React Suite Tooltip Whisper Methods
Last Updated :
06 Jun, 2023
React Suite is a library of UI components for React that offers a variety of customizable and reusable components. One such component is the Tooltip Whisper component. The Tooltip Whisper component is a tooltip that can be used to display information when a user hovers over or clicks on an element. It can be customized to display various types of content such as text, images, and icons. The Tooltip Whisper component can be used in React by importing it from the React Suite library and using it in the JSX code.Â
React Suite Tooltip Whisper Methods:
- open: The open method refers to the process of creating a new whisper session and establishing a private communication channel between two or more users.
- close: The close method refers to the process of ending or terminating an active whisper session, which can be initiated by either the sender or the receiver.
- updatePosition: The updatePosition refers to the ability to change the position of a whisper window on the screen by specifying new coordinates. This can be useful for adjusting the size and location of the whisper window to better fit the user’s preferences and screen layout.
Syntax: Whisper methods are available via ref on the Whisper component.
const whisperRef = useRef();
<Whisper ref={whisperRef} {...}>
...
</Whisper>
Â
Creating React Application And Installing Module:
Step 1: To create a react app, you need to install react modules through the npx command in your current repository.
npx create-react-app ./
Step 2: After creating the React JS application, Install the required module using the following command:
npm install rsuite
Project Structure :
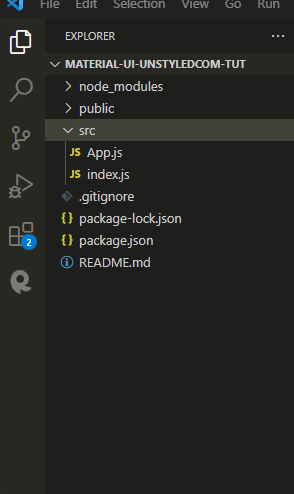
File Structure
Example 1: The Whisper component is a wrapper that takes two child components: the trigger and the speaker. In this example, the Button component is used as the trigger and the Tooltip component is used as the speaker. The ref attribute is used to create a reference to the Whisper component so that it can be manipulated programmatically. The handleClick function is called when the Button is clicked and it calls the open method on the whisperRef object to display the tooltip for 5 seconds.
Filename: App.js
Javascript
import React from 'react' ;
import "rsuite/dist/rsuite.min.css" ;
import { useRef } from 'react' ;
import { Button, Tooltip, Whisper } from 'rsuite' ;
export default function App() {
const whisperRef = useRef( null );
const handleClick = () => {
whisperRef.current.open(5000);
};
return (
<div style={{
display: 'block' , width: 700, paddingLeft: 30
}}>
<h1 style={{ color: 'green' }}>
GeeksForGeeks
</h1>
<h3 style={{ color: 'green' }}>
React Suite Tooltip Whisper methods
</h3>
<Whisper
ref={whisperRef}
trigger= "none"
placement= "right"
speaker={<Tooltip >Required Text</Tooltip>}>
<Button appearance= "subtle"
onClick={handleClick}>
Right
</Button>
</Whisper>
<br />
<hr />
</div>
);
}
|
Step to Run the Application:Â
npm start
Output:
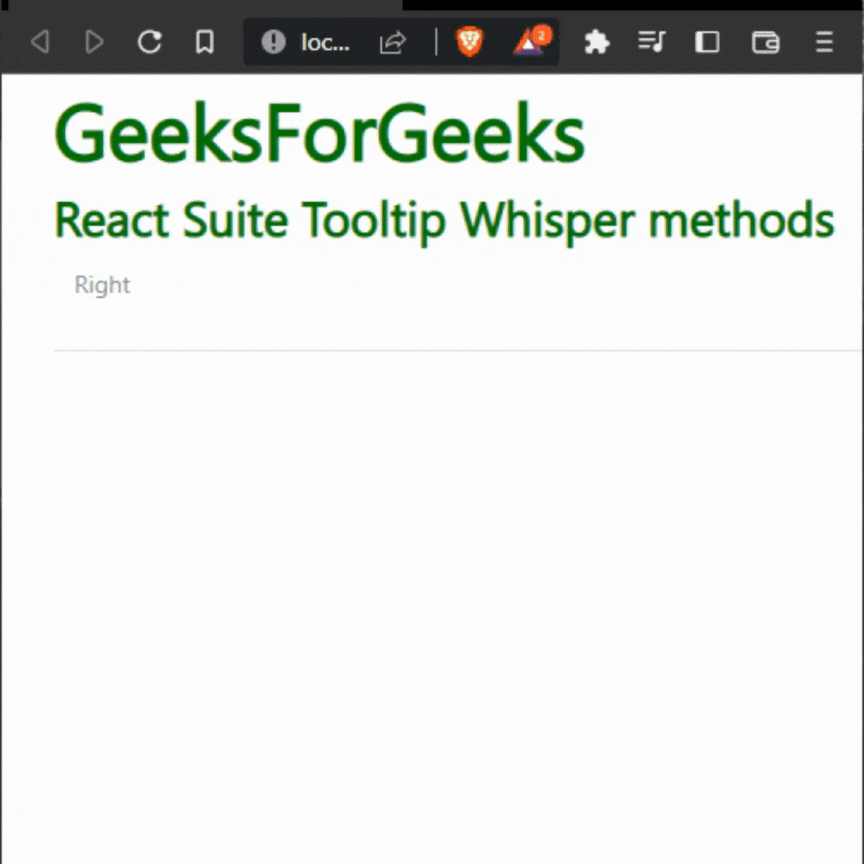
Opens the overlay after a delay of 5 s  Â
Example 2: The Whisper component is a wrapper that takes two child components: the trigger and the speaker. In this example, the Button component is used as the trigger and the Tooltip component is used as the speaker. The ref attribute is used to create a reference to the Whisper component so that it can be manipulated programmatically. The handleClick function is called when the Button is clicked and it calls the close method on the whisperRef object to close the tooltip after 5 seconds.
