Printing string in plus ‘+’ pattern in the matrix
Last Updated :
17 Feb, 2023
Given a string, print it inside a matrix in such a way that a ‘plus’ is formed.
Examples:
Input: TOP
Output:
X T X
T O P
X P X
Input: FEVER
Output:
X X F X X
X X E X X
F E V E R
X X E X X
X X R X X
Approach:
The idea is simple. First we can access every element of the matrix and make it ‘X’. Then we will insert the characters of the string in the middle row as well as in the middle column of the matrix. For example, we have string of length 5. So we will need a (5X5) matrix for it.
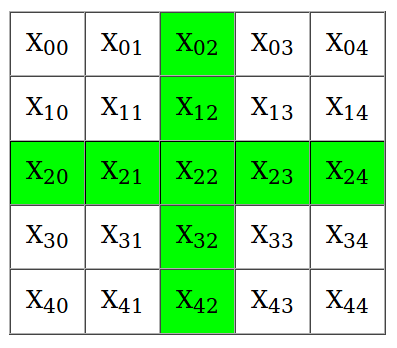
To access the middle column of the matrix, column index is made constant and is equal to (n/2), where n is the length of the string. Row index will go from 0 to (n-1) for it.
To access the middle row, the row index will be made constant and equal to (n/2) and the column index will go from 0 to (n-1).
Below is the implementation of above approach:
C++
#include <bits/stdc++.h>
#define max 100
using namespace std;
void carveCross(string str)
{
int n = str.length();
if (n % 2 == 0)
{
cout << "Not possible. Please enter "
<< "odd length string.\n" ;
}
else {
char arr[max][max];
int m = n / 2;
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < n; j++) {
arr[i][j] = 'X' ;
}
}
for ( int i = 0; i < n; i++)
{
arr[i][m] = str[i];
}
for ( int i = 0; i < n; i++)
{
arr[m][i] = str[i];
}
for ( int i = 0; i < n; i++) {
for ( int j = 0; j < n; j++) {
cout << arr[i][j] << " " ;
}
cout << "\n" ;
}
}
}
int main()
{
string str = "PICTURE" ;
carveCross(str);
return 0;
}
|
Java
Python 3
max = 100
def carveCross( str ):
n = len ( str )
if (n % 2 = = 0 ) :
print ( "Not possible. Please enter "
, "odd length string.\n" )
else :
arr = [[ False for x in range ( max )]
for y in range ( max )]
m = n / / 2
for i in range ( n) :
for j in range (n) :
arr[i][j] = 'X'
for i in range (n):
arr[i][m] = str [i]
for i in range (n):
arr[m][i] = str [i]
for i in range (n):
for j in range (n):
print ( arr[i][j] , end = " " )
print ()
if __name__ = = "__main__" :
str = "PICTURE"
carveCross( str )
|
C#
using System;
class GFG {
static int max = 100;
static void carveCross(String str) {
int n = str.Length;
if (n % 2 == 0) {
Console.Write( "Not possible. Please enter "
+ "odd length string." );
}
else {
char [,]arr = new char [max,max];
int m = n / 2;
for ( int i = 0; i < n; i++)
{
for ( int j = 0; j < n; j++)
{
arr[i,j] = 'X' ;
}
}
for ( int i = 0; i < n; i++) {
arr[i,m] = str[i];
}
for ( int i = 0; i < n; i++) {
arr[m,i] = str[i];
}
for ( int i = 0; i < n; i++)
{
for ( int j = 0; j < n; j++)
{
Console.Write(arr[i,j] + " " );
}
Console.WriteLine();
}
}
}
public static void Main() {
string str = "PICTURE" ;
carveCross(str);
}
}
|
PHP
<?php
function carveCross( $str )
{
$n = strlen ( $str );
if ( $n % 2 == 0)
{
echo ( "Not possible. Please enter " );
echo ( "odd length string.\n" );
}
else
{
$arr = array ();
$m = $n / 2;
for ( $i = 0; $i < $n ; $i ++)
{
for ( $j = 0; $j < $n ; $j ++)
{
$arr [ $i ][ $j ] = 'X' ;
}
}
for ( $i = 0; $i < $n ; $i ++)
{
$arr [ $i ][ $m ] = $str [ $i ];
}
for ( $i = 0; $i < $n ; $i ++)
{
$arr [ $m ][ $i ] = $str [ $i ];
}
for ( $i = 0; $i < $n ; $i ++)
{
for ( $j = 0; $j < $n ; $j ++)
{
echo ( $arr [ $i ][ $j ] . " " );
}
echo ( "\n" );
}
}
}
$str = "PICTURE" ;
carveCross( $str );
?>
|
Javascript
<script>
const max = 100
function carveCross(str)
{
let n = str.length;
if (n % 2 == 0)
{
document.write( "Not possible. Please enter odd length string." , "</br>" );
}
else {
let arr = new Array(max);
for (let i=0;i<max;i++){
arr[i] = new Array(max);
}
let m = Math.floor(n / 2);
for (let i = 0; i < n; i++) {
for (let j = 0; j < n; j++) {
arr[i][j] = 'X' ;
}
}
for (let i = 0; i < n; i++)
{
arr[i][m] = str[i];
}
for (let i = 0; i < n; i++)
{
arr[m][i] = str[i];
}
for (let i = 0; i < n; i++) {
for (let j = 0; j < n; j++) {
document.write(arr[i][j] + " " );
}
document.write( "</br>" );
}
}
}
let str = "PICTURE" ;
carveCross(str);
</script>
|
Output:
X X X P X X X
X X X I X X X
X X X C X X X
P I C T U R E
X X X U X X X
X X X R X X X
X X X E X X X
Time Complexity : O(n2)
Auxiliary Space: O(MAX2) where MAX is a defined constant
Share your thoughts in the comments
Please Login to comment...