NPM Typescript
Last Updated :
10 May, 2024
Node Package Manager (npm) is a package manager for NodeJS and JavaScript, which is mainly used for installing, managing, and sharing reusable code packages. TypeScript, on the other hand, is a superset of JavaScript that adds static typing, enabling developers to write more robust and scalable code with features like type annotations, interfaces, and type checking during compilation.
Prerequisites:
Features of npm typescript
- Package Management: npm provides a vast repository of packages for NodeJS and JavaScript, making it easy to install, manage, and share dependencies.
- Static Typing: TypeScript introduces static typing to JavaScript, enabling developers to catch type-related errors during development and improve code quality and reliability.
- Type Definitions: TypeScript’s ecosystem includes type definitions (often referred to as “typings“) for popular libraries and frameworks, facilitating better code documentation and tooling support.
- Compiler: TypeScript comes with a compiler that translates TypeScript code into plain JavaScript, allowing developers to use modern JavaScript features while targeting different ECMAScript versions for compatibility.
Steps to Create Application and Installing TypeScript Package
Step 1: First, create the folder named npm-typescript using the command mkdir npm-typescript in your terminal or command prompt.
mkdir npm-typescript
Step 2: Navigate to the newly created folder using the cd npm-typescript command.
cd npm-typescript
Step 3: Initialize npm in the folder by running npm init -y to create a package.json file.
npm init -y
Step 4: Install TypeScript globally using npm install typescript, or locally in your project using npm install typescript –save-dev for development dependencies.
npm install typescript
Step 5: Create an index.ts file.
Project Structure:
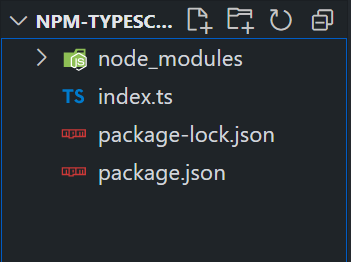
The updated dependencies in package.json file will look like:
"dependencies": {
"typescript": "^5.4.5"
}
Example 1: In this example, a generic function reverseArray is defined to reverse arrays of any type T, demonstrated with both a numeric and string array.
JavaScript
// index.ts
function reverseArray<T>(array: T[]): T[] {
return array.reverse();
}
let numbers: number[] = [1, 2, 3, 4, 5];
let reversedNumbers = reverseArray(numbers);
console.log(reversedNumbers);
let strings: string[] = ["apple", "banana", "cherry"];
let reversedStrings = reverseArray(strings);
console.log(reversedStrings);
To compile and run these TypeScript files, use the TypeScript compiler (tsc).
tsc index.ts
node index.js
Output:
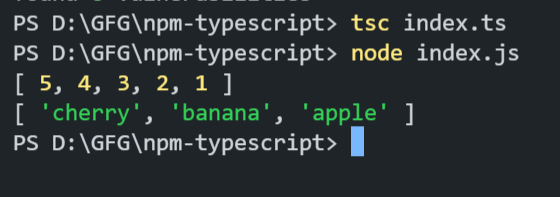
npm typescript output
Example 2: In this example, an interface Calculator is defined with methods for addition and subtraction, implemented in a calculator object literal using arrow functions.
JavaScript
// index.ts
interface Calculator {
add: (a: number, b: number) => number;
subtract: (a: number, b: number) => number;
}
let calculator: Calculator = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
};
console.log(calculator.add(5, 3));
console.log(calculator.subtract(10, 4));
To compile and run these TypeScript files, use the TypeScript compiler (tsc).
tsc index.ts
node index.js
Output:
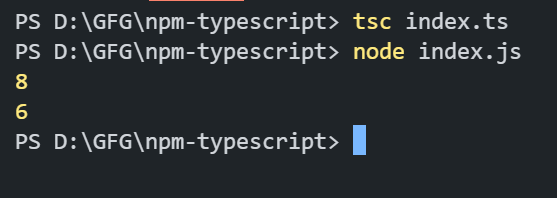
npm typescript output
Share your thoughts in the comments
Please Login to comment...