Real-Time Translation App Using Python
Last Updated :
28 Dec, 2023
In this article, we’ll delve into the creation of a potent real-time translation application using Python. Leveraging the capabilities of the Google Translate API, we’ll walk you through building an intuitive graphical user interface (GUI) with the Tkinter library. With just a few lines of code, you can craft a user-friendly application facilitating instant translation across multiple languages. Whether you’re a novice or a seasoned developer, this tutorial offers a hands-on approach to implementing an efficient language translation tool in Python. Join us on the journey of enhancing cross-language communication with our Real-Time Translation App.
The day-to-day challenge of communication across language barriers can be overcome through the power of Python and Tkinter. This article explores the development of a simple yet effective real-time translator application using only Python and Tkinter. The application takes user input in one language and seamlessly translates it into the selected language, breaking down language obstacles and facilitating smoother communication.
Real-Time Translation App Using Python
Using python we create the Real-Time Translation App with the help of which we can translate any lnagage to any language following language by some simple clicks for creating the Real-Time Translation App Using Python following language the below steps
Step 1: Install Necessary Libraries
Install the ‘translate’ and ‘googletrans’ libraries, where ‘translate’ serves as the translation engine, and ‘googletrans’ provides language information. To install, run these commands in your terminal/command prompt
pip install translate
pip install googletrans
Once these libraries are installed, you’ve met the prerequisites for building the application.
Step 1: Import Necessary Libraries
Here, that three lines import the necessary modules for creating a GUI in Python using Tkinter. `ttk` is used for themed widgets, and `LANGUAGES` from the `googletrans` library provides a dictionary of supported languages.
Python3
from tkinter import *
from tkinter import ttk
from googletrans import LANGUAGES
|
Step 2: Setting up the Tkinter Window
Here , the code create a Tkinter window (`root`) with dimensions 1100×320, set it to be non-resizable, give it a pink background, and assign the title ‘Real-time translator’.
Python3
root = Tk()
root.geometry( '1100x320' )
root.resizable( 0 , 0 )
root[ 'bg' ] = 'pink'
root.title( 'Real-time translator' )
|
Step 3: Creating GUI
Below code sets up a Tkinter-based GUI for a language translation app. It includes labels for the title, input, and output sections, an entry widget for user input, a text widget for displaying translations, and a combobox for selecting the destination language. Specific coordinates are defined for proper placement on the window. This forms the user interface of the app by creating labels, entry widgets, text widgets, and a dropdown menu for language selection.
Python3
Label(root, text = "Language Translator" , font = "Arial 20 bold" ).pack()
Label(root, text = "Enter Text" , font = 'arial 13 bold' , bg = 'white smoke' ).place(x = 165 , y = 90 )
Input_text = Entry(root, width = 60 )
Input_text.place(x = 30 , y = 130 )
Label(root, text = "Output" , font = 'arial 13 bold' , bg = 'white smoke' ).place(x = 780 , y = 90 )
Output_text = Text(root, font = 'arial 10' , height = 5 , wrap = WORD, padx = 5 , pady = 5 , width = 50 )
Output_text.place(x = 600 , y = 130 )
language = list (LANGUAGES.values())
dest_lang = ttk.Combobox(root, values = language, width = 22 )
dest_lang.place(x = 130 , y = 180 )
dest_lang. set ( 'Choose Language' )
|
Step 4: Translation Function
Here , the `Translate` function uses the `googletrans` library to translate text from an entry widget (`Input_text`) to a specified destination language. It clears the output text widget (`Output_text`) before inserting the translated text. In case of any errors during translation, an exception is caught and an error message is printed to the console. Also, error handling is included to catch and print any exceptions that may occur during translation.
Python3
def Translate():
try :
translator = Translator(to_lang = dest_lang.get())
translation = translator.translate(Input_text.get())
Output_text.delete( 1.0 , END)
Output_text.insert(END, translation)
except Exception as e:
print (f "Translation error: {e}" )
|
Step 5: Button For Triggering Translation
here, the code creates a Tkinter button for translation with the label ‘Translate’, styled in Arial font. It triggers the `Translate` function on click, with an orange background and green active background, positioned at (445, 180) in the window.
