Python | Math operations for Data analysis
Last Updated :
21 Mar, 2024
Python is a great language for doing data analysis, primarily because of the fantastic ecosystem of data-centric Python packages. Pandas is one of those packages and makes importing and analyzing data much easier.
There are some important math operations that can be performed on a pandas series to simplify data analysis using Python and save a lot of time.
s=read_csv("stock.csv", squeeze=True)
#reading csv file and making series
Function |
Use |
s.sum() |
Returns sum of all values in the series |
s.mean() |
Returns mean of all values in series. Equals to s.sum()/s.count()
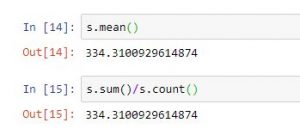
|
s.std() |
Returns standard deviation of all values |
s.min() or s.max() |
Return min and max values from series |
s.idxmin() or s.idxmax() |
Returns index of min or max value in series |
s.median() |
Returns median of all value |
s.mode() |
Returns mode of the series |
s.value_counts() |
Returns series with frequency of each value
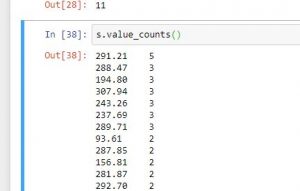
|
s.describe() |
Returns a series with information like mean, mode, etc depending on dtype of data passed
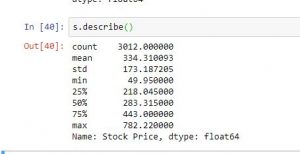
|
Code #1:
Python3
import pandas as pd
s = pd.read_csv( "stock.csv" , squeeze = True )
print (s.count())
print (s. sum ())
print (s.mean())
print (s. sum () / s.count())
print (s.std())
print (s. min ())
print (s. max ())
print (s.median())
print (s.mode())
|
Output:
3012
1006942.0
334.3100929614874
334.3100929614874
173.18720477113115
49.95
782.22
283.315
0 291.21
Code #2:
Python3
import pandas as pd
s = pd.read_csv( "stock.csv" , squeeze = True )
print (s.describe())
print (s.idxmax())
print (s.idxmin())
print (s.value_counts().head( 3 ))
|
Output:
dtype: float64
count 3012.000000
mean 334.310093
std 173.187205
min 49.950000
25% 218.045000
50% 283.315000
75% 443.000000
max 782.220000
Name: Stock Price, dtype: float64
3011
11
291.21 5
288.47 3
194.80 3
Name: Stock Price, dtype: int64
Unexpected Outputs and Restrictions:
- .sum(), .mean(), .mode(), .median() and other such mathematical operations are not applicable on string or any other data type than numeric value.
- .sum() on a string series would give an unexpected output and return a string by concatenating every string.
Share your thoughts in the comments
Please Login to comment...