How to Read Text File Into List in Python?
Last Updated :
19 Dec, 2021
In this article, we are going to see how to read text files into lists in Python.
File for demonstration:
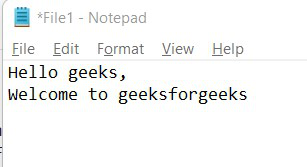
Example 1: Converting a text file into a list by splitting the text on the occurrence of ‘.’.
We open the file in reading mode, then read all the text using the read() and store it into a variable called data. after that we replace the end of the line(‘/n’) with ‘ ‘ and split the text further when ‘.’ is seen using the split() and replace() functions.
read(): The read bytes are returned as a string. Reads n bytes, or the full file if no n is given.
Syntax: fileobject.read(size)
split(): The split() method creates a list from a string. The separator can be specified; the default separator is any whitespace.
Syntax: string.split(separator, maxsplit)
replace(): The replace() method substitutes one phrase for another.
Syntax: string.replace(previous_value, value_to_be_replaced_with, count)
Code:
Python3
my_file = open ( "file1.txt" , "r" )
data = my_file.read()
data_into_list = data.replace( '\n' , ' ' ).split( "." )
print (data_into_list)
my_file.close()
|
Output:
['Hello geeks Welcome to geeksforgeeks']
Example 2: Converting a text file into a list by splitting the text on the occurrence of newline (‘\n’ )
The same process as before but we don’t replace any string here.
Python3
my_file = open ( "file1.txt" , "r" )
data = my_file.read()
data_into_list = data.split( "\n" )
print (data_into_list)
my_file.close()
|
Output:
['Hello geeks', 'Welcome to geeksforgeeks']
Share your thoughts in the comments
Please Login to comment...