Program to print double sided Stair-Case Pattern
Last Updated :
03 Jan, 2024
Creating a double-sided staircase pattern is a fun and educational exercise that helps practice programming skills, especially loops and conditional statements. In this article, we will walk through the process of writing a program to print a double-sided stair-case pattern
Examples:
Input: 10
Output:
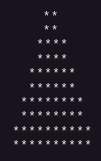
Output for Input: 10
Approach: To solve the problem follow the below idea:
It uses two nested loops: the outer loop iterates from 1 to n, and the inner loops handle space and star printing based on the row number and the condition of odd or even. If a row number is odd, it increments the value of k by 1; otherwise, it keeps it the same. The space and star printing is adjusted accordingly. The output is a staircase-like pattern of stars and spaces.
Below is the implementation of the above approach:
C++
#include <iostream>
int main()
{
int n = 10;
for ( int i = 1; i <= n; ++i) {
int k = (i % 2 != 0) ? i + 1 : i;
for ( int g = 0; g < (n - k); ++g) {
std::cout << " ";
}
for ( int j = 0; j < k; ++j) {
if (j == k - 1) {
std::cout << "* " << std::endl;
}
else {
std::cout << "* ";
}
}
}
return 0;
}
|
Java
public class GFG {
public static void main(String[] args) {
int n = 10 ;
for ( int i = 1 ; i <= n; ++i) {
int k = (i % 2 != 0 ) ? i + 1 : i;
for ( int g = 0 ; g < (n - k); ++g) {
System.out.print( " " );
}
for ( int j = 0 ; j < k; ++j) {
if (j == k - 1 ) {
System.out.print( "*\n" );
} else {
System.out.print( "* " );
}
}
}
}
}
|
Python3
n = 10
for i in range ( 1 , n + 1 ):
k = i + 1 if i % 2 ! = 0 else i
for g in range (n - k):
print ( " " , end = "")
for j in range (k):
if j = = k - 1 :
print ( "* " )
else :
print ( "* " , end = "")
|
C#
using System;
class GFG {
public static void Main ( string [] args) {
int n = 10;
for ( int i = 1; i <= n; ++i) {
int k = (i % 2 != 0) ? i + 1 : i;
for ( int g = 0; g < (n - k); ++g) {
Console.Write ( " " );
}
for ( int j = 0; j < k; ++j) {
if (j == k - 1) {
Console.WriteLine ( "* " );
}
else {
Console.Write ( "* " );
}
}
}
}
}
|
Javascript
function printPattern(n) {
for (let i = 1; i <= n; ++i) {
let k = (i % 2 !== 0) ? i + 1 : i;
for (let g = 0; g < (n - k); ++g) {
process.stdout.write( " " );
}
for (let j = 0; j < k; ++j) {
if (j === k - 1) {
console.log( "*" );
} else {
process.stdout.write( "* " );
}
}
}
}
let n = 10;
printPattern(n);
|
Output
* *
* *
* * * *
* * * *
* * * * * *
* * * * * *
* * * * * * * *
* * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
Time Complexity: O(N2)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...