Repeated Character Whose First Appearance is Leftmost
Last Updated :
24 Aug, 2023
Given a string, find the repeated character present first in the string.
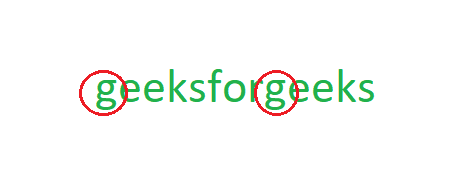
Examples:
Input: geeksforgeeks
Output: g
Input: abcdabcd
Output: a
Input: abcd
Output: -1
Brute Force Approach:
The brute force approach to solve this problem is to consider each character in the string and then check whether it appears again in the remaining part of the string. If it does, then we have found the first repeating character.
Steps that were to follow the above approach:
- Start by iterating through each character in the string.
- For each character, iterate through the remaining characters in the string to check if there is a repeating character.
- If a repeating character is found, return the index of the first occurrence of that character.
- If no repeating character is found, return -1 to indicate that all characters in the string are distinct.
Below is the code to implement the above steps:
C++
#include <bits/stdc++.h>
using namespace std;
int firstRepeating(string& str)
{
int n = str.length();
for ( int i = 0; i < n; i++) {
for ( int j = i + 1; j < n; j++) {
if (str[i] == str[j]) {
return i;
}
}
}
return -1;
}
int main()
{
string str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == -1)
printf ( "Either all characters are "
"distinct or string is empty" );
else
printf ( "First Repeating character"
" is %c" ,
str[index]);
return 0;
}
|
Java
import java.util.*;
public class Main {
public static int firstRepeating(String str) {
int n = str.length();
for ( int i = 0 ; i < n; i++) {
for ( int j = i + 1 ; j < n; j++) {
if (str.charAt(i) == str.charAt(j)) {
return i;
}
}
}
return - 1 ;
}
public static void main(String[] args) {
String str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == - 1 )
System.out.println( "Either all characters are distinct or string is empty" );
else
System.out.println( "First Repeating character is " + str.charAt(index));
}
}
|
Python3
def first_repeating( str ):
n = len ( str )
for i in range (n):
for j in range (i + 1 , n):
if str [i] = = str [j]:
return i
return - 1
str = "geeksforgeeks"
index = first_repeating( str )
if index = = - 1 :
print ( "Either all characters are distinct or string is empty" )
else :
print (f "First Repeating character is {str[index]}" )
|
C#
using System;
class Program
{
static int FirstRepeating( string str)
{
int n = str.Length;
for ( int i = 0; i < n; i++)
{
for ( int j = i + 1; j < n; j++)
{
if (str[i] == str[j])
{
return i;
}
}
}
return -1;
}
static void Main( string [] args)
{
string str = "geeksforgeeks" ;
int index = FirstRepeating(str);
if (index == -1)
{
Console.WriteLine( "Either all characters are distinct or string is empty" );
}
else
{
Console.WriteLine( "First Repeating character is " + str[index]);
}
}
}
|
Javascript
function firstRepeating(str) {
let n = str.length;
for (let i = 0; i < n; i++) {
for (let j = i + 1; j < n; j++) {
if (str[i] === str[j]) {
return i;
}
}
}
return -1;
}
let str = "geeksforgeeks" ;
let index = firstRepeating(str);
if (index === -1)
console.log( "Either all characters are distinct or string is empty" );
else
console.log( "First Repeating character is " + str[index]);
|
Output
First Repeating character is g
Time Complexity: O(N^2)
Auxiliary Space: O(1)
Repeated Character Whose First Appearance is Leftmost by Tracking First Occurrence:
The idea is to keep track of first occurrence of every character and whenever a character repeats check whether it is first repeated character or not.
Follow the steps to solve the problem:
- Initialise an array firstIndex of size 256 which keeps track of the first occurrence of every character in the string.
