POTD Solutions | 26 Oct’ 23 | Minimum Operations
Last Updated :
30 Oct, 2023
Welcome to the daily solutions of our PROBLEM OF THE DAY (POTD). We will discuss the entire problem step-by-step and work towards developing an optimized solution. This will not only help you brush up on your concepts of Mathematics but will also help you build up problem-solving skills.
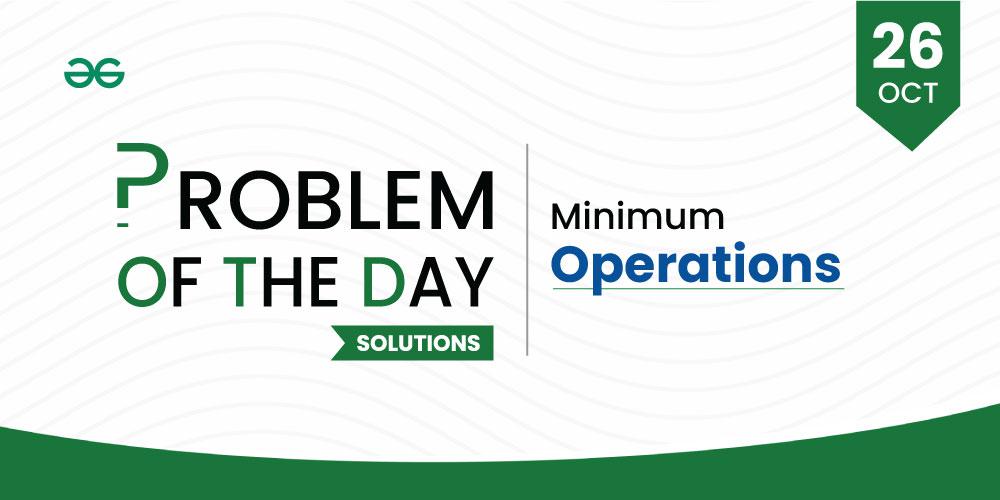
POTD 26 OCT
We recommend you to try this problem on our GeeksforGeeks Practice portal first, and maintain your streak to earn Geeksbits and other exciting prizes, before moving towards the solution.
POTD 26 October: Minimum Operations
Given a number N. Find the minimum number of operations required to reach N starting from 0. You have 2 operations available:
- Double the number
- Add one to the number
Example:
Input: N = 8
Output: 4
Explanation: 0 + 1 = 1 –> 1 + 1 = 2 –> 2 * 2 = 4 –> 4 * 2 = 8.
Input: N = 7
Output: 5
Explanation: 0 + 1 = 1 –> 1 + 1 = 2 –> 1 + 2 = 3 –> 3 * 2 = 6 –> 6 + 1 = 7
Minimum Operations using Mathematics:
The two available operations are doubling the number or adding one to the number. To minimize the number of operations, we will choose between these two operations based on whether the current number is even or odd. If the current number is even we divide the number else we subtract one from the number, as we try to go from n to 0.
Follow the steps to solve the above problem:
- Initialize a variable count equal to 0 to store the minimum number of operations.
- Run a loop while n is not equal to 0.
- If the n is even then divide n by 2.
- Otherwise, subtract 1 from n.
- Increase count by 1.
- Return count.
Below is the implementation of above approach:
C++
class Solution {
public :
int minOperation( int n)
{
int count = 0;
while (n) {
if (n % 2 == 0) {
n = n / 2;
}
else {
n--;
}
count++;
}
return count;
}
};
|
Java
class Solution {
public int minOperation( int n)
{
int count = 0 ;
while (n != 0 ) {
if (n % 2 == 0 ) {
n = n / 2 ;
}
else {
n--;
}
count++;
}
return count;
}
}
|
Python3
class Solution:
def minOperation( self , n):
count = 0
while n:
if n % 2 = = 0 :
n = n / / 2
else :
n - = 1
count + = 1
return count
|
Time Complexity: O(logN), As we divide the number by 2 when we encounter even number thus our complexity becomes O(logN).
Auxiliary Space: O(1), As we are not using any extra space
Share your thoughts in the comments
Please Login to comment...