Self Organizing List : Move to Front Method
Last Updated :
04 Dec, 2023
Self Organizing list is a list that re-organizes or re-arranges itself for better performance. In a simple list, an item to be searched is looked for in a sequential manner which gives the time complexity of O(n). But in real scenario not all the items are searched frequently and most of the time only few items are searched multiple times.Â
So, a self organizing list uses this property (also known as locality of reference) that brings the most frequent used items at the head of the list. This increases the probability of finding the item at the start of the list and those elements which are rarely used are pushed to the back of the list.Â
In Move to Front Method, the recently searched item is moved to the front of the list. So, this method is quite easy to implement but it also moves in-frequent searched items to front. This moving of in-frequent searched items to the front is a big disadvantage of this method because it affects the access time.Â
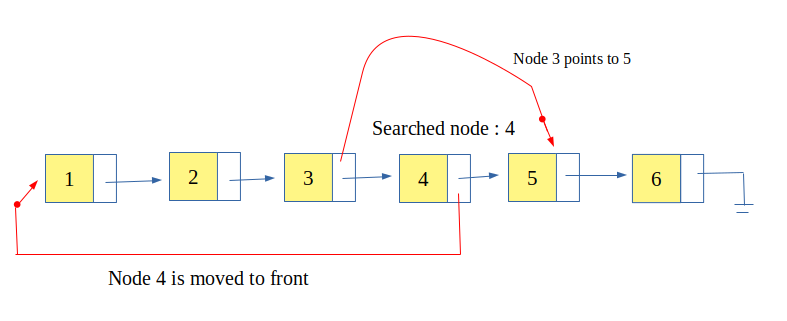
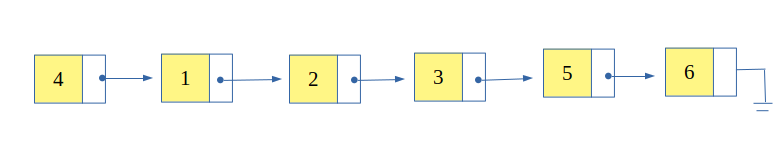
 Examples:
Input : list : 1, 2, 3, 4, 5, 6
searched: 4
Output : list : 4, 1, 2, 3, 5, 6
Input : list : 4, 1, 2, 3, 5, 6
searched : 2
Output : list : 2, 4, 1, 3, 5, 6
Implementation:
CPP
#include <iostream>
using namespace std;
struct self_list {
int value;
struct self_list* next;
};
self_list *head = NULL, *rear = NULL;
void insert_self_list( int number)
{
self_list* temp = (self_list*) malloc ( sizeof (self_list));
temp->value = number;
temp->next = NULL;
if (head == NULL)
head = rear = temp;
else {
rear->next = temp;
rear = temp;
}
}
bool search_self_list( int key)
{
self_list* current = head;
self_list* prev = NULL;
while (current != NULL) {
if (current->value == key) {
if (prev != NULL) {
prev->next = current->next;
current->next = head;
head = current;
}
return true ;
}
prev = current;
current = current->next;
}
return false ;
}
void display()
{
if (head == NULL) {
cout << "List is empty" << endl;
return ;
}
self_list* temp = head;
cout << "List: " ;
while (temp != NULL) {
cout << temp->value;
if (temp->next != NULL)
cout << " --> " ;
temp = temp->next;
}
cout << endl << endl;
}
int main()
{
insert_self_list(1);
insert_self_list(2);
insert_self_list(3);
insert_self_list(4);
insert_self_list(5);
display();
if (search_self_list(4))
cout << "Searched: 4" << endl;
else
cout << "Not Found: 4" << endl;
display();
if (search_self_list(2))
cout << "Searched: 2" << endl;
else
cout << "Not Found: 2" << endl;
display();
return 0;
}
|
Java
public class SelfOrganizingList {
static class Node {
int value;
Node next;
}
static Node head = null ;
static Node rear = null ;
static void insertSelfList( int number)
{
Node temp = new Node();
temp.value = number;
temp.next = null ;
if (head == null ) {
head = rear = temp;
}
else {
rear.next = temp;
rear = temp;
}
}
static boolean searchSelfList( int key)
{
Node current = head;
Node prev = null ;
while (current != null ) {
if (current.value == key) {
if (prev != null ) {
prev.next = current.next;
current.next = head;
head = current;
}
return true ;
}
prev = current;
current = current.next;
}
return false ;
}
static void display()
{
if (head == null ) {
System.out.println( "List is empty" );
return ;
}
Node temp = head;
System.out.print( "List: " );
while (temp != null ) {
System.out.print(temp.value);
if (temp.next != null ) {
System.out.print( " --> " );
}
temp = temp.next;
}
System.out.println( "\n" );
}
public static void main(String[] args)
{
insertSelfList( 1 );
insertSelfList( 2 );
insertSelfList( 3 );
insertSelfList( 4 );
insertSelfList( 5 );
display();
if (searchSelfList( 4 )) {
System.out.println( "Searched: 4" );
}
else {
System.out.println( "Not Found: 4" );
}
display();
if (searchSelfList( 2 )) {
System.out.println( "Searched: 2" );
}
else {
System.out.println( "Not Found: 2" );
}
