In MySQL, the IN clause is a powerful tool for filtering data based on a specified list of values. To enhance flexibility and security, parameterizing the IN clause is a recommended practice. In this article, we will understand the Parameterize a MySQL IN clause with the practice of parameterizing a MySQL IN clause. We’ll break down the syntax involved, offering a comprehensive overview to explain why this approach is used and its significance in database operations.
Introduction to Parameterize an IN clause in MySQL
Parameterizing IN
a clause in MySQL involves using placeholders (?
) instead of static values and binding them with actual values later. The IN
clause is a powerful tool for filtering data based on a specified list of values. Parameterizing this clause provides flexibility, security, and performance benefits in MySQL queries.
Here is the basic syntax of a parameterized IN
clause:
Syntax:
SELECT column1, column2, ...
FROM your_table
WHERE your_column IN (?, ?, ...);
Explanation: The basic syntax of a parameterized IN clause involves using placeholders (?) instead of static values and binding them with actual values later. here as syntax follows.
Parameterizing the IN clause offers Usage:
- Dynamic Queries: It allows the construction of dynamic queries where the list of values can change based on user input, application logic, or other dynamic factors.
- Prevents SQL Injection: By using parameterized queries, you mitigate the risk of SQL injection attacks. Binding values securely prevents malicious input from interfering with the query execution.
- Improved Performance: Parameterized queries can be cached by the database, leading to improved performance as the execution plan is reused for similar queries.
Examples of Parameterize a MySQL IN clause
Example 1: Parameterized IN clause with Dynamic Search
In this example, we have created the Database as an Example we have a ‘users‘ table with columns user_id, username, and email. The goal is to dynamically search for users based on a dynamic list of user IDs.
-- SQL Code
CREATE DATABASE Clause;
USE Clause;
-- Sample users table
CREATE TABLE users
(
user_id INT PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(100)
);
-- Parameterized IN clause with dynamic search
SET @user_ids = '1, 3, 5'; -- Simulate user input
SELECT user_id, username, email
FROM users
WHERE user_id IN (SELECT CAST(value AS UNSIGNED) FROM STRING_SPLIT(@user_ids, ','));
Output:

Output
Explanation: This query retrieves users with IDs 1, 3, and 5 based on the user-provided list stored in the @user_ids variable.
Example 2: Dynamic Category Filtering in Product Search
Using the same database we have a ‘products’ table with columns product_id, product_name, and category. The objective is to retrieve products based on dynamic category filtering.
-- SQL Code
CREATE DATABASE Example ;
USE Example;
-- Sample 'products' table
CREATE TABLE products (
product_id INT PRIMARY KEY,
product_name VARCHAR(100),
category VARCHAR(50)
);
-- Parameterized IN clause example
SET @categories = 'Electronics, Furniture'; -- Simulating dynamic input
SELECT product_id, product_name, category
FROM products
WHERE category IN (SELECT TRIM(value) FROM STRING_SPLIT(@categories, ','));
Output:

Explanation: In this query, we have retrieved the products based on dynamic category filtering with categories like ‘Electronics‘ and ‘Furniture‘
Example 3: Limitations of Static IN Clauses
CREATE DATABASE Clause;
USE Clause;
-- Creating tables for examples
CREATE TABLE orders (
order_id INT PRIMARY KEY,
product VARCHAR(50),
status VARCHAR(50)
);
CREATE TABLE products (
product_id INT PRIMARY KEY,
product VARCHAR(50),
category VARCHAR(50)
);
-- Inserting sample data
INSERT INTO orders (order_id, product, status) VALUES
(1, 'Laptop', 'Shipped'),
(2, 'Smartphone', 'Delivered'),
(3, 'Tablet', 'Shipped'),
(4, 'Camera', 'In Progress');
INSERT INTO products (product_id, product, category) VALUES
(1, 'Laptop', 'Electronics'),
(2, 'Smartphone', 'Electronics'),
(3, 'Tablet', 'Electronics'),
(4, 'Camera', 'Electronics');
Output:
There is no specific output for this part as it serves as an introduction to the limitations of static IN clauses.
Example 4: Parameterizing IN Clause with Variables
Using the above code run the below query.
Query:
-- Parameterized IN Clause with Variables
SET @product1 = 'Laptop';
SET @product2 = 'Smartphone';
SET @product3 = 'Tablet';
SELECT * FROM orders WHERE product IN (@product1, @product2, @product3);
Output:
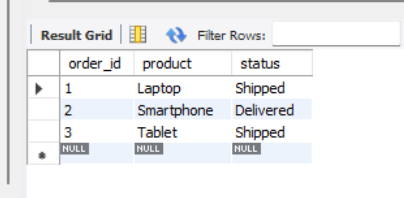
Explanation: The output for both queries will be the same, displaying information about orders for the specified products (‘Laptop’, ‘Smartphone’, ‘Tablet’).
Example 5: Using Prepared Statements for Parameterization
For increased security and performance, use a prepared statement.
Query:
-- Using Prepared Statements
SET @product_list = 'Laptop, Smartphone, Tablet';
SET @query = CONCAT('SELECT * FROM orders WHERE product IN (', @product_list, ')');
PREPARE stmt FROM @query;
EXECUTE stmt;
DEALLOCATE PREPARE stmt;
Output:

