NPM dotenv
Last Updated :
10 May, 2024
Dotenv is a popular npm (Node Package Manager) package used in NodeJS applications to manage environment variables. Environment variables are values that are set outside of an application’s code and are accessible to the application during runtime. These variables often contain sensitive information like API keys, database connection strings, or configuration settings.
Prerequisites:
Features of dotenv
- Simplified Loading: dotenv simplifies the process of loading environment variables from a ‘.env’ file into Node.js applications, reducing the need for manual configuration.
- Improved security: By keeping sensitive information like API keys, database passwords, and other secrets out of your code and in a separate ‘.env’ file, dotenv helps improve security.
- Compatibility: The package is widely compatible with various Node.js frameworks and libraries, ensuring seamless integration into different types of applications.
Working of dontenv
1. Loading Environment Variables: Dotenv simplifies the process of loading environment variables from a ‘.env’ file into Node.js applications. The ‘.env’ file typically resides in the root directory of the project. It stores key-value pairs of environment variables in a simple format: KEY=VALUE.
_PORT = 3000
API_KEY = localhost
API_PASS= mypassword
2. Parsing and Setting Environment Variables: When you launch your Node.js application, dotenv steps into ‘.env’ file. It reads its contents, and creates individual settings (environment variables) within your program. Through these settings, environment variable are easily accessible throughout your code using process.env.VARIABLE_NAME.
const PORT = process.env.PORT
const API_KEY = process.env.API_KEY
Now make a Project to show use of dotenv for better understanding.
Approach to Implement dotenv npm
- We make a node project and install dotenv package. Create a ‘.env’ file and Environment variables as a key-value pair.
- Integration with Node.js is achieved by importing the dotenv package in the app.js file, and invoked dotenv.config() method. This method reads the .env file, parses its contents, and sets the environment variables in the Node.js runtime environment using process.env. These variables can be accessed throughout the application using process.env.VARIABLE_NAME.
Steps to Setup Application
Step 1: Create a application using the following command:
npm init -y
cd foldername
Step 2: Use npm to install dotenv and express package.
npm i dotenv express
Step 3: Create a ‘.env’ file in the root directory and set your sensitive or Important variables.
_PORT = 8000
API_KEY = 'XXXXXX'
ACCESS_TOKEN = 'XXXXXXX'
Project Structure:
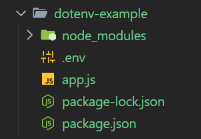
Project Structure
The Updated Dependences in your package.json file is:
"dependencies": {
"dotenv": "^16.4.5",
"express": "^4.19.2"
}
Example: Below is an example of dotenv npm.
JavaScript
// app.js
const express = require('express')
const app = express();
const dotenv = require('dotenv')
// dot env config
dotenv.config()
const PORT = process.env._PORT;
const API_KEY = process.env.API_KEY
const ACCESS_TOKEN = process.env.ACCESS_TOKEN
// use these variable according to your nedd
// Server
app.listen(PORT, () => {
console.log(`Server is Running on PORT No. ${PORT}.`)
})
JavaScript
// .env
_PORT = 8000
API_KEY = 'XXXXXX'
ACCESS_TOKEN = 'XXXXXXX'
Start your application using the following command:
node app.js
Output
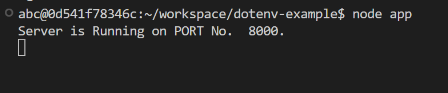
Output
Share your thoughts in the comments
Please Login to comment...