Number Sequences Generator using HTML CSS and JavaScript
Last Updated :
16 Nov, 2023
In this article, we are going to implement a number sequence generator based on HTML, CSS, and JavaScript. In this program, we have three different types of sequences: the Fibonacci Series, the Prime Number Series, and the Even Odd Number Series.
Preview of final output: Let us have a look at how the final output will look like.
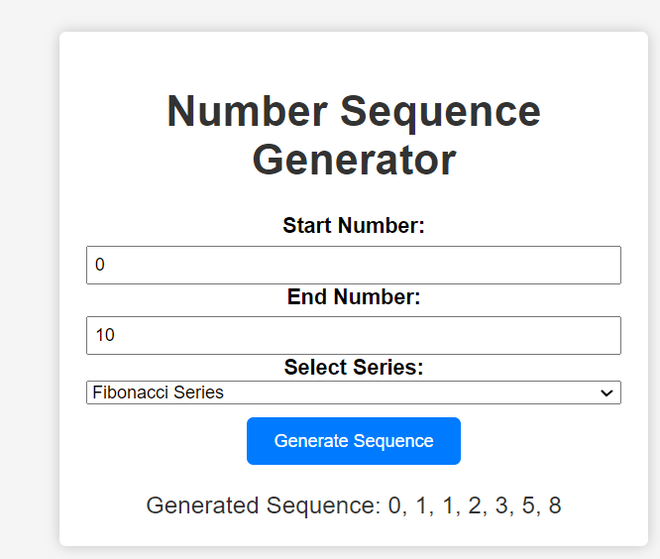
Number Sequence Generator output
Prerequisites
Approach to create Number Sequence Generator
In this method, we have integrated three input fields; two for specifying the range and one for selecting the type of series the user wishes to generate. Additionally, we have incorporated a check for the range input. If the user enters an initial number greater than the final number, the program will prompt an error, emphasizing that the initial number should be smaller than the final number. Finally, there is a ‘Generate Sequence’ button which generates the chosen sequence within the specified range.
Example: This example describes the basic implementation of How to Create a Number Sequences Generator Project using HTML CSS & JavaScript.
Javascript
document.addEventListener(
"DOMContentLoaded" ,
function () {
const startInput =
document.getElementById(
"start"
);
const endInput =
document.getElementById(
"end"
);
const generateButton =
document.getElementById(
"generate"
);
const sequenceDiv =
document.getElementById(
"sequence"
);
const seriesSelect =
document.getElementById(
"series"
);
generateButton.addEventListener(
"click" ,
function () {
const start = parseInt(
startInput.value
);
const end = parseInt(
endInput.value
);
const selectedSeries =
seriesSelect.value;
if (
isNaN(start) ||
isNaN(end)
) {
sequenceDiv.textContent =
"Please enter valid numbers." ;
} else if (
start > end
) {
sequenceDiv.textContent =
`Start number should be less than
or equal to end number.`;
} else {
let sequence = [];
if (
selectedSeries ===
"fibonacci"
) {
sequence =
generateFibonacciSeries(
start,
end
);
} else if (
selectedSeries ===
"prime"
) {
sequence =
generatePrimeNumbers(
start,
end
);
} else if (
selectedSeries ===
"odd-even"
) {
sequence =
generateOddEvenSeries(
start,
end
);
}
sequenceDiv.textContent =
"Generated Sequence: " +
sequence.join(
", "
);
}
}
);
function generateFibonacciSeries(
start,
end
) {
let sequence = [0, 1];
if (start > 0) {
let a = 0;
let b = 1;
while (b <= start) {
let nextFib = a + b;
a = b;
b = nextFib;
}
sequence = [a, b];
}
while (
sequence[
