Spring Boot – Multiple Databases Connection For MongoDB using One Application
Last Updated :
21 Mar, 2024
In Spring Boot, implementing multiple database connections for MongoDB in a spring application and integrating MongoDB with Spring Boot can allow developers to use both technologies to create powerful and scalable applications.
Key Terminologies
- MongoDB Connection Properties: The MongoDB connection properties can be used to configure multiple MongoDB databases into one project. It can be configured in the application.properties
- MongoDB Connection Factory: It can be used to implement a MongoDB connection factory that dynamically creates the connections to the database.
- MongoTemplate: It is a core component of the Spring Data MongoDB, and it can provide a high-level abstraction for interacting with MongoDB databases in the Spring application.
Example Project
In this project, we can develop a spring application that can save employee-added intern data into the different databases using MongoDB.
Step 1: We can create the spring application using the spring initializer, add the below dependencies, create it, and open the IntelliJ IDEA IDE.
Dependencies:
- Lombok
- Spring Data MongoDB
- Spring Web
- Spring Boot DevTools
- Mongo Java Driver
Once you create the project, the file structure looks like the below image.
File Structure:
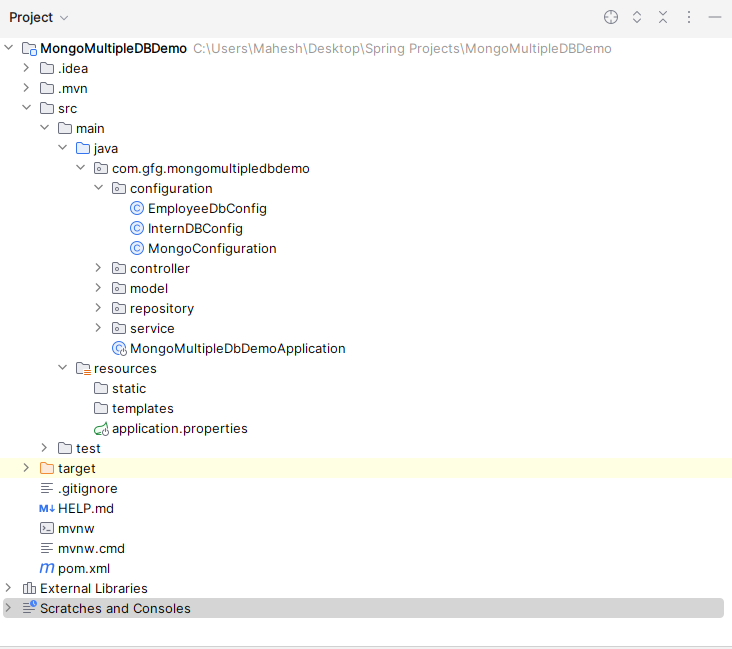
Step 2: Open the application.properties file, and it configures the server port and employee and intern databases.
Go to src > resources > application.properties and put the code below.
server.port=8081
spring.data.mongodb.intern.uri=mongodb://localhost:27017/intern
spring.data.mongodb.employee.uri=mongodb://localhost:27017/employee
spring.autoconfigure.exclude= org.springframework.boot.autoconfigure.mongo.MongoAutoConfiguration
spring.main.allow-bean-definition-overriding=true
Step 3: Open the com.gfg.mongomultipledbdemo package, create the model package, and then create the employee then class.
Go to src > Java> com.gfg.mongomultipledbdemo > model > Employee and put the code below.
Java
package com.gfg.mongomultipledbdemo.model;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "interns_details")
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Employee {
String id;
String employeeName;
String department;
String role;
int salary;
int experience;
}
Step 4: Open the com.gfg.mongomultipledbdemo package and create the Interns class.
Go to src > Java> com.gfg.mongomultipledbdemo > model > Interns and put the code below.
Java
package com.gfg.mongomultipledbdemo.model;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.data.mongodb.core.mapping.Document;
@Document(collection = "employee_details")
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Interns {
private String id;
private String internName;
private String role;
private String department;
private String stipend;
}
Step 5: Open the com.gfg.mongomultipledbdemo package, create the repository package, and create the EmployeeRepository class.
Go to src > Java> com.gfg.mongomultipledbdemo > repository > EmployeeRepository and put the code below.
Java
package com.gfg.mongomultipledbdemo.repository;
import com.gfg.mongomultipledbdemo.model.Employee;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface EmployeeRepository extends MongoRepository<Employee, String> {
}
Step 6: Open the com.gfg.mongomultipledbdemo package and create the InternRepository class.
Go to src > Java> com.gfg.mongomultipledbdemo > repository > InternRepository and put the code below.
Java
package com.gfg.mongomultipledbdemo.repository;
import com.gfg.mongomultipledbdemo.model.Interns;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface InternRepository extends MongoRepository<Interns, String> {
}
Step 7: Open the com.gfg.mongomultipledbdemo package and create the configuration package and the MongoConfiguration class.