Python3
trans_btn = Button(root, text = 'Translate' , font = 'arial 12 bold' , pady = 5 , command = Translate, bg = 'orange' , activebackground = 'green' )
trans_btn.place(x = 445 , y = 180 )
|
Step 6: Run Tkinter main loop
This simply initiates the Tkinter main loop, allowing the GUI to run and respond to user interactions.
Complete Code
Python3
from tkinter import *
from tkinter import ttk
from googletrans import Translator, LANGUAGES
root = Tk()
root.geometry( '1100x320' )
root.resizable( 0 , 0 )
root[ 'bg' ] = 'pink'
root.title( 'Real-time translator' )
Label(root, text = "Language Translator" , font = "Arial 20 bold" ).pack()
Label(root, text = "Enter Text" , font = 'arial 13 bold' , bg = 'white smoke' ).place(x = 165 , y = 90 )
Input_text = Entry(root, width = 60 )
Input_text.place(x = 30 , y = 130 )
Label(root, text = "Output" , font = 'arial 13 bold' , bg = 'white smoke' ).place(x = 780 , y = 90 )
Output_text = Text(root, font = 'arial 10' , height = 5 , wrap = WORD, padx = 5 , pady = 5 , width = 50 )
Output_text.place(x = 600 , y = 130 )
language = list (LANGUAGES.values())
dest_lang = ttk.Combobox(root, values = language, width = 22 )
dest_lang.place(x = 130 , y = 180 )
dest_lang. set ( 'Choose Language' )
def Translate():
try :
translator = Translator()
translation = translator.translate(Input_text.get(), dest = dest_lang.get())
Output_text.delete( 1.0 , END)
Output_text.insert(END, translation.text)
except Exception as e:
print (f "Translation error: {e}" )
trans_btn = Button(root, text = 'Translate' , font = 'arial 12 bold' , pady = 5 , command = Translate, bg = 'orange' , activebackground = 'green' )
trans_btn.place(x = 445 , y = 180 )
root.mainloop()
|
Output :
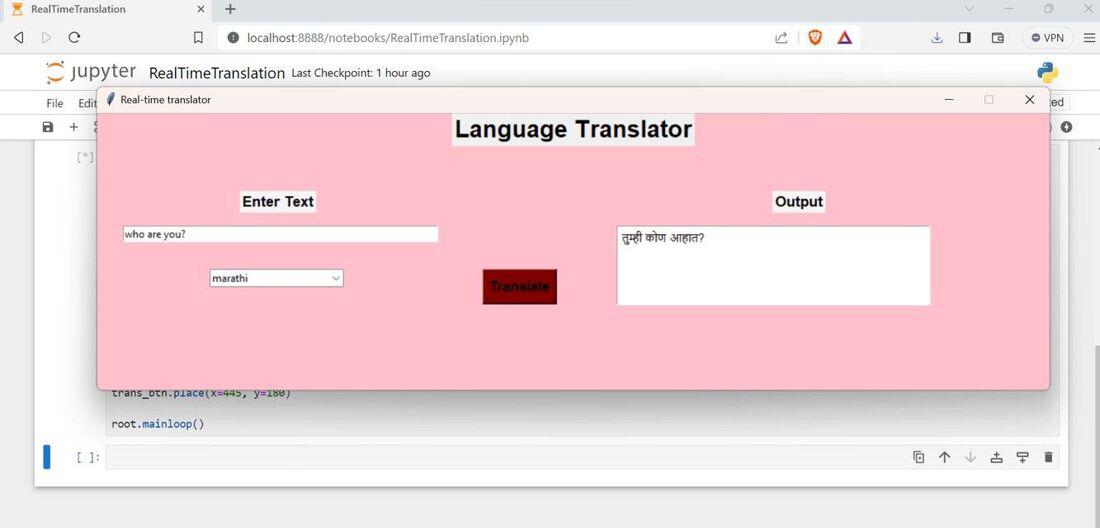
Conclusion
In this article, we delved into the creation of a real-time translator app using Python and Tkinter. The application addresses language barriers in daily communication, enabling users to input text and receive instant translations in their preferred language. Leveraging the simplicity of Tkinter and the translation capabilities of the ‘translate’ library, users can explore creative possibilities in designing diverse language-related tools. Feel free to unleash your creativity in GUI design and uncover the intriguing potential that Python and Tkinter offer in language-related applications.
Share your thoughts in the comments
Please Login to comment...