Parameterize SQL IN Clause
Last Updated :
06 May, 2024
The ‘IN‘ clause in SQL filters query results based on a specified list of values. It retrieves rows where a particular column matches any value within a provided list. Parameterizing the ‘IN’ clause adds flexibility to SQL queries, allowing for dynamic values, enhanced security, and efficient code reuse.
Before delving into the specifics of “Parameterizing an SQL IN clause,” it is essential to have a foundational understanding of SQL query syntax and the ‘IN’ clause itself. Familiarity with SQL queries and database structures is crucial for grasping parameterization concepts.
The ‘IN‘ Operator in SQL
The ‘IN’ operator in SQL selects rows where a specific column matches any value in a provided list. It’s useful for concise filtering and replacing multiple ‘OR‘ conditions. Parameterizing the ‘IN’ clause adds flexibility for dynamic values in queries.
Syntax
SELECT columnName FROM tableName
WHERE columnName IN (value1, value2, value3…);
Here is the syntax of our SQL query with the ‘IN‘ operator and the ‘WHERE‘ clause.
How to Parameterize IN clause in SQL
To Parameterize SQL IN clause means using variables to supply values at runtime. This is very useful when dealing with user inputs or when the list of values is not known beforehand.
To parameterize SQL IN clause, write the SQL statement with placeholders instead of actual values.
Demo SQL Database
Before we start parameterizing the ‘IN’ clause directly, we first need to create a database, the table inside it, and also need to insert the values.
SQL
CREATE DATABASE OnlineStoreDB;
USE OnlineStoreDB;
CREATE TABLE Products (
ProductID INT PRIMARY KEY,
ProductName VARCHAR(255),
Price DECIMAL(10, 2)
);
INSERT INTO Products (ProductID, ProductName, Price) VALUES
(1, 'Product A', 10.99),
(2, 'Product B', 24.50),
(3, 'Product C', 15.75),
(4, 'Product D', 8.99),
(5, 'Product E', 19.99);
Output:
ProductID | ProductName | Price |
---|
1 | Product A | 10.99 |
2 | Product B | 24.5 |
3 | Product C | 15.75 |
4 | Product D | 8.99 |
5 | Product E | 19.99 |
After creating the demo SQL table, let’s perform parameterization on SQL IN clause in the following examples.
Parameterize an SQL IN clause Examples
Let’s understand how to parameterize IN clause in SQL with some examples.
Example 1: Basic Parameterization
We will first set the variable and then select all by using the ‘*’ operator. And, to do that we’ll simply write the following query.
SET @ProductIDs = '1, 3, 5';
SELECT *
FROM Products
WHERE FIND_IN_SET(ProductID, @ProductIDs);
Output:
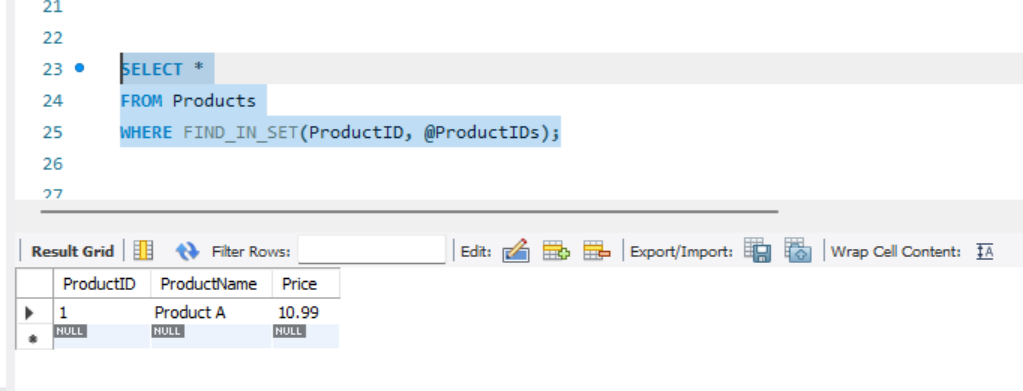
Basic Parameterization
Explanation: In this example, I have used the ‘FIND_IN_SET‘ function to check whether the ‘ProductID‘ is present in the comma-separated list provided by ‘@ProductIDs‘.