sequence.length - 1
] <= end
) {
let nextFib =
sequence[
sequence.length -
1
] +
sequence[
sequence.length -
2
];
if (nextFib <= end) {
sequence.push(
nextFib
);
} else {
break ;
}
}
sequence = sequence.filter(
(num) =>
num >= start &&
num <= end
);
return sequence;
}
function generatePrimeNumbers(
start,
end
) {
let sequence = [];
for (
let num = Math.max(
start,
2
);
num <= end;
num++
) {
if (isPrime(num)) {
sequence.push(num);
}
}
return sequence;
}
function isPrime(num) {
if (num <= 1) return false ;
if (num <= 3) return true ;
if (
num % 2 === 0 ||
num % 3 === 0
)
return false ;
let i = 5;
while (i * i <= num) {
if (
num % i === 0 ||
num % (i + 2) === 0
)
return false ;
i += 6;
}
return true ;
}
function generateOddEvenSeries(
start,
end
) {
let sequence = [];
for (
let num = start;
num <= end;
num++
) {
sequence.push(
num +
(num % 2 === 0
? " (EVEN)"
: " (ODD)" )
);
}
return sequence;
}
}
);
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Number Sequence Generator</ title >
< link rel = "stylesheet" href = "styles.css" >
</ head >
< body >
< div class = "container" >
< h1 >Number Sequence Generator</ h1 >
< div class = "input-container" >
< label for = "start" >
Start Number:
</ label >
< input type = "number" id = "start" min = "1" value = "1" >
< label for = "end" >
End Number:
</ label >
< input type = "number" id = "end" min = "1" value = "10" >
< label for = "series" >
Select Series:
</ label >
< select id = "series" >
< option value = "fibonacci" >
Fibonacci Series
</ option >
< option value = "prime" >
Prime Numbers Series
</ option >
< option value = "odd-even" >
ODD | EVEN Series
</ option >
</ select >
</ div >
< button id = "generate" >
Generate Sequence
</ button >
< div id = "sequence" class = "result" ></ div >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
background-color : #f5f5f5 ;
text-align : center ;
margin : 0 ;
padding : 0 ;
}
.container {
background-color : #fff ;
max-width : 400px ;
margin : 50px auto ;
padding : 20px ;
border-radius: 5px ;
box-shadow: 0 0 10px
rgba( 0 , 0 , 0 , 0.2 );
}
h 1 {
color : #333 ;
}
.input-container {
display : flex;
flex- direction : column;
margin-bottom : 10px ;
}
label {
font-weight : bold ;
}
input {
padding : 5px ;
margin-top : 5px ;
}
button {
background-color : #007bff ;
color : #fff ;
padding : 10px 20px ;
border : none ;
border-radius: 5px ;
cursor : pointer ;
}
.result {
margin-top : 20px ;
font-size : 18px ;
color : #333 ;
}
|
Output
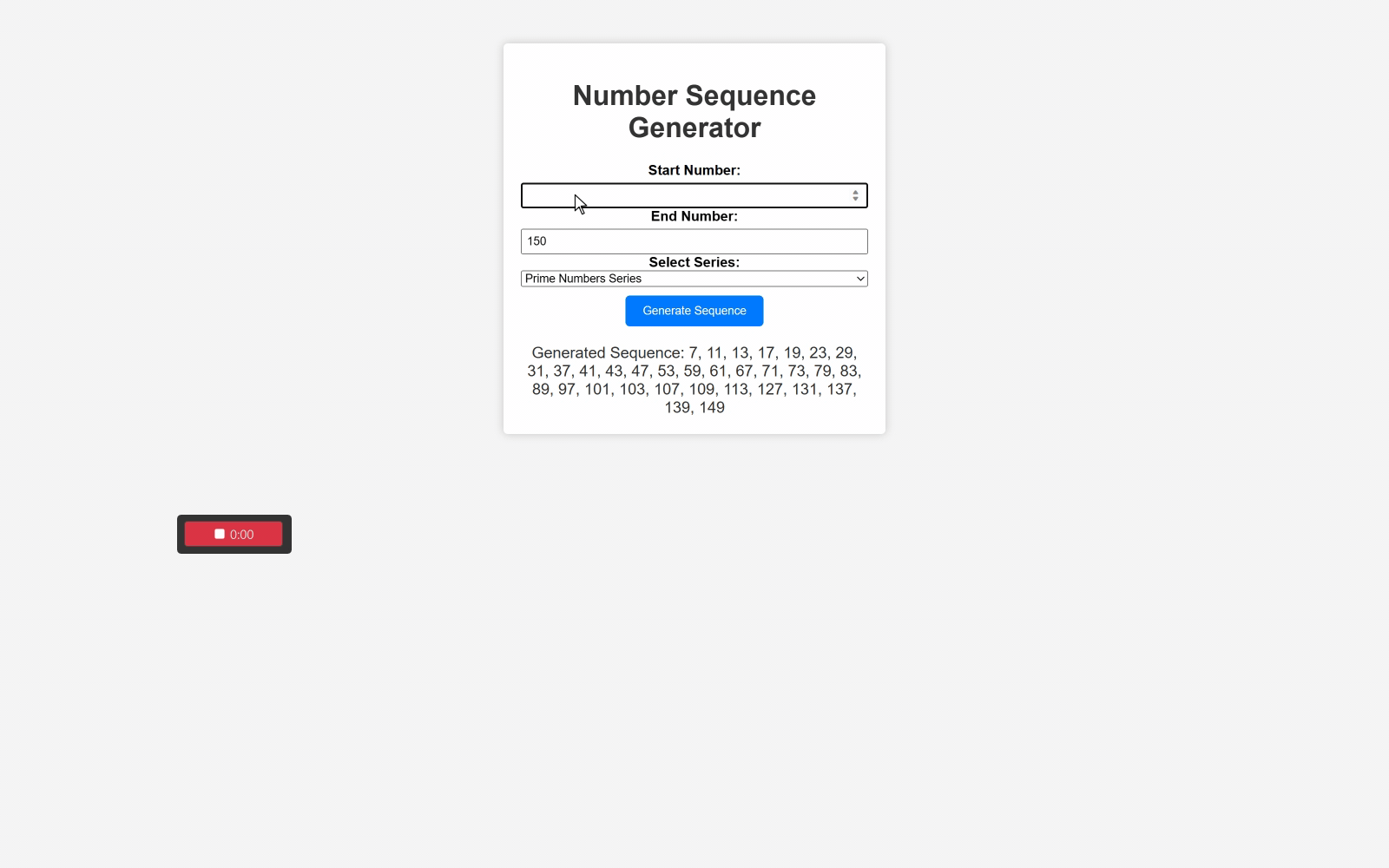
Share your thoughts in the comments
Please Login to comment...