One common way that we interact with the Telegram bot is through the use of keyboard buttons. These buttons give a convenient and intuitive way to input data and perform specific actions within the bot. Without keyboard buttons, we would have to manually type in commands or responses, which could be time-consuming and may lead to errors.
Keyboard buttons also allow us to provide a more user-friendly and engaging experience for their users. By presenting users with clear and well-organized options, bots can guide users through complex workflows or help them quickly access the information or functionality they need.
In this article, we are going to see how to make keyboard buttons in Python with the help of the Aiogram framework.
Aiogram Framework in Python
Aiogram is an open-source asynchronous framework that uses asyncio and aiohttp for building telegram bots. It provides a user-friendly interface for TelegramBotApi, which makes bot development easier and faster. with the help of aiogram, we can easily implement a variety of bot functionality, including sending and receiving messages, managing user interactions with keyboard buttons, handling inline queries, and more.
You can install aiogram by using the below commands in the terminal:
# By using pip
pip install -U aiogram
# By using pipenv
pipenv install aiogram
Types of keyboard buttons in the Telegram Bot
There are two types of keyboard buttons that we can use to enable users to interact with the bot. They are as follows:
i) Â ReplyKeyboardButton: This type of keyboard button is used to present a set of predefined options to the user in a reply keyboard. When the user clicks on a button, the labeled text is sent to the telegram bot as a message. It provides a simple and easy way to interact between the user and the Telegram bot.
ii) InlinekeyboardButton:Â This type of keyboard represents a button of an inline keyboard. These buttons are used to present a set of options to choose from for the users in an inline keyboard to perform a specific task. On clicking the button, the bot receives a callback.
Steps to get a Telegram Bot Token
Let us see how to set up and get the API token for the Telegram Bot.
1. Search for the BotFather bot by typing @BotFather in the search bar.
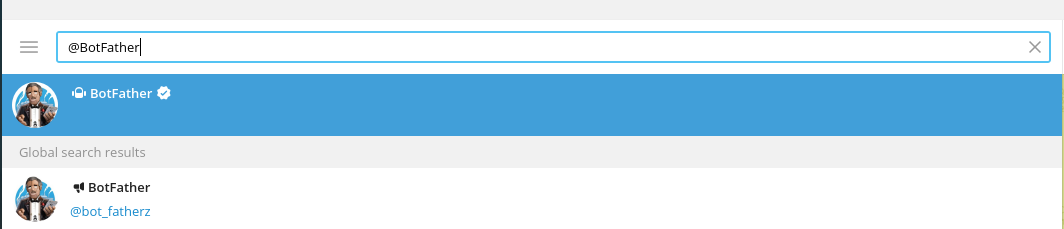
Â
2. Click on it to open the chat with BotFather. Then, click the “Start” button to interact with the BotFather.
3. Enter the command “/newbot” to create a new bot. Then BotFather bot will guide you through the process of creating your bot, including giving your bot a name and a username.
Note: The Username should always be unique and ends with “bot”.
.jpg)
Getting a telegram bot token
4. The above token shown in the image is going to use as a bot token and a key to access Telegram API. Make sure to copy the token and keep it safe.
Note: The minimum version of Python required for using aiogram is Python 3.7 or later.
You can check the version of Python installed on your system by running the following command in your terminal or command prompt:
python --version
InlineKeyboardButton in Telegram Bot using Python
Let us see how we can create Inline Keyboard Button in a Telegram Bot.
Step 1: Importing required libraries
Python3
from aiogram import Bot, Dispatcher, executor, types
from aiogram.types import InlineKeyboardMarkup, InlineKeyboardButton
|
In this step, we import the required libraries for building InlineKeyboardButton using the aiogram library.
- Bot: This class represents a Telegram bot and allows you to send messages, photos, videos, documents, and other types of content to Telegram users.
- Dispatcher: This class is the central part of the aiogram library and handles incoming messages and updates from Telegram users.
- executor: This module contains the functions that run the bot and handle incoming updates from Telegram.
- types: This module contains all the types used in the aiogram library, such as Message, User, Chat, and CallbackQuery.
- InlineKeyboardMarkup: This class is used to create inline keyboards in Telegram.Â
- InlineKeyboardButton: This class represents a single button in an inline keyboard.
Step 2: Put the token that you received from BotFather in the quotes
In this step, we create a Bot object and pass the bot token obtained from BotFather. we have already discussed how to get this bot token.
Step 3: Initializing the dispatcher object
In this step, we are initializing the Dispatcher object with the Bot object that we created in the previous step. The Dispatcher object is responsible for processing incoming updates and connecting them to the appropriate handlers.
Step 4: Defining and adding buttons
In this step, we are going to define two InlineKeyboardButton objects named button1 and button2 and add them to an InlineKeyboardMarkup object named keyboard_inline. The text attribute of each button sets the label displayed on the button, and the callback_data attribute is the data that the bot will receive when the button is pressed.
Python3
button1 = InlineKeyboardButton(text = "button1" , callback_data = "In_First_button" )
button2 = InlineKeyboardButton(text = "button2" , callback_data = "In_Second_button" )
keyboard_inline = InlineKeyboardMarkup().add(button1, button2)
|
Step 5: Message handler for the “/start” command
In this step, we are defining a message handler using the message_handler decorator. This handler is called whenever a user sends the “/start” command to the bot. The check function sends a message to the user with the text “hi! how are you”, and attaches the inline keyboard that we defined in the previous step using the reply_markup parameter.
