Maximum number of edges that N-vertex graph can have such that graph is Triangle free | Mantel’s Theorem
Last Updated :
23 Apr, 2021
Given a number N which is the number of nodes in a graph, the task is to find the maximum number of edges that N-vertex graph can have such that graph is triangle-free (which means there should not be any three edges A, B, C in the graph such that A is connected to B, B is connected to C and C is connected to A). The graph cannot contain a self-loop or multi edges.
Examples:
Input: N = 4
Output: 4
Explanation:
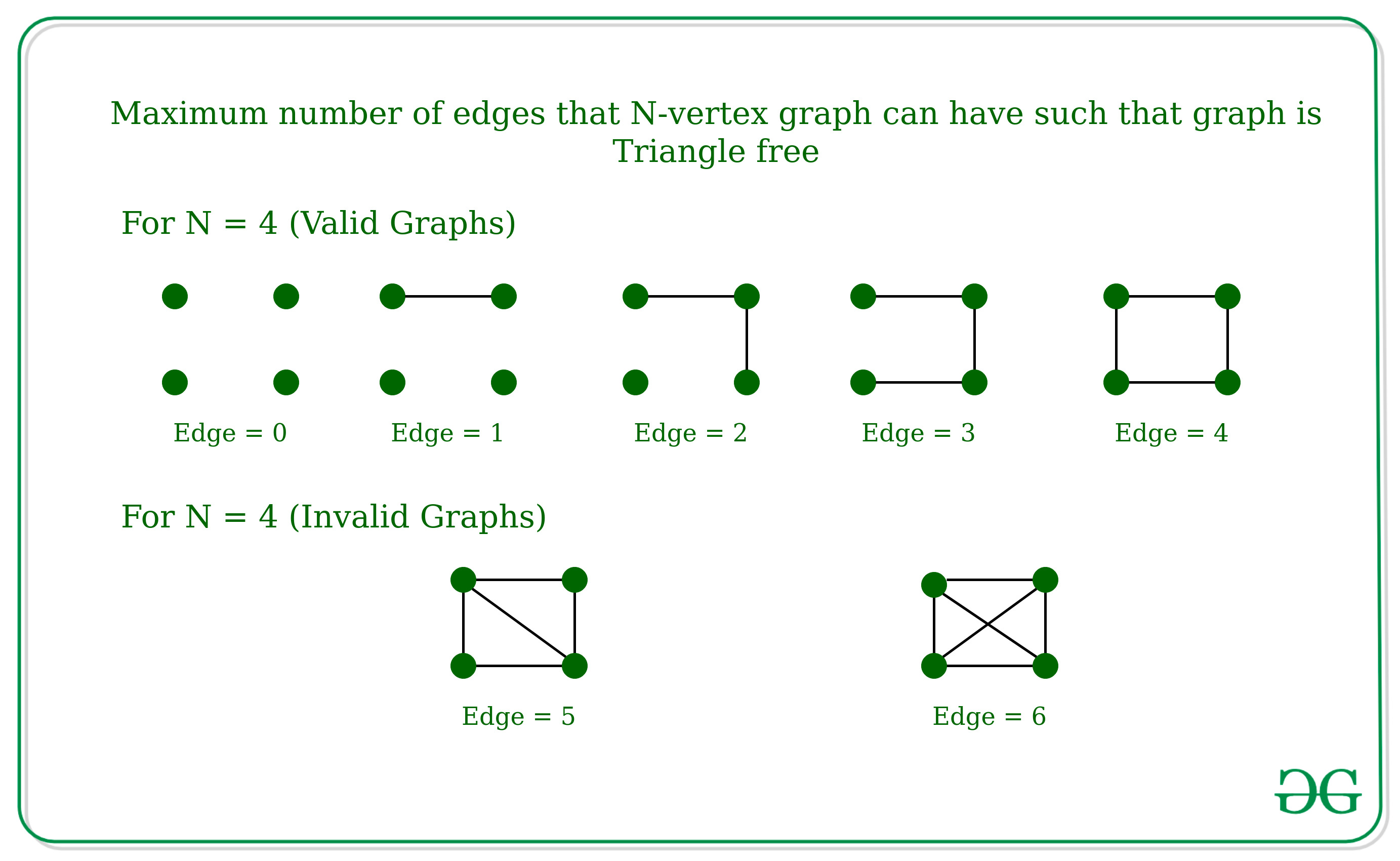
Input: N = 3
Output: 2
Explanation:
If there are three edges in 3-vertex graph then it will have a triangle.
Approach: This Problem can be solved using Mantel’s Theorem which states that the maximum number of edges in a graph without containing any triangle is floor(n2/4). In other words, one must delete nearly half of the edges to obtain a triangle-free graph.
How Mantel’s Theorem Works ?
For any Graph, such that the graph is Triangle free then for any vertex Z can only be connected to any of one vertex from x and y, i.e. For any edge connected between x and y, d(x) + d(y) ? N, where d(x) and d(y) is the degree of the vertex x and y.
- Then, the Degree of all vertex –

- By Cauchy-Schwarz inequality –
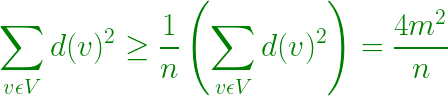
- Therefore, 4m2 / n ? mn, which implies m ? n2 / 4
Below is the implementation of above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int solve( int n)
{
int ans = (n * n / 4);
return ans;
}
int main()
{
int n = 10;
cout << solve(n) << endl;
return 0;
}
|
Java
class GFG
{
public static int solve( int n)
{
int ans = (n * n / 4 );
return ans;
}
public static void main(String args[])
{
int n = 10 ;
System.out.println(solve(n));
}
}
|
C#
using System;
class GFG
{
public static int solve( int n)
{
int ans = (n * n / 4);
return ans;
}
public static void Main()
{
int n = 10;
Console.WriteLine(solve(n));
}
}
|
Python3
def solve(n):
ans = (n * n / / 4 )
return ans
if __name__ = = '__main__' :
n = 10
print (solve(n))
|
Javascript
<script>
function solve(n)
{
var ans = (n * n / 4);
return ans;
}
var n = 10;
document.write(solve(n));
</script>
|
Time Complexity: O(1)
Share your thoughts in the comments
Please Login to comment...