MediaMetadataRetriever Class in Android with Examples
Last Updated :
18 Feb, 2021
anMediaMetadataRetriever class provides a unified interface for retrieving frames and metadata from an input media file. It is located under android.media package. For example: retrieving song name, artist name, width or height of the video, video format/mime-type, duration of media, media modified date, etc. Constants/Keys provided by MediaMetadataRetriever class are plentiful. These constants are used to retrieve media information. Although, the work done by many of the Constants is obvious from their name, here is a small description of each constant present in MediaMetadataRetriever class.
Important Constants of MediaMetadataRetriever Class
Constant Type
|
Constant Name
|
Description
|
int |
METADATA_KEY_ALBUM |
The metadata key to retrieve the information about the album title of the data source. |
int |
METADATA_KEY_ALBUMARTIST |
The metadata key to retrieve the information about the performers or artist associated with the data source. |
int |
METADATA_KEY_ARTIST |
The metadata key to retrieve the information about the artist of the data source. |
int |
METADATA_KEY_AUTHOR |
The metadata key to retrieve the information about the author of the data source. |
int |
METADATA_KEY_CD_TRACK_NUMBER |
The metadata key to retrieve the numeric string describing the order of the audio data source on its original recording. |
int |
METADATA_KEY_COMPILATION |
The metadata key to retrieve the music album compilation status. |
int |
METADATA_KEY_COMPOSER |
The metadata key to retrieve the information about the composer of the data source. |
int |
METADATA_KEY_DATE |
The metadata key to retrieve the date when the data source was created or modified. |
int |
METADATA_KEY_DISC_NUMBER |
The metadata key to retrieve the numeric string that describes which part of a set the audio data source comes from. |
int |
METADATA_KEY_DURATION |
The metadata key to retrieve the playback duration of the data source. |
Constant Type
|
Constant Name
|
Description
|
int |
METADATA_KEY_GENRE |
The metadata key to retrieve the content type or genre of the data source. |
int |
METADATA_KEY_MIMETYPE |
The metadata key to retrieve the mime type of the data source. |
int |
METADATA_KEY_NUM_TRACKS |
The metadata key to retrieve the number of tracks, such as audio, video, text, in the data source, such as a mp4 or 3gpp file. |
int |
METADATA_KEY_TITLE |
The metadata key to retrieve the data source title. |
int |
METADATA_KEY_WRITER |
The metadata key to retrieve the information of the writer (such as lyricist) of the data source. |
int |
METADATA_KEY_YEAR |
The metadata key to retrieve the year when the data source was created or modified. |
int |
OPTION_CLOSEST |
This option is used with getFrameAtTime(long, int) to retrieve a frame (not necessarily a keyframe) associated with a data source that is located closest to or at the given time. |
int |
OPTION_CLOSEST_SYNC |
This option is used with getFrameAtTime(long, int) to retrieve a sync (or key) frame associated with a data source that is located closest to (in time) or at the given time. |
int |
OPTION_NEXT_SYNC |
This option is used with getFrameAtTime(long, int) to retrieve a sync (or key) frame associated with a data source that is located right after or at the given time. |
int |
OPTION_PREVIOUS_SYNC |
This option is used with getFrameAtTime(long, int) to retrieve a sync (or key) frame associated with a data source that is located right before or at the given time. |
Methods Available in MediaMetadataRetriever Class
Method Type
|
Methods
|
String |
extractMetadata(int keyCode)
Call this method after setDataSource().
|
byte[] |
getEmbeddedPicture()
Call this method after setDataSource().
|
Bitmap |
getFrameAtTime(long timeUs, int option)
Call this method after setDataSource().
|
Bitmap |
getFrameAtTime(long timeUs)
Call this method after setDataSource().
|
Bitmap |
getFrameAtTime()
Call this method after setDataSource().
|
void |
release()
Call it when one is done with the object.
This method releases the memory allocated internally.
|
void |
setDataSource(FileDescriptor fd, long offset, long length)
Sets the data source (FileDescriptor) to use.
|
void |
setDataSource(String path)
Sets the data source (file pathname) to use.
|
void |
setDataSource(FileDescriptor fd)
Sets the data source (FileDescriptor) to use.
|
void |
setDataSource(Context context, Uri uri)
Sets the data source as a content Uri.
|
void |
Close()
Closes this resource, relinquishing any underlying resources.
