Node Child Process
Last Updated :
18 Jan, 2024
Node is a tool that uses JavaScript and has many useful parts to it. Normally, it does work with one thread at a time, which means it can handle tasks without waiting. However, when there’s a lot of work to be done, we use the child_process module to create additional threads. These extra threads can talk to each other using a built-in messaging system.
The following are the four different ways to create a child process in Nodejs:
Above mentioned ways are explained below:Â
spawn() method:
spawn() method is a new process using the given command and the command line arguments in args. The ChildProcess instance implements EventEmitterAPI which enables us to register handlers for events on child objects directly. Some events that can be registered for handling with the ChildProcess are exit, disconnect, error, close, and message.Â
Syntax:
child_process.spawn(command[, args][, options])
Parameters:
- command: Accepts a string which is the command to run.
- args: List of string arguments. The default value is an empty array.
- options:
- shell: Accepts a boolean value. If true, runs the command inside of a shell. The different shells can be specified as a string. The default value is false which implies no shell. By default, spawn() does not create a shell to execute the command hence it is important to pass it as an option while invoking the child process.
Return Value: Returns a ChildProcess object.Â
Example: In this example, we will see the use of the spawn() method.
Javascript
const { spawn } =
require( 'child_process' );
const child =
spawn(
'dir' , [ 'D:\Test' ],
{ shell: true }
);
child.stdout.on( 'data' ,
(data) => {
console.log(`stdout: ${data}`);
});
child.stderr.on( 'data' ,
(data) => {
console.error(`stderr: ${data}`);
});
child.on( 'close' ,
(code) => {
console.log(
`child process exited with code ${code}`
);
});
|
Output:
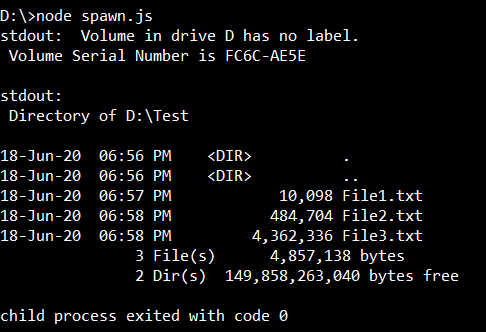
fork() method:
child_process.fork() is a variant of child_process.spawn() allowing communication between parent and child via send(). It facilitates the isolation of compute-heavy tasks from the main event loop, but numerous child processes can impact performance due to each having its own memory.
Syntax:
child_process.fork(modulePath[, args][, options])
Parameters:
- modulePath: Accepts a string that specifies the module to run in the child.
- args: List of string arguments.
- options: CWD, detached, env, execPath, and execArgv are some of the available options for this method.
Return Value: Returns a ChildProcess instance.Â
Example: In this example, we will see the use of fork() method.
Javascript
const cp = require( 'child_process' );
let child = cp.fork(__dirname + '/sub.js' );
child.on( 'message' , function (m) {
console.log( 'Parent process received:' , m);
});
child.send({ hello: 'from parent process' });
child.on( 'close' , (code) => {
console.log(`child process exited with code ${code}`);
});
|
Javascript
process.on( 'message' , function (m) {
console.log( 'Child process received:' , m);
});
process.send({ hello: 'from child process' });
|
Output:

exec() method:
exec() method in JavaScript is used to test for the match in a string. If there is a match this method returns the first match else it returns NULL.
Syntax:
child_process.exec(command[, options][, callback])
Parameters:
- command: Accepts a string that specifies the command to run with space-separated arguments.
- options: Some of the options available are cwd, env, encoding, shell, timeout etc
- callback: The callback function is called when the process terminates. The arguments to this function are error, stdout, and stderr respectively.
Return Value: Returns an instance of ChildProcess.
Example: In this example, we will see the use of the exec() method.
javascript
const { exec } = require( 'child_process' );
exec( 'dir | find /c /v ""' , (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return ;
}
console.log(`stdout: No. of directories = ${stdout}`);
if (stderr != "" )
console.error(`stderr: ${stderr}`);
});
|
Output:

execFile() method:
The child_process.execFile() function is does not spawn a shell by default. It is slightly more efficient than child_process.exec() as the specified executable file is spawned directly as a new process.
 Syntax:
child_process.execFile(file[, args][, options][, callback])
Parameters:
- file: Accepts a string that specifies the name or path of the file to run.
- args: List of string arguments.
- options: Some of the options available are cwd, env, encoding, shell, timeout etc
- callback: The callback function is called when the process terminates. The arguments to this function are error, stdout, and stderr respectively.
Return Value: Returns an instance of ChildProcess.Â
Example: In this example, we will see the use of the execFile() method.
javascript
const { execFile } = require( 'child_process' );
const child = execFile( 'node' , [ 'exec.js' ],
(error, stdout, stderr) => {
if (error) {
throw error;
}
console.log(stdout);
});
|
Output:
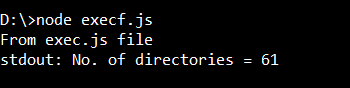
Share your thoughts in the comments
Please Login to comment...