Pairwise Swap Nodes of a given Linked List
Last Updated :
01 Feb, 2023
Given a singly linked list, write a function to swap elements pairwise.
Input : 1->2->3->4->5->6->NULL
Output : 2->1->4->3->6->5->NULL
Input : 1->2->3->4->5->NULL
Output : 2->1->4->3->5->NULL
Input : 1->NULL
Output : 1->NULL
For example, if the linked list is 1->2->3->4->5 then the function should change it to 2->1->4->3->5, and if the linked list is then the function should change it to.
METHOD 1 (Iterative)
Start from the head node and traverse the list. While traversing swap data of each node with its next node’s data.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
class Node {
public :
int data;
Node* next;
};
void pairWiseSwap(Node* head)
{
Node* temp = head;
while (temp != NULL && temp->next != NULL) {
swap(temp->data,
temp->next->data);
temp = temp->next->next;
}
}
void push(Node** head_ref, int new_data)
{
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList(Node* node)
{
while (node != NULL) {
cout << node->data << " " ;
node = node->next;
}
}
int main()
{
Node* start = NULL;
push(&start, 5);
push(&start, 4);
push(&start, 3);
push(&start, 2);
push(&start, 1);
cout << "Linked list "
<< "before calling pairWiseSwap()\n" ;
printList(start);
pairWiseSwap(start);
cout << "\nLinked list "
<< "after calling pairWiseSwap()\n" ;
printList(start);
return 0;
}
|
C
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void swap( int * a, int * b);
void pairWiseSwap( struct Node* head)
{
struct Node* temp = head;
while (temp != NULL && temp->next != NULL) {
swap(&temp->data, &temp->next->data);
temp = temp->next->next;
}
}
void swap( int * a, int * b)
{
int temp;
temp = *a;
*a = *b;
*b = temp;
}
void push( struct Node** head_ref, int new_data)
{
struct Node* new_node = ( struct Node*) malloc ( sizeof ( struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList( struct Node* node)
{
while (node != NULL) {
printf ( "%d " , node->data);
node = node->next;
}
}
int main()
{
struct Node* start = NULL;
push(&start, 5);
push(&start, 4);
push(&start, 3);
push(&start, 2);
push(&start, 1);
printf ( "Linked list before calling pairWiseSwap()\n" );
printList(start);
pairWiseSwap(start);
printf ( "\nLinked list after calling pairWiseSwap()\n" );
printList(start);
return 0;
}
|
Java
import java.io.*;
class LinkedList {
Node head;
class Node {
int data;
Node next;
Node( int d)
{
data = d;
next = null ;
}
}
void pairWiseSwap()
{
Node temp = head;
while (temp != null && temp.next != null ) {
int k = temp.data;
temp.data = temp.next.data;
temp.next.data = k;
temp = temp.next.next;
}
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
System.out.print(temp.data + " " );
temp = temp.next;
}
System.out.println();
}
public static void main(String args[])
{
LinkedList llist = new LinkedList();
llist.push( 5 );
llist.push( 4 );
llist.push( 3 );
llist.push( 2 );
llist.push( 1 );
System.out.println( "Linked List before calling pairWiseSwap() " );
llist.printList();
llist.pairWiseSwap();
System.out.println( "Linked List after calling pairWiseSwap() " );
llist.printList();
}
}
|
Python
class Node:
def __init__( self , data):
self .data = data
self . next = None
class LinkedList:
def __init__( self ):
self .head = None
def pairwiseSwap( self ):
temp = self .head
if temp is None :
return
while (temp and temp. next ):
if (temp.data ! = temp. next .data):
temp.data, temp. next .data = temp. next .data, temp.data
temp = temp. next . next
def push( self , new_data):
new_node = Node(new_data)
new_node. next = self .head
self .head = new_node
def printList( self ):
temp = self .head
while (temp):
print temp.data,
temp = temp. next
llist = LinkedList()
llist.push( 5 )
llist.push( 4 )
llist.push( 3 )
llist.push( 2 )
llist.push( 1 )
print "Linked list before calling pairWiseSwap() "
llist.printList()
llist.pairwiseSwap()
print "\nLinked list after calling pairWiseSwap()"
llist.printList()
|
C#
using System;
class LinkedList {
Node head;
public class Node {
public int data;
public Node next;
public Node( int d)
{
data = d;
next = null ;
}
}
void pairWiseSwap()
{
Node temp = head;
while (temp != null && temp.next != null ) {
int k = temp.data;
temp.data = temp.next.data;
temp.next.data = k;
temp = temp.next.next;
}
}
public void push( int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
void printList()
{
Node temp = head;
while (temp != null ) {
Console.Write(temp.data + " " );
temp = temp.next;
}
Console.WriteLine();
}
public static void Main(String[] args)
{
LinkedList llist = new LinkedList();
llist.push(5);
llist.push(4);
llist.push(3);
llist.push(2);
llist.push(1);
Console.WriteLine( "Linked List before calling pairWiseSwap() " );
llist.printList();
llist.pairWiseSwap();
Console.WriteLine( "Linked List after calling pairWiseSwap() " );
llist.printList();
}
}
|
Javascript
<script>
var head;
class Node {
constructor(val) {
this .data = val;
this .next = null ;
}
}
function pairWiseSwap() {
var temp = head;
while (temp != null && temp.next != null ) {
var k = temp.data;
temp.data = temp.next.data;
temp.next.data = k;
temp = temp.next.next;
}
}
function push(new_data) {
var new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
function printList() {
var temp = head;
while (temp != null ) {
document.write(temp.data + " " );
temp = temp.next;
}
document.write( "<br/>" );
}
push(5);
push(4);
push(3);
push(2);
push(1);
document.write(
"Linked List before calling pairWiseSwap() <br/>"
);
printList();
pairWiseSwap();
document.write(
"Linked List after calling pairWiseSwap()<br/> "
);
printList();
</script>
|
Output
Linked list before calling pairWiseSwap()
1 2 3 4 5
Linked list after calling pairWiseSwap()
2 1 4 3 5
Time complexity: O(N), As we traverse the linked list only once.