Example 2: Using Parameters in Query
SET @ProductIDs = '1, 3, 5';
SELECT *
FROM Products
WHERE ProductID IN (
SELECT CAST(value AS UNSIGNED)
FROM (
SELECT TRIM(SUBSTRING_INDEX(SUBSTRING_INDEX(@ProductIDs, ',', n.digit+1), ',', -1)) AS value
FROM (
SELECT 0 AS digit UNION ALL SELECT 1 UNION ALL SELECT 2 UNION ALL SELECT 3 UNION ALL SELECT 4
) n
WHERE n.digit < LENGTH(@ProductIDs) - LENGTH(REPLACE(@ProductIDs, ',', '')) + 1
) AS split_values
);
Output:
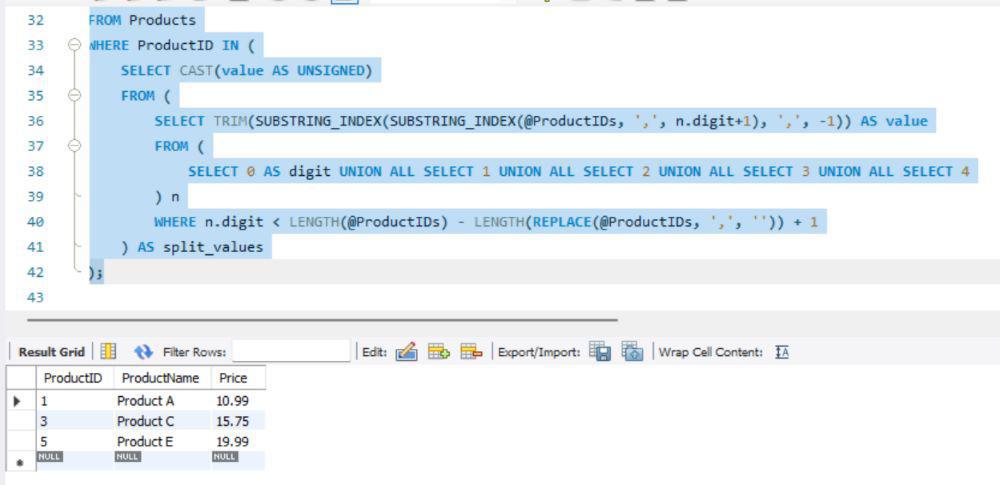
Parameters in Query
Explanation: From this example, we can understand that it utilizes a strong split of the comma-separated values in ‘@ProductIDs‘ using nested ‘SELECT‘ statements.
Example 3: Parameterizing with Price Range
SET @PriceRange = '10.00, 15.00';
SELECT *
FROM Products
WHERE Price BETWEEN
(SELECT CAST(SUBSTRING_INDEX(@PriceRange, ',', 1) AS DECIMAL(10, 2)))
AND
(SELECT CAST(SUBSTRING_INDEX(@PriceRange, ',', -1) AS DECIMAL(10, 2)));
Output:
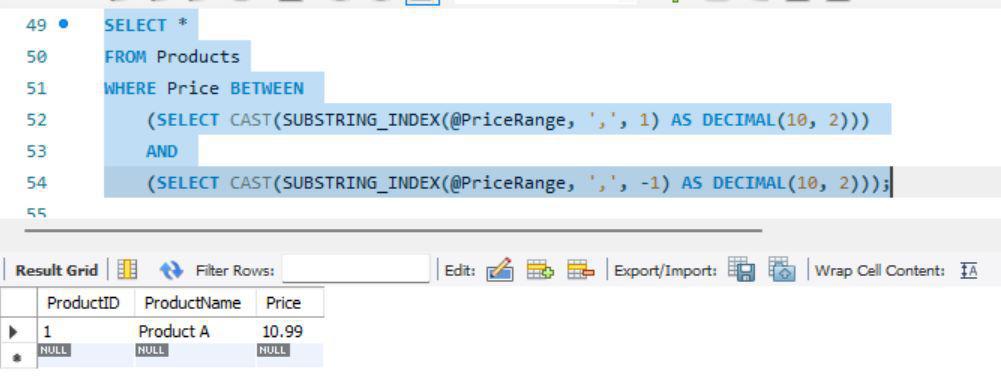
Parameterizing with Price Range
Explanation: In this example, I have set a parameter ‘@PriceRange‘ that represents a dynamic range of prices. Then the ‘BETWEEN‘ clause is used to filter the necessary rows where the product price falls within the specified range.
This shows how parameterizing the IN clause can extend to various scenarios. Also, it offers the adaptability to different filtering criteria.
Conclusion
Parameterizing the ‘IN‘ clause in SQL is a valuable practice for creating dynamic and secure queries. When working with SQL, by using variables or parameters, you can improve the flexibility and reusability of your MySQL code, and make it easier to adapt to changing the requirements as well. Additionally, this approach promotes efficient code maintenance and adaptability to evolving database needs, fostering a robust and scalable SQL environment.
Share your thoughts in the comments
Please Login to comment...