Go to src > Java> com.gfg.mongomultipledbdemo > configuration > MongoConfiguration and put the code below.
Java
package com.gfg.mongomultipledbdemo.configuration;
import com.mongodb.MongoClientURI;
import com.mongodb.client.MongoClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.autoconfigure.mongo.MongoProperties;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.data.mongodb.MongoDatabaseFactory;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.SimpleMongoClientDatabaseFactory;
@Configuration
public class MongoConfiguration {
@Primary
@Bean(name = "internDBProperties")
@ConfigurationProperties(prefix = "spring.data.mongodb.intern")
public MongoProperties getInternProps() throws Exception {
return new MongoProperties();
}
@Bean(name = "employeeDBProperties")
@ConfigurationProperties(prefix = "spring.data.mongodb.employee")
public MongoProperties getemployeeProps() throws Exception {
return new MongoProperties();
}
@Primary
@Bean(name = "internMongoTemplate")
public MongoTemplate internsMongoTemplate() throws Exception {
return new MongoTemplate(internMongoDatabaseFactory(getInternProps()));
}
@Bean(name ="employeeMongoTemplate")
public MongoTemplate employeeMongoTemplate() throws Exception {
return new MongoTemplate(employeeMongoDatabaseFactory(getemployeeProps()));
}
@Primary
@Bean
public MongoDatabaseFactory internMongoDatabaseFactory(MongoProperties mongo) throws Exception {
return new SimpleMongoClientDatabaseFactory(
mongo.getUri()
);
}
@Bean
public MongoDatabaseFactory employeeMongoDatabaseFactory(MongoProperties mongo) throws Exception {
return new SimpleMongoClientDatabaseFactory(
mongo.getUri()
);
}
}
Open the com.gfg.mongomultipledbdemo package and create the EmployeeDbConfig class.
Go to src > Java> com.gfg.mongomultipledbdemo > configuration > EmployeeDbConfig and put the code below.
Java
package com.gfg.mongomultipledbdemo.configuration;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.mongodb.repository.config.EnableMongoRepositories;
@Configuration
@EnableMongoRepositories(basePackages = {"com.gfg.mongomultipledbdemo.repository"},
mongoTemplateRef = EmployeeDbConfig.MONGO_TEMPLATE
)
public class EmployeeDbConfig {
protected static final String MONGO_TEMPLATE = "employeeMongoTemplate";
}
Open the com.gfg.mongomultipledbdemo package and create the InternDBConfig class.
Go to src > Java> com.gfg.mongomultipledbdemo > configuration > InternDBConfig and put the code below.
Java
package com.gfg.mongomultipledbdemo.configuration;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.mongodb.repository.config.EnableMongoRepositories;
@Configuration
@EnableMongoRepositories(basePackages = {"com.gfg.mongomultipledbdemo.repository"},
mongoTemplateRef = InternDBConfig.MONGO_TEMPLATE
)
public class InternDBConfig {
protected static final String MONGO_TEMPLATE = "internMongoTemplate";
}
Step 8: Open the com.gfg.mongomultipledbdemo package, create the service package, and then create the EmployeeService class.
Go to src > Java> com.gfg.mongomultipledbdemo > service > EmployeeService and put the code below.
Java
package com.gfg.mongomultipledbdemo.service;
import com.gfg.mongomultipledbdemo.model.Employee;
import com.gfg.mongomultipledbdemo.repository.EmployeeRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
public void saveEmployee(Employee employee) {
employeeRepository.save(employee);
}
}
Open the com.gfg.mongomultipledbdemo package and create the InternService class.
Go to src > Java> com.gfg.mongomultipledbdemo > service > InternService and put the code below.
Java
package com.gfg.mongomultipledbdemo.service;
import com.gfg.mongomultipledbdemo.model.Interns;
import com.gfg.mongomultipledbdemo.repository.InternRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class InternService {
@Autowired
private InternRepository internRepository;
public void saveIntern(Interns intern) {
internRepository.save(intern);
}
}
Step 9: Open the com.gfg.mongomultipledbdemo package and create the controller package and the EmployeeController class.
Go to src > Java> com.gfg.mongomultipledbdemo > controller > EmployeeController and put the code below.
Java
package com.gfg.mongomultipledbdemo.controller;
import com.gfg.mongomultipledbdemo.model.Employee;
import com.gfg.mongomultipledbdemo.repository.EmployeeRepository;
import com.gfg.mongomultipledbdemo.service.EmployeeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/employee")
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
@Autowired
EmployeeRepository employeeRepository;
@PostMapping("/save")
public ResponseEntity<String> saveEmployee(@RequestBody Employee employee) {
employeeService.saveEmployee(employee);
return ResponseEntity.ok("Employee data saved successfully.");
}
}
Open the com.gfg.mongomultipledbdemo package and create the InternController class.
