Minimum value of root node
Last Updated :
13 Dec, 2023
Given an integer X, a tree of N nodes rooted at node 0 and an array P[] of size N, such that P[i] = Parent of node i (P[0] = -1). Assign non-negative values to all the nodes such that the value of the parent node >= sum of immediate child nodes and the sum of all nodes >= X. Find the minimum value that needs to be assigned to the tree’s root.
Examples:
Input: N = 4, X = 7, P = [-1, 0, 1, 2]
Output: 2
Explanation: The minimum value of root is 2.
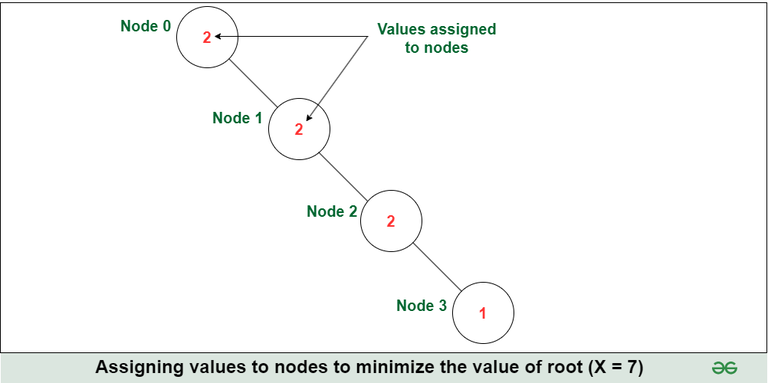
Input: N = 4, X = 10, P = [-1, 0, 1, 0]
Output: 4
Explanation: The minimum value of root is 4.
-768.png)
Approach: This can be solved with the following idea:
We can solve this problem by calculating the maximum depth of a node in the tree. Let’s say there is a node A which has the maximum depth D, then it means we can distribute X equally over all the nodes from root to node A and if we are left with some remainder we can add 1 to the elements which are at the top. So, the minimum value of the root node can be ceil(X/(D + 1)).
Below are the steps involved:
- Construct the graph using array P[].
- Run a DFS to find the maximum depth D of any node.
- Calculate the value ceil(X / (D+1)) and return it.
Below is the implementation of the code:
C++
#include <bits/stdc++.h>
using namespace std;
void dfs(vector< int >* adj, int & D, int node, int depth)
{
D = max(D, depth);
for ( auto child : adj[node]) {
dfs(adj, D, child, depth + 1);
}
}
int optimalValue( int N, int X, vector< int >& P)
{
vector< int > adj[N];
for ( int node = 1; node < N; node++) {
int par = P[node];
adj[par].push_back(node);
}
int D = 0;
dfs(adj, D, 0, 0);
return ceil (X / (1.0 * (D + 1)));
}
int main()
{
int N = 4;
int X = 7;
vector< int > P = { -1, 0, 1, 2 };
cout << optimalValue(N, X, P);
return 0;
}
|
Java
import java.util.ArrayList;
import java.util.List;
class GFG {
public static void dfs(List<Integer>[] adj, int [] D, int node, int depth) {
D[ 0 ] = Math.max(D[ 0 ], depth);
for ( int child : adj[node]) {
dfs(adj, D, child, depth + 1 );
}
}
public static int optimalValue( int N, int X, List<Integer> P) {
List<Integer>[] adj = new List[N];
for ( int i = 0 ; i < N; i++) {
adj[i] = new ArrayList<>();
}
for ( int node = 1 ; node < N; node++) {
int par = P.get(node);
adj[par].add(node);
}
int [] D = new int [ 1 ];
dfs(adj, D, 0 , 0 );
return ( int ) Math.ceil(X / ( 1.0 * (D[ 0 ] + 1 )));
}
public static void main(String[] args) {
int N = 4 ;
int X = 7 ;
List<Integer> P = new ArrayList<>();
P.add(- 1 );
P.add( 0 );
P.add( 1 );
P.add( 2 );
System.out.println(optimalValue(N, X, P));
}
}
|
Python3
def dfs(adj, D, node, depth):
D[ 0 ] = max (D[ 0 ], depth)
for child in adj[node]:
dfs(adj, D, child, depth + 1 )
def optimal_value(N, X, P):
adj = [[] for _ in range (N)]
for node in range ( 1 , N):
par = P[node]
adj[par].append(node)
D = [ 0 ]
dfs(adj, D, 0 , 0 )
return (X / / (D[ 0 ] + 1 )) + (X % (D[ 0 ] + 1 ) > 0 )
N = 4
X = 7
P = [ - 1 , 0 , 1 , 2 ]
print (optimal_value(N, X, P))
|
C#
using System;
using System.Collections.Generic;
class Program
{
public static void Dfs(List< int >[] adj, int [] D, int node, int depth)
{
D[0] = Math.Max(D[0], depth);
foreach ( int child in adj[node])
{
Dfs(adj, D, child, depth + 1);
}
}
public static int OptimalValue( int N, int X, List< int > P)
{
List< int >[] adj = new List< int >[N];
for ( int i = 0; i < N; i++)
{
adj[i] = new List< int >();
}
for ( int node = 1; node < N; node++)
{
int par = P[node];
adj[par].Add(node);
}
int [] D = new int [1];
Dfs(adj, D, 0, 0);
return ( int )Math.Ceiling(X / (1.0 * (D[0] + 1)));
}
static void Main( string [] args)
{
int N = 4;
int X = 7;
List< int > P = new List< int > { -1, 0, 1, 1 };
Console.WriteLine(OptimalValue(N, X, P));
}
}
|
Javascript
function GFG(adj, D, node, depth) {
D[0] = Math.max(D[0], depth);
for (let child of adj[node]) {
GFG(adj, D, child, depth + 1);
}
}
function optimalValue(N, X, P) {
const adj = Array.from({ length: N }, () => []);
for (let node = 1; node < N; node++) {
const par = P[node];
adj[par].push(node);
}
const D = [0];
GFG(adj, D, 0, 0);
return Math.ceil(X / (D[0] + 1));
}
const N = 4;
const X = 7;
const P = [-1, 0, 1, 2];
const result = optimalValue(N, X, P);
console.log(result);
|
Time Complexity: O(N), where N is the number of nodes in the graph
Auxiliary Space: O(N)
Share your thoughts in the comments
Please Login to comment...