display();
}
}
|
Python3
class self_list:
def __init__( self , value = None , next = None ):
self .value = value
self . next = next
head = None
rear = None
def insert_self_list(number):
global head, rear
temp = self_list(number)
if head is None :
head = rear = temp
else :
rear. next = temp
rear = temp
def search_self_list(key):
global head
current = head
prev = None
while current is not None :
if current.value = = key:
if prev is not None :
prev. next = current. next
current. next = head
head = current
return True
prev = current
current = current. next
return False
def display():
global head
if head is None :
print ( "List is empty" )
return
temp = head
print ( "List: " , end = "")
while temp is not None :
print (temp.value, end = "")
if temp. next is not None :
print ( " --> " , end = "")
temp = temp. next
print ( "\n" )
if __name__ = = "__main__" :
insert_self_list( 1 )
insert_self_list( 2 )
insert_self_list( 3 )
insert_self_list( 4 )
insert_self_list( 5 )
display()
if search_self_list( 4 ):
print ( "Searched: 4" )
else :
print ( "Not Found: 4" )
display()
if search_self_list( 2 ):
print ( "Searched: 2" )
else :
print ( "Not Found: 2" )
display()
|
C#
using System;
public class SelfOrganizingList
{
public class Node
{
public int value;
public Node next;
}
static Node head = null ;
static Node rear = null ;
static void InsertSelfList( int number)
{
Node temp = new Node();
temp.value = number;
temp.next = null ;
if (head == null )
{
head = rear = temp;
}
else
{
rear.next = temp;
rear = temp;
}
}
static bool SearchSelfList( int key)
{
Node current = head;
Node prev = null ;
while (current != null )
{
if (current.value == key)
{
if (prev != null )
{
prev.next = current.next;
current.next = head;
head = current;
}
return true ;
}
prev = current;
current = current.next;
}
return false ;
}
static void Display()
{
if (head == null )
{
Console.WriteLine( "List is empty" );
return ;
}
Node temp = head;
Console.Write( "List: " );
while (temp != null )
{
Console.Write(temp.value);
if (temp.next != null )
{
Console.Write( " --> " );
}
temp = temp.next;
}
Console.WriteLine( "\n" );
}
public static void Main( string [] args)
{
InsertSelfList(1);
InsertSelfList(2);
InsertSelfList(3);
InsertSelfList(4);
InsertSelfList(5);
Display();
if (SearchSelfList(4))
{
Console.WriteLine( "Searched: 4" );
}
else
{
Console.WriteLine( "Not Found: 4" );
}
Display();
if (SearchSelfList(2))
{
Console.WriteLine( "Searched: 2" );
}
else
{
Console.WriteLine( "Not Found: 2" );
}
Display();
}
}
|
Javascript
class SelfListNode {
constructor(value) {
this .value = value;
this .next = null ;
}
}
let head = null ;
let rear = null ;
function insertSelfList(number) {
const newNode = new SelfListNode(number);
if (head === null ) {
head = rear = newNode;
} else {
rear.next = newNode;
rear = newNode;
}
}
function searchSelfList(key) {
let current = head;
let prev = null ;
while (current !== null ) {
if (current.value === key) {
if (prev !== null ) {
prev.next = current.next;
current.next = head;
head = current;
}
return true ;
}
prev = current;
current = current.next;
}
return false ;
}
function display() {
if (head === null ) {
console.log( "List is empty" );
return ;
}
let temp = head;
let result = "List: " ;
while (temp !== null ) {
result += temp.value;
if (temp.next !== null )
result += " --> " ;
temp = temp.next;
}
console.log(result + "\n" );
}
( function main() {
insertSelfList(1);
insertSelfList(2);
insertSelfList(3);
insertSelfList(4);
insertSelfList(5);
display();
if (searchSelfList(4))
console.log( "Searched: 4\n" );
else
console.log( "Not Found: 4\n" );
display();
if (searchSelfList(2))
console.log( "Searched: 2\n" );
else
console.log( "Not Found: 2\n" );
display();
})();
|
Output
List: 1 --> 2 --> 3 --> 4 --> 5
Searched: 4
List: 4 --> 1 --> 2 --> 3 --> 5
Searched: 2
List: 2 --> 4 --> 1 --> 3 --> 5
Share your thoughts in the comments
Please Login to comment...