework that can store key-value pairs inside it.
2. Why use HashMap in Java?
HashMap in Java is used for storing key-value pairs where each key is unique.
3. What is the benefit of HashMap?
HashMap is used because of it provides features like:
- Fast retrieval
- Efficient storage
- Flexible to use
- Easy to use
- Suitable for large data sets
Share your thoughts in the comments
Please Login to comment...