This method is invoked automatically on objects managed by
the try-with-resources statement.
|
Examples
1. Get mp3 duration
Here is the sample code snippet in Java to get the mp3 duration.
Java
MediaMetadataRetriever metaRetriever = new MediaMetadataRetriever();
metaRetriever.setDataSource(filePath);
String duration = metaRetriever.extractMetadata(MediaMetadataRetriever.METADATA_KEY_DURATION);
long dur = Long.parseLong(duration);
String seconds = String.valueOf((dur % 60000 ) / 1000 );
String minutes = String.valueOf(dur / 60000 );
String out = minutes + ":" + seconds;
if (seconds.length() == 1 ) {
txtTime.setText( "0" + minutes + ":0" + seconds);
}
else {
txtTime.setText( "0" + minutes + ":" + seconds);
}
metaRetriever.release();
|
2. Detect the orientation of the video
Below is a sample video
Here is the sample code snippet in Java to detect the orientation of the video
Java
MediaMetadataRetriever m = new MediaMetadataRetriever();
m.setDataSource(path);
Bitmap thumbnail = m.getFrameAtTime();
if (Build.VERSION.SDK_INT >= 17 ) {
String s = m.extractMetadata(MediaMetadataRetriever.METADATA_KEY_VIDEO_ROTATION);
}
MediaMetadataRetriever retriever = new MediaMetadataRetriever();
retriever.setDataSource(inputPath);
video_width = Integer.valueOf(retriever.extractMetadata(MediaMetadataRetriever.METADATA_KEY_VIDEO_WIDTH));
video_height = Integer.valueOf(retriever.extractMetadata(MediaMetadataRetriever.METADATA_KEY_VIDEO_HEIGHT));
retriever.release();
if ((video_width > video_height)) {
}
else {
}
|
3. Setting up the Album cover and Album title in a Music App
Below is a sample image
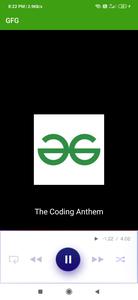
Here is the sample code snippet in Java to setting up the Album cover and Album title in a Music App.
Java
MediaMetadataRetriever retriever = new MediaMetadataRetriever();
retriever.setDataSource(filePath);
byte [] art = retriever.getEmbeddedPicture();
if (art != null ) {
imgAlbum.setImageBitmap(BitmapFactory.decodeByteArray(art, 0 , art.length));
}
else {
imgAlbum.setImageResource(R.drawable.no_image);
}
retriever.release();
|
4. Making a CropVideo Activity like TikTok
Below is a sample video
Here is the sample code snippet in Java to make a CropVideo Activity like TikTok.
Java
try {
MediaMetadataRetriever mediaMetadataRetriever = new MediaMetadataRetriever();
mediaMetadataRetriever.setDataSource(context, videoUri);
long interval = (endPosition - startPosition) / (totalThumbsCount - 1 );
for ( long i = 0 ; i < totalThumbsCount; ++i) {
long frameTime = startPosition + interval * i;
Bitmap bitmap = mediaMetadataRetriever.getFrameAtTime(frameTime * 1000 , MediaMetadataRetriever.OPTION_CLOSEST_SYNC);
if (bitmap == null )
continue ;
try {
bitmap = Bitmap.createScaledBitmap(bitmap, THUMB_WIDTH, THUMB_HEIGHT, false );
}
catch ( final Throwable t) {
t.printStackTrace();
}
}
mediaMetadataRetriever.release();
}
catch ( final Throwable e) {
Thread.getDefaultUncaughtExceptionHandler().uncaughtException(Thread.currentThread(), e);
}
|
Note:
- Always check for illegal filePath.
- Handle the null bitmap condition. There may be cases when the media doesn’t return a valid bitmap.
- The frame index must be that of a valid frame. The total number of frames available for retrieval can be queried via the METADATA_KEY_VIDEO_FRAME_COUNT key.
- When retrieving the frame at the given time position, there is no guarantee that the data source has a frame located at the position. When this happens, a frame nearby will be returned. If time is negative, time position and option will be ignored, and any frame that the implementation considers as representative may be returned.
- setDataSource(), Call this method before the rest of the methods in this class. This method may be time-consuming.
Reference link: https://developer.android.com/reference/android/media/MediaMetadataRetriever
Share your thoughts in the comments
Please Login to comment...