- Traverse string from left to right and If a character repeats, compare its leftmost index with the current result
- Update the result if the result is greater.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
#define NO_OF_CHARS 256
int firstRepeating(string& str)
{
int firstIndex[NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
firstIndex[i] = -1;
int res = INT_MAX;
for ( int i = 0; i < str.length(); i++) {
if (firstIndex[str[i]] == -1)
firstIndex[str[i]] = i;
else
res = min(res, firstIndex[str[i]]);
}
return (res == INT_MAX) ? -1 : res;
}
int main()
{
string str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == -1)
printf ( "Either all characters are "
"distinct or string is empty" );
else
printf ( "First Repeating character"
" is %c" ,
str[index]);
return 0;
}
|
Java
class GFG
{
static int NO_OF_CHARS = 256 ;
static int firstRepeating( char [] str)
{
int [] firstIndex = new int [NO_OF_CHARS];
for ( int i = 0 ; i < NO_OF_CHARS; i++)
{
firstIndex[i] = - 1 ;
}
int res = Integer.MAX_VALUE;
for ( int i = 0 ; i < str.length; i++)
{
if (firstIndex[str[i]] == - 1 )
{
firstIndex[str[i]] = i;
}
else
{
res = Math.min(res, firstIndex[str[i]]);
}
}
return (res == Integer.MAX_VALUE) ? - 1 : res;
}
public static void main(String[] args)
{
char [] str = "geeksforgeeks" .toCharArray();
int index = firstRepeating(str);
if (index == - 1 )
{
System.out.printf( "Either all characters are "
+ "distinct or string is empty" );
}
else
{
System.out.printf( "First Repeating character"
+ " is %c" , str[index]);
}
}
}
|
Python3
import sys
NO_OF_CHARS = 256
def firstRepeating( str ):
firstIndex = [ 0 for i in range (NO_OF_CHARS)]
for i in range (NO_OF_CHARS):
firstIndex[i] = - 1
res = sys.maxsize
for i in range ( len ( str )):
if (firstIndex[ ord ( str [i])] = = - 1 ):
firstIndex[ ord ( str [i])] = i
else :
res = min (res, firstIndex[ ord ( str [i])])
if res = = sys.maxsize:
return - 1
else :
return res
if __name__ = = '__main__' :
str = "geeksforgeeks"
index = firstRepeating( str )
if (index = = - 1 ):
print ( "Either all characters are distinct or string is empty" )
else :
print ( "First Repeating character is" , str [index])
|
C#
using System;
using System.Collections.Generic;
class GFG
{
static int NO_OF_CHARS = 256;
static int firstRepeating( char [] str)
{
int [] firstIndex = new int [NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
{
firstIndex[i] = -1;
}
int res = int .MaxValue;
for ( int i = 0; i < str.Length; i++)
{
if (firstIndex[str[i]] == -1)
{
firstIndex[str[i]] = i;
}
else
{
res = Math.Min(res, firstIndex[str[i]]);
}
}
return (res == int .MaxValue) ? -1 : res;
}
public static void Main(String[] args)
{
char [] str = "geeksforgeeks" .ToCharArray();
int index = firstRepeating(str);
if (index == -1)
{
Console.Write( "Either all characters are "
+ "distinct or string is empty" );
}
else
{
Console.Write( "First Repeating character"
+ " is {0}" , str[index]);
}
}
}
|
Javascript
<script>
let NO_OF_CHARS = 256;
function firstRepeating(str)
{
let firstIndex = new Array(NO_OF_CHARS);
for (let i = 0; i < NO_OF_CHARS; i++)
{
firstIndex[i] = -1;
}
let res = Number.MAX_VALUE;
for (let i = 0; i < str.length; i++)
{
if (firstIndex[str[i].charCodeAt()] == -1)
{
firstIndex[str[i].charCodeAt()] = i;
}
else
{
res = Math.min(res, firstIndex[str[i].charCodeAt()]);
}
}
return (res == Number.MAX_VALUE) ? -1 : res;
}
let str = "geeksforgeeks" .split( '' );
let index = firstRepeating(str);
if (index == -1)
{
document.write( "Either all characters are "
+ "distinct or string is empty" );
}
else
{
document.write( "First Repeating character is " + str[index]);
}
</script>
|
Output
First Repeating character is g
Time Complexity: O(N). Traversing string one time
Auxiliary Space: O(1)
Repeated Character Whose First Appearance is Leftmost by Reverse Traversal:
The idea is to track the characters which have encountered while traversing from right to left. Whenever a character is already repeated then take that index to our answer.
