Map True/False to 1/0 in a Pandas DataFrame
Last Updated :
29 Sep, 2023
In this article, we will see how to map True/False to 1/0 in a Pandas DataFrame. The transformation of True/False to 1/0 is vital when carrying out computations and makes it easy to analyze the data. So, we will see how we can convert the same.
Pandas DataFrame Map True/False to 1/0
Below are the methods by which we can map True/False to 1/0 in Pandas DataFrame:
Python Pandas replace()
In this example, we are using the Pandas replace() method to map True/False to 1/0. Here, we create a sample DataFrame called df with two columns, ‘Column1’ and ‘Column2’, containing Boolean values. Then, we use the .replace() method on the DataFrame df. We provide a dictionary where we specify that True should be replaced with 1 and False should be replaced with 0.
Python3
import pandas as pd
data = { 'Column1' : [ True , False , True , False ],
'Column2' : [ False , True , False , True ]}
df = pd.DataFrame(data)
print (df, '\n' )
df = df.replace({ True : 1 , False : 0 })
print (df)
|
Output
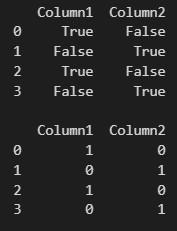
Pandas applymap() function
In this example, we are using Pandas applymap(). Here, e create the sample DataFrame df containing Boolean values. Then, we use the .applymap() function on the DataFrame df. The lambda function lambda x: 1 if x else 0 is applied element-wise to each value in the DataFrame. It checks if the value x is True, and if it is, it returns 1; otherwise, it returns 0.
Python3
import pandas as pd
data = { 'Column1' : [ True , False , True , False ],
'Column2' : [ False , True , False , True ]}
df = pd.DataFrame(data)
print (df, '\n' )
df = df.applymap( lambda x: 1 if x else 0 )
print (df)
|
Output
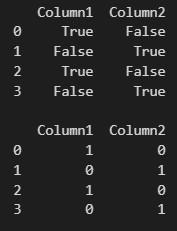
Python Pandas astype() method
In this example, we are using Pandas astype() method to map True/False to 1/0.
Python3
import pandas as pd
data = { 'Column1' : [ True , False , True , False ],
'Column2' : [ False , True , False , True ]}
df = pd.DataFrame(data)
print (df, '/n' )
df[ 'Column1' ] = df[ 'Column1' ].astype( int )
df[ 'Column2' ] = df[ 'Column2' ].astype( int )
print (df)
|
Output
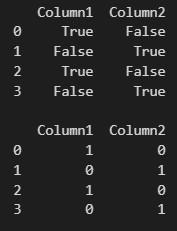
Pandas apply() with lambda function
In this example, we are using Pandas apply() method to map Boolean values to 1/0 in a Pandas DataFrame. Here, we are creating a sample DataFrame named ‘df’ with two columns, ‘Column1’ and ‘Column2’, both containing Boolean values. The code then employs the apply() method along with a lambda function. The lambda function checks each element in the DataFrame and returns 1 if it’s True and 0 if it’s False. This mapping is applied to both ‘Column1’ and ‘Column2’ using .apply(), effectively converting all True values to 1 and all False values to 0. The result is stored in ‘df_apply,’ and the code prints this updated DataFrame.
Python3
import pandas as pd
data = { 'Column1' : [ True , False , True , False ],
'Column2' : [ False , True , False , True ]}
df = pd.DataFrame(data)
print (df, "\n" )
df_apply = df. apply ( lambda x: x. apply ( lambda y: 1 if y else 0 ))
print ( "\nUsing .apply() method with lambda function:" )
print (df_apply)
|
Output
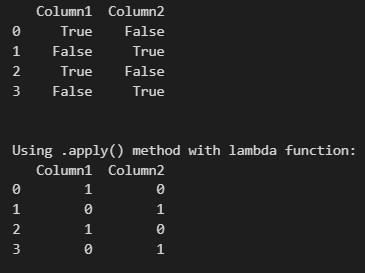
Python Pandas map()
In this example, we are using Pandas map() method to map Boolean values to 1/0 in a Pandas DataFrame. Here, we are creating a sample DataFrame named ‘df’ with two columns, ‘Column1’ and ‘Column2,’ both containing Boolean values. The original DataFrame is then printed to display its initial contents. To perform the mapping, we use the map() method on ‘Column1’ and provide a dictionary that specifies the mapping: True is mapped to 1, and False is mapped to 0. This operation directly converts all True values in ‘Column 1 to 1 and all False values to 0. The updated ‘Column1’ in the original DataFrame ‘df’ reflects this transformation.
Python3
import pandas as pd
data = { 'Column1' : [ True , False , True , False ],
'Column2' : [ False , True , False , True ]}
df = pd.DataFrame(data)
print (df, "\n" )
df[ 'Column1' ] = df[ 'Column1' ]. map ({ True : 1 , False : 0 })
print ( "\nUsing .map() method for 'Column1':" )
print (df)
|
Output
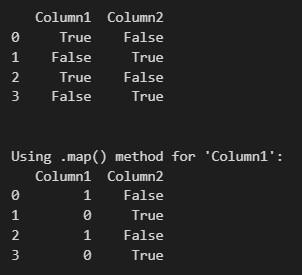
Share your thoughts in the comments
Please Login to comment...