How to Read and Write XML Files in Java?
Last Updated :
02 Feb, 2024
XML is defined as the Extensible Markup Language, and it is mostly used as a format for storing and exchanging data between systems. To read and write XML files, Java programming offers several easily implementable libraries. The most widely used library is the built-in JAXP (Java API for XML processing).
What is DOM?
The Document Object Model (DOM) is defined as a programming interface for web documents. In the context of XML processing in Java, DOM represents XML document as a tree model, where each node in the tree model corresponds to a part of the document. They are used to work with and navigate this tree using methods of the DOM API.
Requirements to work with XML:
- Install the JDK (Java Development Kit) installed in your system.
- Once installed the code into your choice either VSCode, Eclipse, or InteljIdea wherever based on your choice code editor.
- We need to work with XML file processing and must import the javax.xml package. This package contains a parser and transforms pre-defined classes to work with XML files.
The Java API for XML Processing (JAXMP) provides a set of interfaces and classes for processing XML documents in Java programming. Here are some interfaces and classes.
- DocumentBuilder: DocumentBuilder is a pre-defined interface in the JAXMP library that defines the methods for parsing XML documents and creating a DOM (Document Object Model) tree.
- DocumentBuilderFactory: This class provides a factory for creating DocumentBuilder objects. It obtains the document builder instance, which is then used to parse XML documents.
- Document: Document is an interface that offers ways to read and modify the content of an XML document while also representing the full document.
- Element: An XML document’s interface that represents an element. Elements are the fundamental units of the XML structure.
- NodeList: An XML document’s ordered collection of nodes represented by an interface that is usually used to traverse over a list of elements.
- Transformer: An XML document transformation interface that specifies how to change the content of a Source tree into a result tree.
- Source: An interface that can represent a stream of XML, a DOM tree, or other sources as the input for a transformation.
- StreamResult: a class that shows the output of a straightforward transformation, usually as a character or byte stream.
Steps-by-Step Implementation
Step 1: Create the DocumentBuilder and Document
In Java Programming, we need to work with XML processing into the program. First, we create the DocumentBuilderFactory is used to accesses a DocumentBuilder instance and it instance is used to create a new Document which represents the XML structure.
// Create a DocumentBuilder
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
// Create a new Document
Document document = builder.newDocument();
Step 2: Create the root element
Once the XML document, we need to create one root element for the XML document then root element adds into the document.
// Create root element
Element root = document.createElement("library");
document.appendChild(root);
Step 3: Create child elements
Create child elements for root element add into the document using createElement and appendElement methods are used to add content into the XML document.
Step 4: Write to XML file
Write the document into the XML file using Transformer to transform the DOM structure into a stream of characters. And using StreamResult specify the output file.
Program to Write XML File in Java
Below is the code implementation to write XML files in Java Programming language.
Java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
public class XMLWriterExample {
public static void main(String[] args) throws Exception {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.newDocument();
Element root = document.createElement( "library" );
document.appendChild(root);
Element book1 = document.createElement( "Program1" );
book1.appendChild(document.createTextNode( "Java Programming" ));
Element book2 = document.createElement( "Program2" );
book2.appendChild(document.createTextNode( "Python Programming" ));
Element book3 = document.createElement( "Program3" );
book3.appendChild(document.createTextNode( "JavaScript" ));
Element book4 = document.createElement( "Program4" );
book4.appendChild(document.createTextNode( "C Programming" ));
root.appendChild(book1);
root.appendChild(book2);
root.appendChild(book3);
root.appendChild(book4);
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
DOMSource source = new DOMSource(document);
StreamResult result = new StreamResult( "C:/Users/Mahesh/Desktop/DSAˍPratice/output.xml" );
transformer.transform(source, result);
System.out.println( "XML file created successfully!" );
}
}
|
Output:
XML file created successfully
Below is the generated XML File:
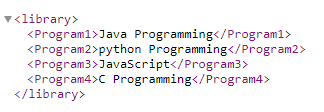
Note: This example processes XML using the integrated JAXP API.
Program to Read XML File in Java
Below are the steps to implement:
- Specify the XML file path as String and give the file location of XML file.
- Create the DocumentBuilder and that can be used to parse the XML file.
- After that accesses the elements using TagName.
- Once the accesses the element using for loop then print the elements into the XML file.
XMLReaderExample.java:
Java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
import org.w3c.dom.Node;
import java.io.File;
public class XMLReaderExample {
public static void main(String[] args) throws Exception {
File xmlFile = new File( "C:/Users/Mahesh/Desktop/DSAˍPratice/output.xml" );
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(xmlFile);
NodeList nodeList = document.getElementsByTagName( "library" );
for ( int i = 0 ; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
System.out.println( "Element Content: " + node.getTextContent());
}
}
}
|
Output:
Element Content: Java Programming python Programming JavaScript C Programming
Share your thoughts in the comments
Please Login to comment...