Auxiliary Space: O(1), As constant extra space is used.
METHOD 2 (Recursive)
If there are 2 or more than 2 nodes in Linked List then swap the first two nodes and recursively call for the rest of the list.
Below image is a dry run of the above approach:
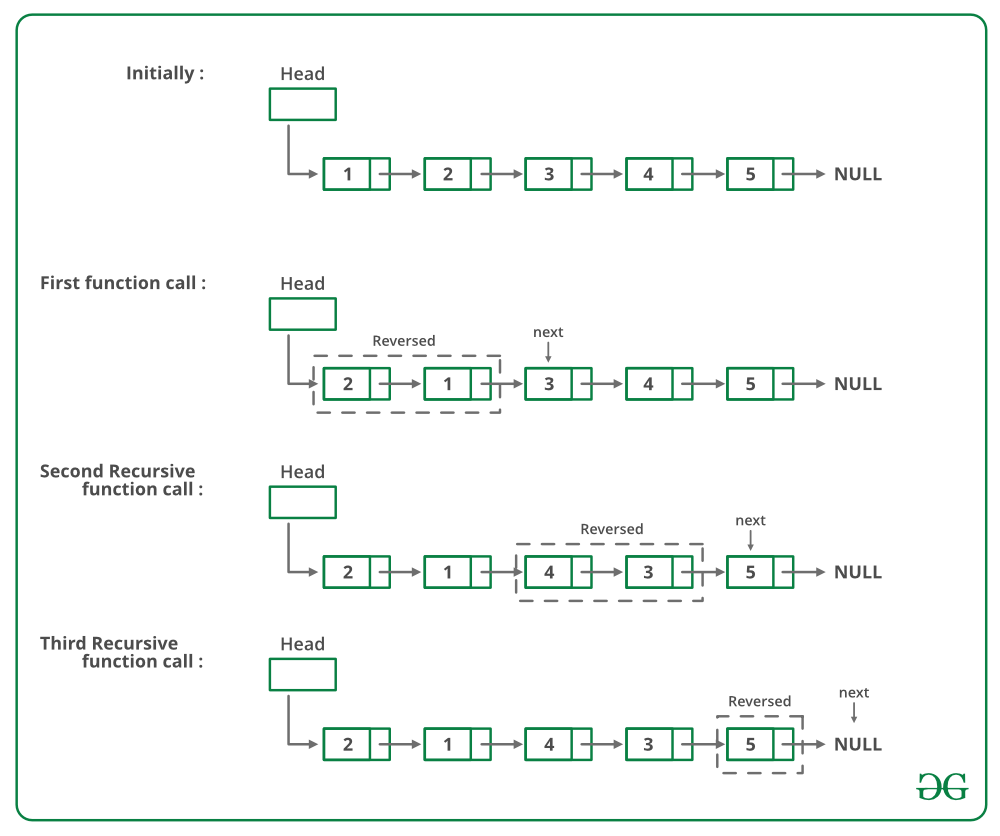
Below is the implementation of the above approach:
C++
void pairWiseSwap(node* head)
{
if (head != NULL && head->next != NULL) {
swap(head->data, head->next->data);
pairWiseSwap(head->next->next);
}
}
|
C
void pairWiseSwap( struct node* head)
{
if (head != NULL && head->next != NULL) {
swap(head->data, head->next->data);
pairWiseSwap(head->next->next);
}
}
|
Python3
def pairWiseSwap(head):
if (head ! = None and head. next ! = None ):
swap(head.data, head. next .data)
pairWiseSwap(head. next . next )
|
C#
static void pairWiseSwap(node head)
{
if (head != null && head.next != null ) {
swap(head.data, head.next.data);
pairWiseSwap(head.next.next);
}
}
|
Javascript
<script>
function pairWiseSwap(head)
{
if (head != null && head.next != null ) {
swap(head.data, head.next.data);
pairWiseSwap(head.next.next);
}
}
</script>
|
Java
public static void pairWiseSwap(Node head)
{
if (head != null && head.next != null ) {
int temp = head.data;
head.data = head.next.data;
head.next.data = temp;
pairWiseSwap(head.next.next);
}
}
|
Time complexity: O(n)
Auxiliary Space: O(1), As it is a tail recursive function, function call stack would not be build and thus no extra space will be used.
The solution provided here swaps data of nodes. If the data contains many fields (for example a linked list of Student Objects), the swap operation will be costly. See the below article for a better solution that works well for all kind of linked lists
Pairwise Swap Nodes by Changing Links
Please write comments if you find any bug in the above code/algorithm, or find other ways to solve the same problem.
Share your thoughts in the comments
Please Login to comment...