Prepared Statements for Parameterization Output
Explanation: This Query will also give the same output for both queries will be the same, displaying information about orders for the specified products (‘Laptop’, ‘Smartphone’, ‘Tablet’).
Example 6: Parameterizing with Subqueries
Want to filter based on values retrieved from another query? Use a subquery
Query:
-- Parameterized IN Clause with Subquery
SELECT * FROM orders WHERE product IN (SELECT DISTINCT product FROM products WHERE category = 'Electronics');
Output:
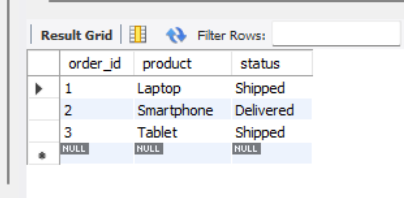
Parameterizing with Subqueries Output
Explanation: The output will display information about orders where the product is in the distinct list of products from the ‘Electronics’ category.
Example 7: Handling Null Values in Parameterized IN Clauses
Null values in parameterized IN clauses require careful handling. Use IS NULL or IS NOT NULL clauses appropriately.
Query:
-- Parameterized IN Clause with NULL Handling
SET @product_list = 'Laptop, Smartphone, Tablet, NULL';
SET @query = CONCAT('SELECT * FROM orders WHERE product IN (', @product_list, ')');
PREPARE stmt FROM @query;
EXECUTE stmt;
DEALLOCATE PREPARE stmt;
Output:
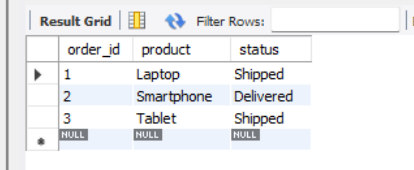
Handling Null Values in Parameterized Output
Explanation: The output will display information about orders for the specified products (‘Laptop’, ‘Smartphone’, ‘Tablet’) and orders where the product is NULL.
Example 8: Combining Parameterized IN Clauses
Complex filtering can involve combining multiple parameterized IN clauses using AND/OR operators.
Query:
-- Combining Parameterized IN Clauses
SET @product_list = 'Laptop, Smartphone, Tablet';
SET @status_list = 'Shipped, Delivered';
SET @query = CONCAT('SELECT * FROM orders WHERE product IN (', @product_list, ') AND status IN (', @status_list, ')');
PREPARE stmt FROM @query;
EXECUTE stmt;
DEALLOCATE PREPARE stmt;
Output:
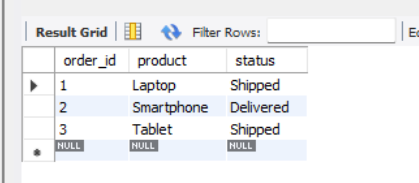
Combining Parameterized Output
Explanation: The output will display information about orders where the product is in the specified list and the status is in the specified list.
Conclusion
Parameterizing the IN clause in MySQL is a best practice that brings flexibility, security, and performance benefits to your queries. By allowing dynamic construction of the list of values and ensuring secure binding, this approach empowers developers to create robust and efficient database interactions. Adopting parameterized queries is a key step in building secure and adaptable database applications.
Share your thoughts in the comments
Please Login to comment...