Follow the steps to solve the problem:
- Initialise an array visited of size 256 which keeps track of characters that have been encountered.
- Traverse string from right to left and If a character repeats, add that index to the result.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
#define NO_OF_CHARS 256
int firstRepeating(string& str)
{
bool visited[NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
visited[i] = false ;
int res = -1;
for ( int i = str.length() - 1; i >= 0; i--) {
if (visited[str[i]] == false )
visited[str[i]] = true ;
else
res = i;
}
return res;
}
int main()
{
string str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == -1)
printf ( "Either all characters are "
"distinct or string is empty" );
else
printf ( "First Repeating character"
" is %c" ,
str[index]);
return 0;
}
|
Java
import java.util.*;
class GFG
{
static int NO_OF_CHARS= 256 ;
static int firstRepeating(String str)
{
boolean []visited = new boolean [NO_OF_CHARS];
for ( int i = 0 ; i < NO_OF_CHARS; i++)
visited[i] = false ;
int res = - 1 ;
for ( int i = str.length() - 1 ; i >= 0 ; i--)
{
if (visited[str.charAt(i)] == false )
visited[str.charAt(i)] = true ;
else
res = i;
}
return res;
}
public static void main(String[] args)
{
String str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == - 1 )
System.out.printf( "Either all characters are "
+ "distinct or string is empty" );
else
System.out.printf( "First Repeating character"
+ " is %c" ,
str.charAt(index));
}
}
|
Python3
NO_OF_CHARS = 256
def firstRepeating(string) :
visited = [ False ] * NO_OF_CHARS;
for i in range (NO_OF_CHARS) :
visited[i] = False ;
res = - 1 ;
for i in range ( len (string) - 1 , - 1 , - 1 ) :
if (visited[string.index(string[i])] = = False ):
visited[string.index(string[i])] = True ;
else :
res = i;
return res;
if __name__ = = "__main__" :
string = "geeksforgeeks" ;
index = firstRepeating(string);
if (index = = - 1 ) :
print ( "Either all characters are" ,
"distinct or string is empty" );
else :
print ( "First Repeating character is:" , string[index]);
|
C#
using System;
class GFG
{
static int NO_OF_CHARS = 256;
static int firstRepeating(String str)
{
Boolean []visited = new Boolean[NO_OF_CHARS];
for ( int i = 0; i < NO_OF_CHARS; i++)
visited[i] = false ;
int res = -1;
for ( int i = str.Length - 1; i >= 0; i--)
{
if (visited[str[i]] == false )
visited[str[i]] = true ;
else
res = i;
}
return res;
}
public static void Main(String[] args)
{
String str = "geeksforgeeks" ;
int index = firstRepeating(str);
if (index == -1)
Console.Write( "Either all characters are " +
"distinct or string is empty" );
else
Console.Write( "First Repeating character" +
" is {0}" , str[index]);
}
}
|
Javascript
<script>
let NO_OF_CHARS = 256;
function firstRepeating(str)
{
let visited = new Array(NO_OF_CHARS);
for (let i = 0; i < NO_OF_CHARS; i++)
visited[i] = false ;
let res = -1;
for (let i = str.length - 1; i >= 0; i--)
{
if (visited[str[i].charCodeAt()] == false )
visited[str[i].charCodeAt()] = true ;
else
res = i;
}
return res;
}
let str = "geeksforgeeks" ;
let index = firstRepeating(str);
if (index == -1)
document.write( "Either all characters are " +
"distinct or string is empty" );
else
document.write( "First Repeating character" +
" is " + str[index]);
</script>
|
Output
First Repeating character is g
Time Complexity: O(N). Traversing string one time
Auxiliary Space: O(1)
Discussed more approaches in Find repeated character present first in a string.
Share your thoughts in the comments
Please Login to comment...