Resolvers in GraphQL
Last Updated :
05 Mar, 2024
Resolvers are a crucial part of GraphQL that determines how data is fetched and returned in response to a query. They act as the bridge between the client’s request and the data source, whether it’s a database, API, or any other data store. Resolvers are responsible for fetching the data for each field in a query and transforming it into the format expected by the client. In this article, we will learn about Resolvers along with an understanding of How Resolvers Work with examples and so on.
What are GraphQL Resolvers?
GraphQL resolvers are defined inside a GraphQL Schema and are responsible for resolving the fields that are represented in a given query. Resolvers have to fetch the data and transform it into the required format before sending it to the client. Resolvers can return scalar values like strings or numbers, as well as complex types like objects or arrays, depending on the schema definition. They can be synchronous or asynchronous, allowing for complex data-fetching operations. They can also interact with 3rd party APIs or external databases, depending on the use case and requirements of the product.
How to Resolvers Work?
- A client makes a GraphQL query request and sends it to the GraphQL server. Each field in the query corresponds to a separate resolver function defined in the GraphQL server.
- The resolvers are responsible for fetching the data, from the database or some other services, for all the fields that are requested in the query.
- Once the data is retrieved, the resolver sends back the response in the same format as requested by the client
- A resolver accepts 4 arguments:
- Parent: It represents the data that is returned by the parent’s resolver field, if we have nested resolvers present inside the query.
- Arguments: These represent the additional arguments that is passed to the query by the user.
- Context: It represents a shared object that is present across the resolvers that get called during a single query operation.
- Info: It represents the data that is present during the query operation, and represents the state of the query, like the field or the path to the field for which the resolver is getting resolved.
Resolver Anatomy
Let’s find out how a resolver looks when implemented.
Example
In the below example, we will create a resolver inside a Query object and we will name it `getUser`. The resolver takes in 4 arguments, as mentioned above. Inside the resolver, we write the logic to fetch the data from the database, and return that data in the required format. For the sake of this example, we will return dummy data to the client.
Javascript
const resolvers = {
Query: {
getUser(parent, args, context, info) {
return {
"id" : "1" ,
"name: " GFG:
}
}
}
};
|
Using Resolvers in Queries
In this step, we will create our own resolver for queries in a GraphQL API.
Step 1: Creating a Node.js Server
We will create a basic node.js server, and then later, we will create a Schema resolver.
First, run the below command to initialise an npm project
npm init -y
Now, install the dependencies for creating an Apollo Server using the below command –
npm i apollo-server
Now, create a new file called server.js, where we will write the logic of creating a GraphQL server, and we will also add GraphQL resolver in the same file. The final project structure should look like below
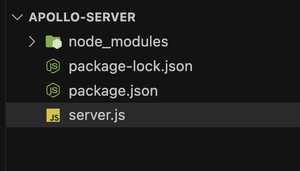
Step 2: Defining Schema Type
In this step, we will create a GraphQL Schema that will have a type Animal, with the properties of id, and name. We will also define a resolver inside it to get an animal by an ID.
Javascript
const typeDefs = gql`
type Animal {
id: ID!
name: String!
}
type Query {
getAnimal(id: ID!): Animal
}
`;
|
Step 3: Creating a Resolver to Serve the Requests
In this step, we will create a GraphQL resolver to serve the requests that are hit to the GraphQL endpoint. We will create a resolver for the `getAnimal`, and return a dummy data for any ID passed in the resolver argument.
Javascript
const resolvers = {
Query: {
getAnimal: (_, { id }) => {
return {
id: '1' ,
name: 'Lion'
}
},
},
};
|
Step 4: Creating and Starting the Apollo Server
In this step, we will create and start our Apollo Server on port 4000.
Javascript
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server running at ${url}`);
});
|
Step 5: Integrating the Above Code Together in a Single File
We will put the whole code above into a single file, and name the file “server.js”. The file would look like below –
Filename: server.js
Javascript
const { ApolloServer, gql } = require( 'apollo-server' );
const typeDefs = gql`
type Animal {
id: ID!
name: String!
}
type Query {
getAnimal(id: ID!): Animal
}
`;
const resolvers = {
Query: {
getAnimal: (_, { id }) => {
return {
id: '1' ,
name: 'Lion'
}
},
},
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server running at ${url}`);
});
|
Let’s start our server by running the below command –
node server.js
You will see the output of the server running –
Server running at http://localhost:4000/
Now, open the graphQL playground here, and execute the below query to fetch the data on the basis of provided schema
query {
getAnimal(id: "1") {
id
name
}
}
Output:
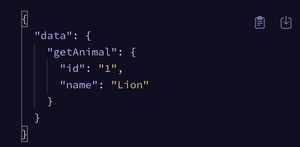
Conclusion
Overall, GraphQL resolvers are essential components that bridge the gap between client queries and data sources. They fetch and transform data as per the query, enabling flexible and efficient data retrieval. Resolvers can interact with various data sources, making them versatile for different use cases. Understanding resolver anatomy and how they work is crucial for building robust GraphQL APIs.
Share your thoughts in the comments
Please Login to comment...