Program to Count numbers on fingers
Last Updated :
31 Mar, 2023
Count the given numbers on your fingers and find the correct finger on which the number ends.
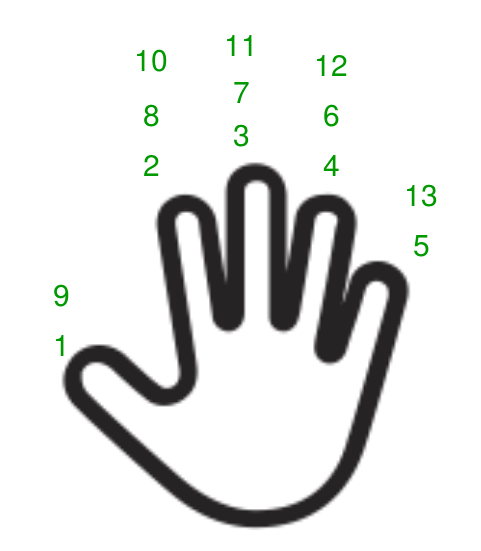
Examples:
Input : 17
Output :1
Input :27
Output :3
Approach: The first number starts from the thumb, second on the index finger, third on the middle finger, fourth on the ring finger, and fifth on the little finger.
- Again six comes on the ring finger and so on.
- Here we observer a pattern, 8(last number) and 2 ends up in 2nd position, 3rd or 7th on the middle finger, and so on.
- The pattern keeps repeating after every 8 numbers
- 1 to 8
- 9 to 16
- 17 to 24, and so on
C++
Java
class GFG
{
static int count_num_finger( int n)
{
int r = n % 8 ;
if (r == 0 )
return 2 ;
if (r < 5 )
return r;
else
return 10 - r;
}
public static void main(String[] args)
{
int n;
n = 30 ;
System.out.println(count_num_finger(n));
}
}
|
Python3
def count_num_finger( n ):
r = n % 8
if r = = 0 :
return 2
if r < 5 :
return r
else :
return 10 - r
n = 30
print (count_num_finger(n))
|
Javascript
<script>
function count_num_finger(n)
{
let r = n % 8;
if (r == 0)
return 2;
if (r < 5)
return r;
else
return 10 - r;
}
let n;
n = 30;
document.write(count_num_finger(n));
</script>
|
C#
PHP
<?php
function count_num_finger( $n )
{
$r = $n % 8;
if ( $r == 2)
return 0;
if ( $r < 5)
return $r ;
else
return 10 - $r ;
}
$n = 30;
echo (count_num_finger( $n ));
?>
|
Time Complexity: O(1), As we are doing only constant time operations.
Auxiliary Space: O(1), As constant extra space is used.
Asked in Paytm Campus Placement August 2017
#Example:2
C++
#include <iostream>
using namespace std;
int countFingers( int n)
{
int full_hands = n / 10;
int full_fingers = full_hands * 10;
int remaining_fingers = n % 10;
int total_fingers = full_fingers + remaining_fingers;
return total_fingers;
}
int main()
{
int n = 23;
int total_fingers = countFingers(n);
cout << "Total number of fingers used to count " << n
<< " is " << total_fingers << endl;
return 0;
}
|
Java
import java.util.*;
public class Gfg {
public static int countFingers( int n) {
int full_hands = n / 10 ;
int full_fingers = full_hands * 10 ;
int remaining_fingers = n % 10 ;
int total_fingers = full_fingers + remaining_fingers;
return total_fingers;
}
public static void main(String[] args) {
int n = 23 ;
int total_fingers = countFingers(n);
System.out.println( "Total number of fingers used to count " + n
+ " is " + total_fingers);
}
}
|
Python3
def count_fingers(n):
full_hands = n / / 10
full_fingers = full_hands * 10
remaining_fingers = n % 10
total_fingers = full_fingers + remaining_fingers
return total_fingers
n = 23
total_fingers = count_fingers(n)
print ( "Total number of fingers used to count" , n, "is" , total_fingers)
|
Javascript
function count_fingers(n) {
let full_hands = Math.floor(n / 10);
let full_fingers = full_hands * 10;
let remaining_fingers = n % 10;
let total_fingers = full_fingers + remaining_fingers;
return total_fingers;
}
let n = 23;
let total_fingers = count_fingers(n);
console.log( 'Total number of fingers used to count ' +n+ ' is' + total_fingers);
|
C#
using System;
class Program {
static int CountFingers( int n) {
int fullHands = n / 10;
int fullFingers = fullHands * 10;
int remainingFingers = n % 10;
int totalFingers = fullFingers + remainingFingers;
return totalFingers;
}
static void Main( string [] args) {
int n = 23;
int totalFingers = CountFingers(n);
Console.WriteLine( "Total number of fingers used to count {0} is {1}" , n, totalFingers);
}
}
|
Output
Total number of fingers used to count 23 is 23
Approach:
1.Define a function count_fingers that takes an integer n as input. The function will return the total number of fingers used to count up to n.
2.Calculate the number of full hands needed to count up to n by dividing n by 10 and using integer division to round down to the nearest whole number. Assign this value to the variable full_hands.
3.Calculate the number of fingers used in the full hands by multiplying full_hands by 10. Assign this value to the variable full_fingers.
4.Calculate the number of remaining fingers needed to count up to n after using all full hands by taking the remainder of n divided by 10. 5.Assign this value to the variable remaining_fingers.
6.Calculate the total number of fingers used to count up to n by adding full_fingers and remaining_fingers. Assign this value to the variable total_fingers.
7.Return the total_fingers value.
8.In the example usage, create an integer n and call the count_fingers function with this argument. Finally, print the total number of fingers used to count up to n.
Time complexity: O(1).
Space complexity: O(1).
Share your thoughts in the comments
Please Login to comment...