RSelenium – Search by name
Last Updated :
18 Mar, 2022
In the article, we are going to learn how we can search an element using the name locator in Rselenium. To be more specific, we are going to learn how to use the findElement(using = ‘name’, value = ‘the name of the element’) method in Rselenium.
Syntax:
rsDriver$findElement(using = ‘name’, value = ‘the name of the element’)
Example:
Consider the following HTML code:
HTML
< html >
< body >
< form >
< input type = "text" name = "firstname" value = "Enter Your firstname" >
< input type = "text" name = "lastname" value = "Enter Your lastname" >
< input type = "submit" value = "Submit" >
</ form >
</ body >
</ html >
|
Output:

Now, if we want to automate the filling of this form. We can use the findElement by name locator. We can use the following code to fill out the form:
Code:
R
rsDriver$ findElement (using = 'name' , value = 'firstname' ) .sendKeys ( 'Ram' )
rsDriver$ findElement (using = 'name' , value = 'lastname' ) .sendKeys ( 'Singh' )
|
Now, let’s try to implement it with the help of Rselenium in R.
Step-by-step Implementation
Step 1: open up the Rstudio and create a new script named searchByName.R
Step 2: Import and load the Rselenium package into the Rstudio by using the following command:
R
install.packages ( "Rselenium" )
library (RSelenium)
|
Step 3: create a new Rselenium server using the Chrome web driver.
R
rdriver <- rsDriver (browser = "chrome" ,
port = 8090L,
chromever = "98.0.4758.102" ,
)
|
This will create a new Rselenium server and will start the Chrome web driver.
Step 4: Create a client object of the Rselenium server to interact with the web browser by using the following command:
R
rseleniumClientObj <- rsDriver$client
|
Step 5: Navigate to the URL [https://www.google.com/] using the following command:
Step 6: To find the element by the name locator [q] and send the search keyword, we can use the following command:
R
searchUsingName <- rseleniumClientObj$ findElement (using = 'name' , value = 'q' )
searchUsingName$ sendKeys ( 'GeeksforGeeks' ,key = "enter" )
|
Here, q is the name of the element in the Google search page. The searchUsingName with sendKeys method will send the search keyword [“GeeksforGeeks”] to the element and then press the enter key.
Step 7: To close the browser and server, run the following command:
R
rseleniumClientObj$ close ()
|
The above piece of code in R will close the Chrome web browser and the Rselenium server.
Full implementation of the whole R program code :
R
library (Rselenium)
rdriver <- rsDriver (browser = "chrome" ,
port = 2020L,
chromever = "98.0.4758.102" ,
)
rseleniumClientObj <- rdriver$client
searchUsingName <- rseleniumClientObj$ findElement (using = 'name' ,
value = 'q' )
searchUsingName$ sendKeysToElement ( list ( "GeeksforGeeks" , key = "enter" ))
rseleniumClientObj$ close ()
|
Output:
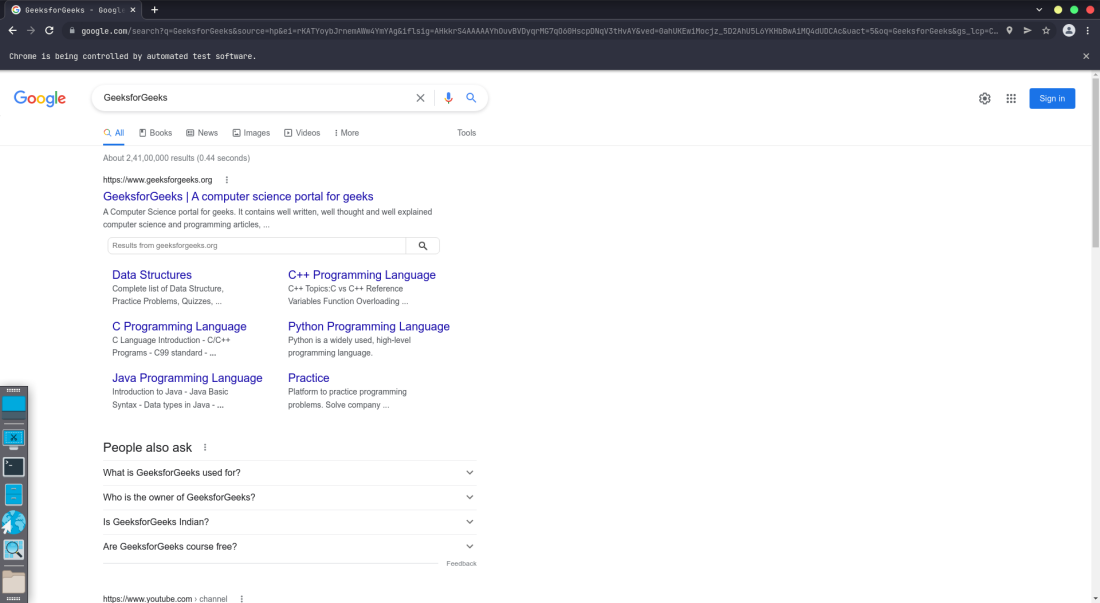
Share your thoughts in the comments
Please Login to comment...