How to Read and Write Files Using the New I/O (NIO.2) API in Java?
Last Updated :
18 Mar, 2024
In this article, we will learn how to read and write files using the new I/O (NIO) API in Java. For this first, we need to import the file from the NIO package in Java. This NIO.2 is introduced from the Java 7 version. This provides a more efficient way of handling input and output operations compared to the traditional Java package java.io. Now, by using java.nio package we can write and read a file.
Below we have provided two examples for writing a file and reading a file.
Write files Using NIO
For writing information into files, we have one class that is Files which is used for handling file operations in NIO. This class is available in java.nio. Then take the information and write it into an example file. Here we have taken an example.txt file for writing and reading operations.
Java Program to Write Files using the New I/O (NIO.2) API
Below is the Program to write files using the New I/O (Nio.2) API:
Java
// Java Program to Write Files using the New I/O (NIO.2) API
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
public class FileWriteExample {
public static void main(String[] args) {
Path filePath = Paths.get("example.txt");
try {
// Create a list of strings to be written to the file
List<String> lines = new ArrayList<>();
lines.add("Welcome");
lines.add("to");
lines.add("GeeksForGeeks");
// Write the lines to the file
Files.write(filePath, lines);
System.out.println("File written successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
OutputFile written successfully.
Explanation of the Program:
- First, we have created a Java Class.
- After that, we get path information about the target file by using Path class available in java.nio package.
- Here our target file is example.txt.
- Next in try catch block, we have taken some String values as a list because the write method takes byte array only.
- Then perform write operation by using Files.write().
Read files using NIO
In this example, we can read existing data from the example.txt using Files.readAllLines(). This method can be able to read all data from the file. This method takes one argument that file path.
Java
// Java Program to Read Files using the New I/O (NIO.2) API
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.List;
public class File1 {
public static void main(String[] args) {
// Define the path to the file
Path filePath = Paths.get("example.txt");
try {
// Read all lines from the file into a list
List<String> lines = Files.readAllLines(filePath);
// Print each line
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
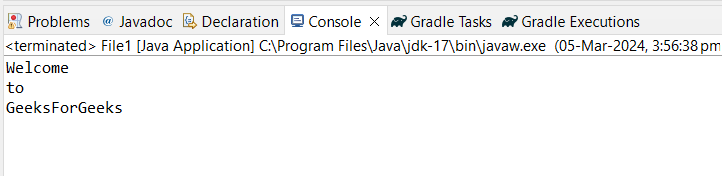
Explanation of the Program:
- First, we have created a Java class.
- After that, we get path information about the target file by using Path class available in java.nio package.
- Here our target file example.txt
- Next in try catch block, we read all data from file by using Files.readAllLines(filePath).
- After that by using for loop, it prints each and every string in the file.
Share your thoughts in the comments
Please Login to comment...