Go to src > Java> com.gfg.mongomultipledbdemo > controller > InternController and put the code below.
Java
package com.gfg.mongomultipledbdemo.controller;
import com.gfg.mongomultipledbdemo.model.Interns;
import com.gfg.mongomultipledbdemo.repository.InternRepository;
import com.gfg.mongomultipledbdemo.service.InternService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/interns")
public class InternController {
@Autowired
private InternService internService;
@Autowired
InternRepository internRepository;
@PostMapping("/save")
public ResponseEntity<String> saveIntern(@RequestBody Interns intern) {
internService.saveIntern(intern);
return ResponseEntity.ok("Intern data saved successfully.");
}
}
Step 10: Open the com.gfg.mongomultipledbdemo package, open the main class, and enter the below code.
Java
package com.gfg.mongomultipledbdemo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MongoMultipleDbDemoApplication {
public static void main(String[] args) {
SpringApplication.run(MongoMultipleDbDemoApplication.class, args);
}
}
pom.xml file:
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.gfg</groupId>
<artifactId>MongoMultipleDBDemo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>MongoMultipleDBDemo</name>
<description>MongoMultipleDBDemo</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-mongodb</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.mongodb/mongo-java-driver -->
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>3.12.14</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Step 11: Once you complete the spring project and run the application, it can start the port number 8081. Please refer to the below image for understanding.

Employee API:
http://localhost:8081/employee/save
Refer the below image
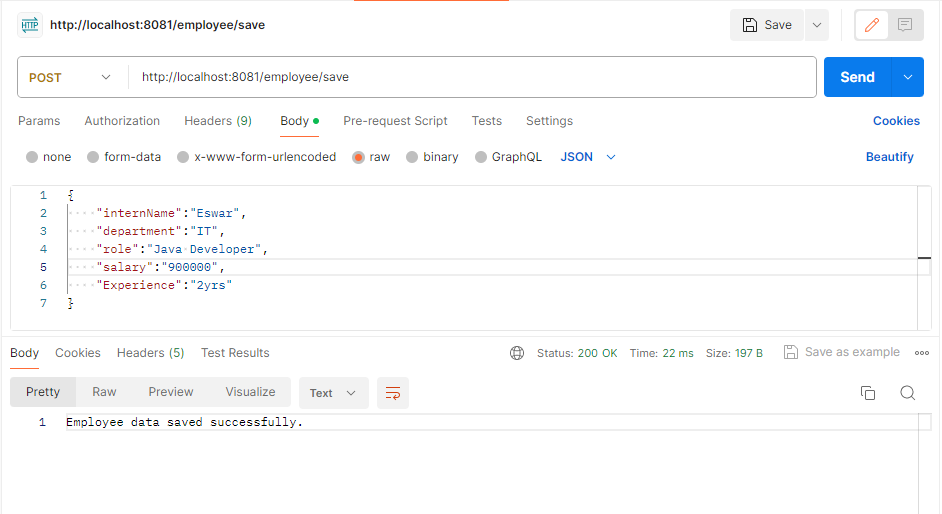
Intern API:
http://localhost:8081/interns/save
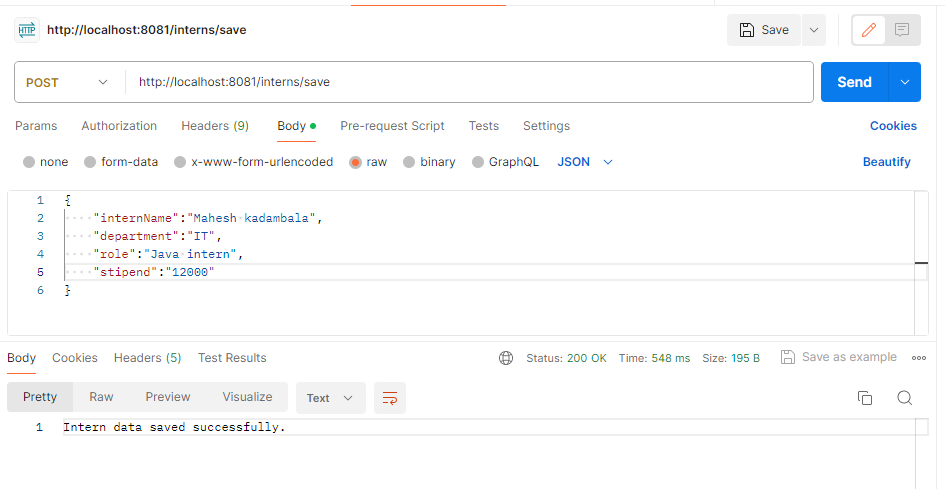
You can follow the above steps, and then you can successfully create a spring application that can be connected to multiple databases in the MongoDB database.
Share your thoughts in the comments
Please Login to comment...