Organic Tree
Last Updated :
10 Jan, 2024
Given the value of k and n (the number of nodes of type A) in the tree, compute the total number of nodes of type B present in the tree. Each node of type A is required to have exactly k edges, while each node of type B should have only one edge.
Note: A tree with m nodes has m – 1 edges and no cycle.
Examples:
Input: n = 2, k = 4
Output: 6
Explanation: The tree is given below where 3 B’s are connected to each A, which are connected to each other.
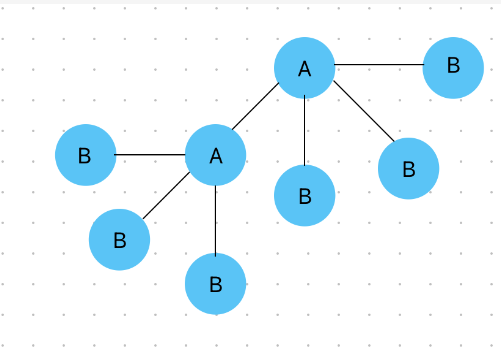
Input: n = 3, k = 5
Output: 11
Explanation: One of the valid trees is given below.
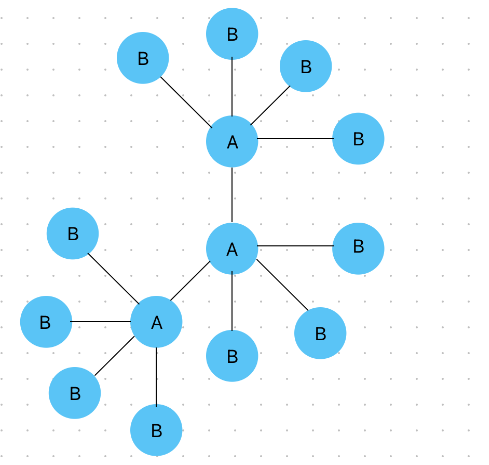
Approach: We can solve this problem using below Idea.
This problem can be solved by observation and maths. We can see that B is always going to be a leaf node and A will be our internal nodes . No of B nodes will not depend upon the structure of Tree. It will always equal to n*(k-2) + 2 .
Steps to solve this problem:
- Intialise a cnt = 0 which will be number of B types node.
- As there are n, A types nodes:
- Subtract A nodes from cnt.
- Return cnt, which will be B nodes.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
#include <iostream>
using namespace std;
long long countB( int n, int k)
{
long long sum = 0;
sum = n * 1ll * k;
sum -= n;
sum -= n - 2;
return sum;
}
int main()
{
int n = 4;
int k = 2;
cout << countB(n, k);
return 0;
}
|
Java
class GFG {
static long countB( int n, int k)
{
long sum = 0 ;
sum = n * 1L * k;
sum -= n;
sum -= n - 2 ;
return sum;
}
public static void main(String[] args)
{
int n = 4 ;
int k = 2 ;
System.out.println(countB(n, k));
}
}
|
Python3
def count_b(n, k):
sum_value = 0
sum_value = n * k
sum_value - = n
sum_value - = n - 2
return sum_value
if __name__ = = "__main__" :
n = 4
k = 2
print (count_b(n, k))
|
C#
using System;
class GFG
{
static long CountB( int n, int k)
{
long sum = 0;
sum = n * 1L * k;
sum -= n;
sum -= n - 2;
return sum;
}
public static void Main( string [] args)
{
int n = 4;
int k = 2;
Console.WriteLine(CountB(n, k));
}
}
|
Javascript
function countB(n, k) {
let sum = 0;
sum = BigInt(n) * BigInt(k);
sum -= BigInt(n);
sum -= BigInt(n - 2);
return sum;
}
const n = 4;
const k = 2;
console.log(countB(n, k).toString());
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...