Python3
@dp .message_handler(commands = [ 'start' ])
async def check(message: types.Message):
await message.reply( "hi! how are you" , reply_markup = keyboard_inline)
|
Step 6: Callback query handler for the inline keyboard buttons
In this step, we define a callback query handler using the callback_query_handler decorator. This handler is called whenever a user clicks one of the inline.
Python3
@dp .callback_query_handler(text = [ "In_First_button" , "In_Second_button" ])
async def check_button(call: types.CallbackQuery):
if call.data = = "In_First_button" :
await call.message.answer( "Hi! This is the first inline keyboard button." )
if call.data = = "In_Second_button" :
await call.message.answer( "Hi! This is the second inline keyboard button." )
await call.answer()
|
Step 7: Start the bot
In this step, we start the bot using the start_polling method of the executor module. This method starts an infinite loop that listens for incoming updates from Telegram and passes them to the Dispatcher object for processing. The bot will continue to run until we do not terminate the program manually.
Python3
executor.start_polling(dp)
|
Full implementation of InlineKeyboardButton
Here is the entire code for InlineKeyboardButtons in Telegram Bot:
Python3
from aiogram import Bot, Dispatcher, executor, types
from aiogram.types import InlineKeyboardMarkup, InlineKeyboardButton
bot = Bot(token = '')
dp = Dispatcher(bot)
button1 = InlineKeyboardButton(text = "button1" , callback_data = "In_First_button" )
button2 = InlineKeyboardButton(
text = "button2" , callback_data = "In_Second_button" )
keyboard_inline = InlineKeyboardMarkup().add(button1, button2)
@dp .message_handler(commands = [ 'start' ])
async def check(message: types.Message):
await message.reply( "hi! how are you" , reply_markup = keyboard_inline)
@dp .callback_query_handler(text = [ "In_First_button" , "In_Second_button" ])
async def check_button(call: types.CallbackQuery):
if call.data = = "In_First_button" :
await call.message.answer( "Hi! This is the first inline keyboard button." )
if call.data = = "In_Second_button" :
await call.message.answer( "Hi! This is the second inline keyboard button." )
await call.answer()
executor.start_polling(dp)
|
Output:
.jpg)
Output for Inline Keyboard Button
ReplyKeyboardButton in Telegram Bot using Python
Let us see how to create a Reply Keyboard Button in a Telegram Bot using the Aiogram framework.
Step 1:Importing the required libraries
- Almost all modules imported for the ReplyKeyboardButton implementation are identical to Inlinekeyboards.
- ReplyKeyboardButton is used for creating a custom keyboard with specific buttons to be sent as a reply to a user message.
Step 2: Setting up the Bot
- bot setup and dispatcher are identical for both buttons.
Step 3: Creating the reply keyboard
In this step, we create a new ReplyKeyboardMarkup object and add two buttons to it with the add method. The resize_keyboard and one_time_keyboard parameters are set to True, which means that the keyboard will be resized to fit the user’s device and will only be shown once after the user selects a button.
Python3
keyboard_reply = ReplyKeyboardMarkup(
resize_keyboard = True , one_time_keyboard = True ).add( "_button1" , "_button2" )
|
Step 4: Handling the “/start” and “/help” commands
In this step, we define a message handler function that is executed when the user sends a “/start” or “/help” command to the bot. The function sends a greeting message to the user with the reply keyboard that we created earlier.
Python3
@dp .message_handler(commands = [ 'start' , 'help' ])
async def welcome(message: types.Message):
await message.reply( "Hello! how are you?" , reply_markup = keyboard_reply)
|
Step 5: Handling all other messages
 In this step, we define a message handler function that is executed for all other messages that the bot receives. The function checks the text of the user’s message and responds accordingly. If the user selected the first or second button on the reply keyboard, the bot sends a message that corresponds to the button. If the user typed any other message, the bot sends a message that includes the text of the user’s message.
Python3
@dp .message_handler()
async def check_rp(message: types.Message):
if message.text = = '_button1' :
await message.reply( "Hi! this is first reply keyboards button." )
elif message.text = = '_button2' :
await message.reply( "Hi! this is second reply keyboards button." )
else :
await message.reply(f "Your message is: {message.text}" )
|
Step 6: Starting the bot
- This section is identical in both buttons, so you may refer to step 7 of InlineKeyboardButton.
Full implementation of ReplyKeyboardButton
Here is the entire code for ReplyKeyboardButtons in Telegram Bot:
Python3
from aiogram import Bot, Dispatcher, executor, types
from aiogram.types import ReplyKeyboardMarkup
bot = Bot(token = '')
dp = Dispatcher(bot)
keyboard_reply = ReplyKeyboardMarkup(
resize_keyboard = True , one_time_keyboard = True ).add( "_button1" , "_button2" )
@dp .message_handler(commands = [ 'start' , 'help' ])
async def welcome(message: types.Message):
await message.reply( "Hello! how are you?" , reply_markup = keyboard_reply)
@dp .message_handler()
async def check_rp(message: types.Message):
if message.text = = '_button1' :
await message.reply( "Hi! this is first reply keyboards button." )
elif message.text = = '_button2' :
await message.reply( "Hi! this is second reply keyboards button." )
else :
await message.reply(f "Your message is: {message.text}" )
executor.start_polling(dp)
|
Output: Â Â Â
.jpg)
The output of Reply Keyboard Button
Share your thoughts in the comments
Please Login to comment...