Slice Composite Literal in Go
Last Updated :
02 Mar, 2023
There are two terms i.e. Slice and Composite Literal. Slice is a composite data type similar to an array which is used to hold the elements of the same data type. The main difference between array and slice is that slice can vary in size dynamically but not an array. Composite literals are used to construct the values for arrays, structs, slices, and maps. Each time they are evaluated, it will create new value. They consist of the type of the literal followed by a brace-bound list of elements. (Did you get this point!) Well, after this read you will get to know what is a composite literal and you will be shocked that you know it already !!!! Let’s see how to create a slice and make the use of composite literal:
Go
package main
import "fmt"
func main() {
s1 := []int{ 23 , 56 , 89 , 34 }
fmt.Println(s1)
}
|
Output:
[23 56 89 34]
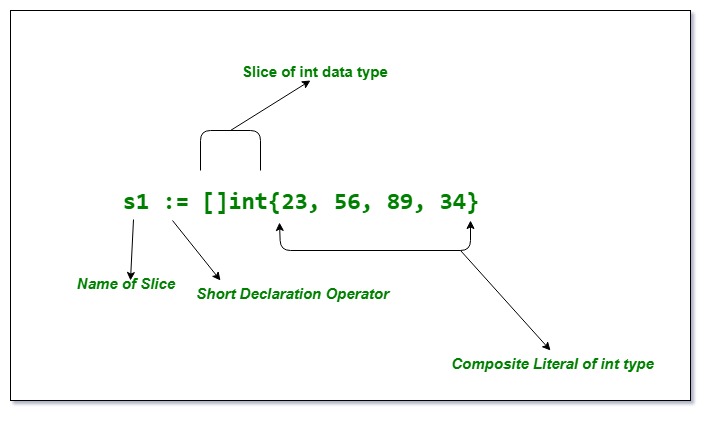
Hope you understand the term what exactly a composite literally. So basically assigning the values or initialization of arrays, slice, etc. are done using composite literals. These are generally used to compose a bunch of values of similar types.
A slice composite literal in Go is a shorthand syntax for creating a slice by specifying its elements directly. A slice composite literal is written as []T{e1, e2, …, ek} where T is the type of the elements in the slice and e1, e2, …, ek are the elements in the slice.
Here’s an example that demonstrates how to create a slice composite literal in Go:
Go
package main
import "fmt"
func main() {
slice := []int{ 1 , 2 , 3 , 4 , 5 }
fmt.Println( "Slice: " , slice)
}
|
Output:
Slice: [1 2 3 4 5]
In this example, the slice composite literal []int{1, 2, 3, 4, 5} creates a slice with the elements 1, 2, 3, 4, 5. The type of the elements in the slice is int, so the type of the slice is []int.
A slice composite literal can also be used to create slices of other types, such as string, float64, or custom types. The syntax is the same, and the elements in the slice must have the same type.
Here’s an example that demonstrates how to create a slice composite literal of type string in Go:
Go
package main
import "fmt"
func main() {
slice := [] string { "apple" , "banana" , "cherry" }
fmt.Println( "Slice: " , slice)
}
|
Output:
Slice: [apple banana cherry]
In this example, the slice composite literal []string{“apple”, “banana”, “cherry”} creates a slice with the elements “apple”, “banana”, “cherry”. The type of the elements in the slice is string, so the type of the slice is []string.
Slice composite literals provide a concise and convenient way to create slices in Go. They are widely used in Go programs, and they can make your code more readable and concise.
Share your thoughts in the comments
Please Login to comment...