Read a text file using Python Tkinter
Last Updated :
16 Feb, 2024
Graphical User Interfaces (GUIs) are an essential aspect of modern software development, providing users with interactive and visually appealing applications. Python’s Tkinter library is a robust tool for creating GUIs, and in this article, we will delve into the process of building a Tkinter application that reads and displays the content of a text file.
Read a Text File Using Python Tkinter
Below is the step-by-step procedure by which we can read a text file using Python Tkinter in Python:
Step 1: Installation
We will install the following modules before starting with the program.
Step 2: Import Tkinter
Start by importing the Tkinter module and the `filedialog` submodule.
Python3
import tkinter as tk
from tkinter import filedialog
|
Step 3: Create the main window
Create the main Tkinter window using the `Tk` class.
Python3
root = tk.Tk()
root.title( "Text File Reader" )
|
Step 4: Create a Text widget
Add a `Text` widget to the window. This widget will serve as the area to display the content of the text file.
Python3
text_widget = tk.Text(root, wrap = "word" , width = 40 , height = 10 )
text_widget.pack(pady = 10 )
|
Step 5: Create a button to open the file
Include a button that users can click to open a text file.
Python3
open_button = tk.Button(root, text = "Open File" , command = open_file)
open_button.pack(pady = 10 )
|
Step 6: Define the `open_file` function
Create a function that prompts the user to select a text file and displays its content in the `Text` widget.
Python3
def open_file():
file_path = filedialog.askopenfilename(
title = "Select a Text File" , filetypes = [( "Text files" , "*.txt" )])
if file_path:
with open (file_path, 'r' ) as file :
content = file .read()
text_widget.delete( 1.0 , tk.END)
text_widget.insert(tk.END, content)
|
Step 7: Run the Tkinter event loop
Start the Tkinter event loop to run the GUI application.
Full Code Implementation
In this code, a simple Tkinter GUI application is created to open and display the content of a text file. The program utilizes the `tkinter` library, implements a function (`open_file`) to open a text file through a file dialog, and displays the file’s content in a Tkinter Text widget within the main window.
Python3
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename(
title = "Select a Text File" , filetypes = [( "Text files" , "*.txt" )])
if file_path:
with open (file_path, 'r' ) as file :
content = file .read()
text_widget.delete( 1.0 , tk.END)
text_widget.insert(tk.END, content)
root = tk.Tk()
root.title( "Text File Reader" )
text_widget = tk.Text(root, wrap = "word" , width = 40 , height = 10 )
text_widget.pack(pady = 10 )
open_button = tk.Button(root, text = "Open File" , command = open_file)
open_button.pack(pady = 10 )
root.mainloop()
|
Output:
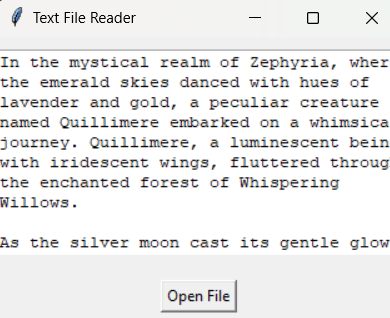
Tkinter window
Conclusion
This article provided a comprehensive guide on using Tkinter to build a text file reader GUI application in Python. By understanding the concepts of Tkinter widgets and file handling, developers can create powerful applications that enhance user interaction and experience. The example and step-by-step guide serve as a foundation for more complex projects involving file interactions within a graphical interface.
Share your thoughts in the comments
Please Login to comment...