JUnit 5 – @AfterEach
Last Updated :
15 Jan, 2024
JUnit 5 is the recent version of the JUnit testing framework. JUnit 5 is the most powerful version compared to the other versions because it consists of enhanced features that make the developers’ lives easy. Moreover, writing and running the unit tests with the JUnit 5 testing framework increases the correctness of your code and ensures your Java application has no defects.
In JUnit 5, the @AfterEach annotation is just as same as the @After annotation which is in JUnit 4 testing framework. The only difference is that the naming convention between these both. If your JUnit testing framework is Junit4 then go for @After Annotation and JUnit 5 @AfterEach Annotation.
Prerequisites
- Knowledge of Java Programming Language.
- Basic understanding of Junit testing.
- Hands-on experience with Eclipse IDE.
Setting up Junit 5 Jar Files
First, we will write test cases in a simple Java project. To make sure that JUnit works finely in our program, download the Junit5 jar files from online and import them into the IDE. To import the jar files into the Java project,
Right-click on your Java Project > Select the option “Buildpath” > Select “Add libraries”. Then import the jar file from the local computer into the libraries.
@AfterEach Annotation
@AfterEach annotation is generally used to indicate that the annotated method must be executed after every @Test, @RepeatedTest, @ParameterizedTest, or @TestFactory in the same Test class. This means whenever you run the test method, the @AfterEach method will be executed at least once.
Example of @AfterEach annotation:
Now, let’s go through an example to understand more about @AfterEach annotation.
Project Structure:
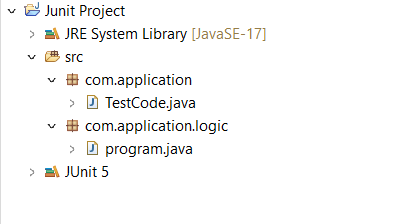
Main Class:
Java
package com.application.logic;
public class Program {
public int findMult( int a, int b)
{
return a*b;
}
}
|
In the above program, we have created a class with name “Program” with a method “findMult” inside. This method calculates product of two integers and returns the same. So, we will be testing the above code with the @AfterEach annotation and find out the functionality of it.
Test Class:
Java
package com.application;
import static org.junit.Assert.assertEquals;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.RepeatedTest;
import org.junit.jupiter.api.RepetitionInfo;
import org.junit.jupiter.api.TestInfo;
import com.application.logic.Program;
public class TestCode {
@RepeatedTest ( 3 )
public void testMul(TestInfo testInfo, RepetitionInfo repetitionInfo)
{
System.out.println( "Test Running: " +repetitionInfo.getCurrentRepetition());
Program obj= new Program();
assertEquals( 8 ,obj.findMult( 4 , 2 ));
System.out.println( "in multiplication" );
}
@AfterEach
public void cleanCheck()
{
System.out.println( "Running in CleanCheck " );
System.out.println( "---------------------------------------------------------" );
}
}
|
Output:
Test Running: 1
in multiplication
Running in CleanCheck
---------------------------------------------------------
Test Running: 2
in multiplication
Running in CleanCheck
---------------------------------------------------------
Test Running: 3
in multiplication
Running in CleanCheck
---------------------------------------------------------
Explaination of the above Method:
- In the above program we have created a class “TestCode” for testing our code. Inside this we have created a test method “testMul” which is our test case.
- @RepeatedTest annotation is used to iterate the test case method for 3 times so that we can know how the @AfterEach annotated method is working.
- (TestInfo testInfo, RepetitionInfo repetitionInfo) parameters are passed to this method to get the count of every time the test case is iterated.
- “testMul” method is the test case where it actually tests through assertEquals by passing two integers to the “findMul” method which is in the “Program” class and tests the output of two integers.
- cleanCheck() method is annotated with @AfterEach annotation. This method is executed each time whenever the “testMul” method is tested at least once.
Note: The @AfterEach annotated method should never be a static method. This will throw a runtime error
In the above output, we can see that whenever the “testMul” method is executed parallelly @AfterEach annotated method “cleanCheck” is also executed. which means after “in multiplication” is displayed “Running in CleanCheck ” is printed right after it.
Conclusion
@AfterEach annotation enhances the test isolation whenever each iteration is tested. This helps developers to maintain and run their tests without any difficulties. Test readability is increased with this annotation. The Junit 5 @AfterEach annotation serves the same functionality as @After annotation which is in JUnit 4 testing framework. The only difference is the naming convention and the version difference of the testing framework.
Share your thoughts in the comments
Please Login to comment...