Sports Score Tracker with NodeJS and ExpressJS
Last Updated :
18 Apr, 2024
In sports, real-time updates and scores are very important for fans so that they can stay engaged and informed. In this tutorial, we’ll explore how to build a Sports Score Tracker using Node.js and Express.js.
Preview Image:
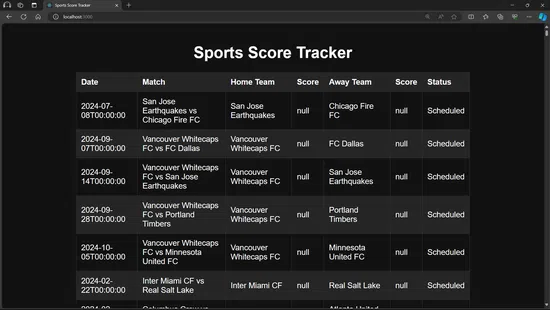
Preview look
Prerequisites
Approach
- Start by setting up a new Node.js project using npm or yarn.
- Initialize a new project directory and install necessary dependencies such as Express.js for the backend server.
- Create the main entry file (e.g., app.js) where you’ll define the Express.js application.
- Integration of Sports API:
- Choose a sports API provider that offers the data you need (e.g., SportsDataIO, ESPN).
- Sign up for an account with the API provider and obtain an API key, which you’ll use to authenticate your requests.
- Implement logic in your backend to make HTTP requests to the API endpoints provided by the sports API.
- Handle the API responses and extract the relevant data (e.g., sports scores, match details).
- Optionally, implement caching mechanisms to reduce the number of requests to the API and improve performance.
- Define Routes and Controllers:
- Define routes in your Express.js application to handle incoming HTTP requests related to fetching sports data.
- Organize your code using controllers to encapsulate the logic for handling each route.
- Define route handlers in the controllers to process incoming requests, interact with the sports API, and send back the appropriate responses.
- Use middleware as needed to add functionality such as error handling, request validation, and authentication.
- Frontend Integration (Optional):
- Optionally, create a frontend interface (e.g., HTML/CSS/JavaScript) to display the sports data fetched from the backend.
- Use JavaScript to make HTTP requests to the backend routes defined in Express.js.
- Dynamically update the frontend UI with the data received from the backend, providing a user-friendly interface for viewing sports scores, match details, and other relevant information.
- Implement features such as live updates using WebSockets or polling, search functionality, and pagination to enhance the user experience.
Steps to create the Project
Step 1: Create a folder for the application using the following command.
mkdir sports-score-tracker
cd sports-score-tracker
Step 2: Initialize the Node application.
npm init -y
Step 3: Install the required dependencies.
npm install express axios
Step 4: Integrating a Sports API
To fetch sports data, we’ll use a third-party API. There are several APIs available, such as the SportsDataIO API or the ESPN API. For this example, let’s assume we’re using the SportsDataIO API. You’ll need to sign up for an API key on their website and then integrate it into your application.
Project Structure:
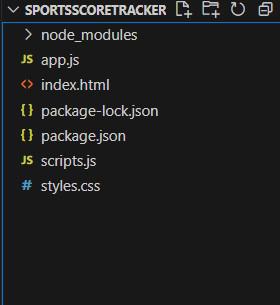
File Structure
Dependencies:
"dependencies": {
"axios": "^1.6.8",
"express": "^4.19.2"
}
Example: Create the required files as shown in the folder structure and add the following codes.
HTML
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sports Score Tracker</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Sports Score Tracker</h1>
<table id="scoreTable">
<thead>
<tr>
<th>Date</th>
<th>Match</th>
<th>Home Team</th>
<th>Score</th>
<th>Away Team</th>
<th>Score</th>
<th>Status</th>
</tr>
</thead>
<tbody id="scoreBody">
</tbody>
</table>
</div>
<script src="scripts.js"></script>
</body>
</html>
CSS
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
h1 {
text-align: center;
}
table {
width: 100%;
border-collapse: collapse;
margin-top: 20px;
}
th,
td {
padding: 10px;
border: 1px solid #ddd;
text-align: left;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
JavaScript
// app.js
const express = require("express");
const path = require("path");
const axios = require("axios");
const app = express();
const PORT = process.env.PORT || 3000;
const API_KEY = "8bec9510e7784559bab7ff96bf4b749d";
app.use(express.static(__dirname));
app.get("/", (req, res) => {
res.sendFile(path.join(__dirname, "index.html"));
});
app.get("/scores", async (req, res) => {
try {
const response = await axios.get(
"https://replay.sportsdata.io/api/v4/soccer/scores/json/schedulesbasic/mls/2024?key=8bec9510e7784559bab7ff96bf4b749d",
{
headers: {
"Ocp-Apim-Subscription-Key": API_KEY,
},
}
);
const scores = response.data;
res.json(scores);
} catch (error) {
console.error("Error fetching scores:", error);
res.status(500).json({ error: "Failed to fetch scores" });
}
});
// Start server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
JavaScript
// scripts.js
document.addEventListener('DOMContentLoaded', async () => {
try {
const response = await fetch('/scores');
if (!response.ok) {
throw new Error('Failed to fetch scores');
}
const matches = await response.json();
const scoreBody = document.getElementById('scoreBody');
matches.forEach(match => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${match.Day}</td>
<td>${match.HomeTeamName} vs ${match.AwayTeamName}</td>
<td>${match.HomeTeamName}</td>
<td>${match.HomeTeamScore}</td>
<td>${match.AwayTeamName}</td>
<td>${match.AwayTeamScore}</td>
<td>${match.Status}</td>
`;
scoreBody.appendChild(row);
});
} catch (error) {
console.error('Error fetching scores:', error);
}
});
Please note that you’ll need to replace placeholders like YOUR_API_KEY with actual values from your chosen sports API provider.
Steps to run the application: You can now start your server by typing the following command in the terminal.
node app.js
Output:
Share your thoughts in the comments
Please Login to comment...