Filename: App.js
Javascript
import React from 'react' ;
import "rsuite/dist/rsuite.min.css" ;
import { useRef } from 'react' ;
import { Button, Tooltip, Whisper } from 'rsuite' ;
export default function App() {
const whisperRef = useRef( null );
const handleClick = () => {
whisperRef.current.close(5000);
};
return (
<div style={{
display: 'block' , width: 700, paddingLeft: 30
}}>
<h1 style={{ color: 'green' }}>
GeeksForGeeks
</h1>
<h3 style={{ color: 'green' }}>
React Suite Tooltip Whisper methods
</h3>
<Whisper
ref={whisperRef}
trigger= "none"
placement= "right"
speaker={
<Tooltip >Required Text</Tooltip>
}>
<Button appearance= "subtle"
onClick={handleClick}>
Right
</Button>
</Whisper>
<br />
<hr />
</div>
);
}
|
Step to Run the Application:Â
npm start
Output:
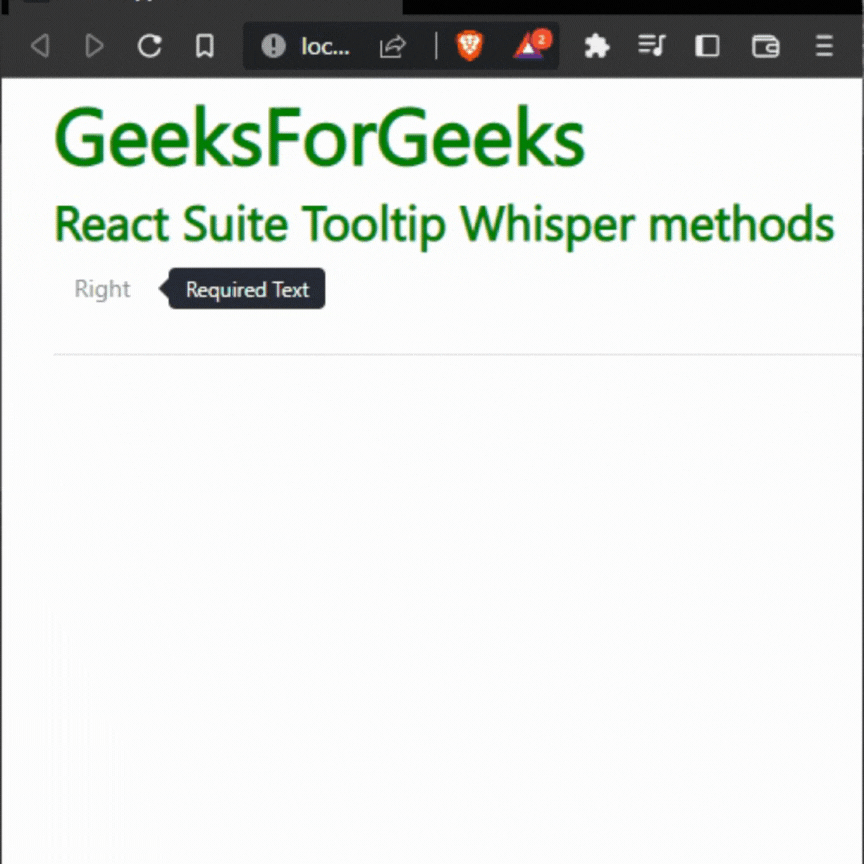
closes the overlay after a delay of 5sÂ
Reference: https://rsuitejs.com/components/whisper/
Share your thoughts in the comments
Please Login to comment...