Multilevel Inheritance in Python
Last Updated :
23 Feb, 2024
Python is one of the most popular and widely used Programming Languages. Python is an Object Oriented Programming language which means it has features like Inheritance, Encapsulation, Polymorphism, and Abstraction. In this article, we are going to learn about Multilevel Inheritance in Python.
Pre-Requisite
Python Multilevel Inheritance
Multilevel Inheritance in Python is a type of Inheritance in which a class inherits from a class, which itself inherits from another class. It allows a class to inherit properties and methods from multiple parent classes, forming a hierarchy similar to a family tree. It consists of two main aspects:
- Base class:Â This represents a broad concept.
- Derived classes:Â These inherit from the base class and add specific traits.
Diagram for Multilevel Inheritance in Python
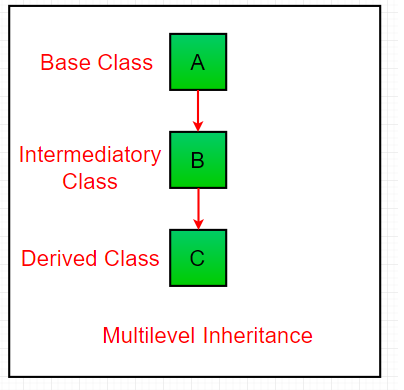
Multilevel Inheritance in Python
Below are some of the examples by which we can understand more about multilevel inheritance in Python:
Example 1: Simple Multilevel Inheritance
In this method, we have three different classes that is Base, Intermediate and derived, and we have inherited the feature of Base to Intermediate and then the feature of Intermediate to derived class.
Python3
class Base:
def __init__( self , name, roll, role):
self .name = name
self .roll = roll
self .role = role
class Intermediate(Base):
def __init__( self , age, name, roll, role):
super ().__init__(name, roll, role)
self .age = age
class Derived(Intermediate):
def __init__( self , age, name, roll, role):
super ().__init__(age, name, roll, role)
def Print_Data( self ):
print (f "The Name is : {self.name}" )
print (f "The Age is : {self.age}" )
print (f "The role is : {self.role}" )
print (f "The Roll is : {self.roll}" )
obj = Derived( 21 , "Lokesh Singh" , 25 , "Software Trainer" )
obj.Print_Data()
|
Output
The Name is : Lokesh Singh
The Age is : 21
The role is : Software Trainer
The Roll is : 25
Example 2: Multilevel Inheritance with Method Overriding
In this method, we have three different class and we have a method in the Intermediate class which we are going to Override in the derived class with the help of Multilevel Inheritance.
Python3
class Shape:
def area( self ):
raise NotImplementedError( "Subclasses must Implement area()" )
class Rectangle(Shape):
def __init__( self , length, width):
self .width = width
self .length = length
def area( self ):
print (f "The Area of Rectangle is {self.length*self.width}" )
class Square(Rectangle):
def __init__( self , side):
super ().__init__(side, side)
def area( self ):
print (f "The Area of Rectangle is {self.length ** 2}" )
Rect = Rectangle( 3 , 4 )
my_sqre = Square( 4 )
Rect.area()
my_sqre.area()
|
Output
The Area of Rectangle is 12
The Area of Rectangle is 16
Share your thoughts in the comments
Please Login to comment...